When we are developing an application with Node.js, we could put all functionalities into one single huge file.
For the Node.js interpreter this isn’t a problem but, we could have problems in terms of code organization.
It is a best practice breaking down our huge files in more small files.
In node.js a functionality organized in single or multiple Javascript files, which can be reused throughout the main application, is called Module.
There are many modules in Node.js (we have used ‘readline-sync’ in a previous post) and we can create our custom Modules too.
In this post, we will create a simple application (index.js) that will use two custom modules (operation.js and operation2.js), containing some basic arithmetic operations.
[OPERATION.JS]
function add(val1,val2)
{
return val1 + val2;
}
function mult(val1,val2)
{
return val1 * val2;
}
// the command exports makes methods available outside the file.
module.exports = {
add,
mult
}
[OPERATIONS2.JS]
function sub(val1,val2)
{
return val1 - val2;
}
function div(val1,val2)
{
return val1/val2;
}
// the command exports makes methods available outside the file.
module.exports = {
sub,
div
}
[INDEX.JS]
// require the two files
var oper = require('./operation')
var oper2 = require('./operation2')
// run the methods of the first file
console.log("4+5 = " + oper.add(4,5))
console.log("5*4 = " + oper.mult(5,4))
// run the methods of the second file
console.log("50/10 = " + oper2.div(50,10))
console.log("50-35 = " + oper2.sub(50,35))
If we run the application, this will be the result:
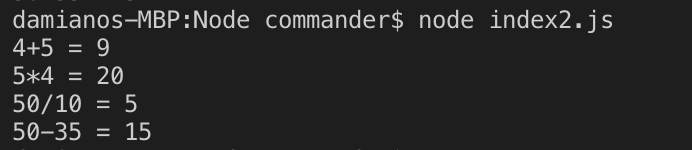