In this post, we will see how to manage For and While in Swift.
FOR
// [For]
let tot = 5
print("First loop")
for i in 1...tot{
print("Hello\(i)")
}
print("")
print("Second loop")
for i in 1..<tot{
print("Hello\(i)")
}
let tot2 = [10,20,30,40,50]
print("")
print("Third loop")
for i in tot2{
print(i)
}
print("")
print("Fourth loop")
for i in tot2{
if i==30
{
break
}
print(i)
}
print("")
print("Fifth loop")
for i in tot2{
if i==30
{
continue
}
print(i)
}
If we run this code in Playground, this will be the result:
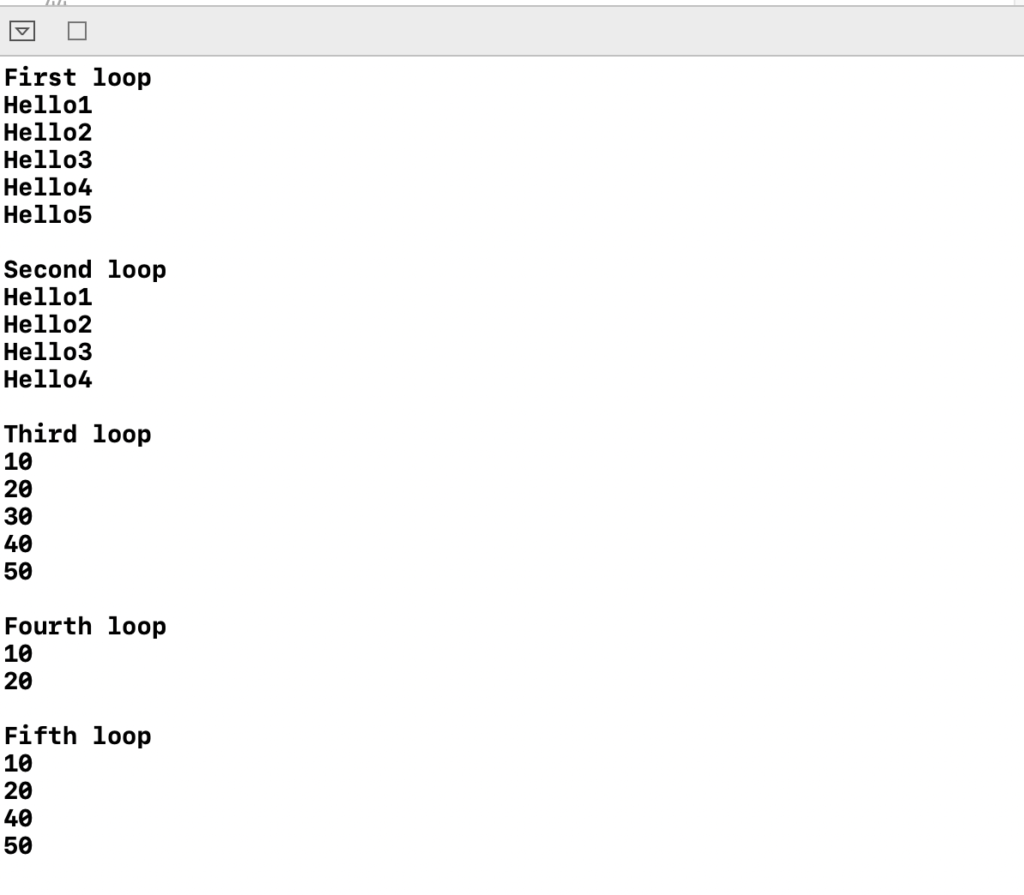
WHILE
// [While]
var count3 = 6
print("First While loop")
while count3>2{
print(count3)
count3 -= 1
}
count3 = 6
print("")
print("Second While loop")
while count3>2{
if(count3==5)
{
count3 -= 1
continue
}
else
{
print(count3)
count3 -= 1
}
}
count3 = 6
print("")
print("Third While loop")
while count3>2{
if(count3==5)
{
break
}
else
{
print(count3)
count3 -= 1
}
}
If we run this code in Playground, this will be the result:
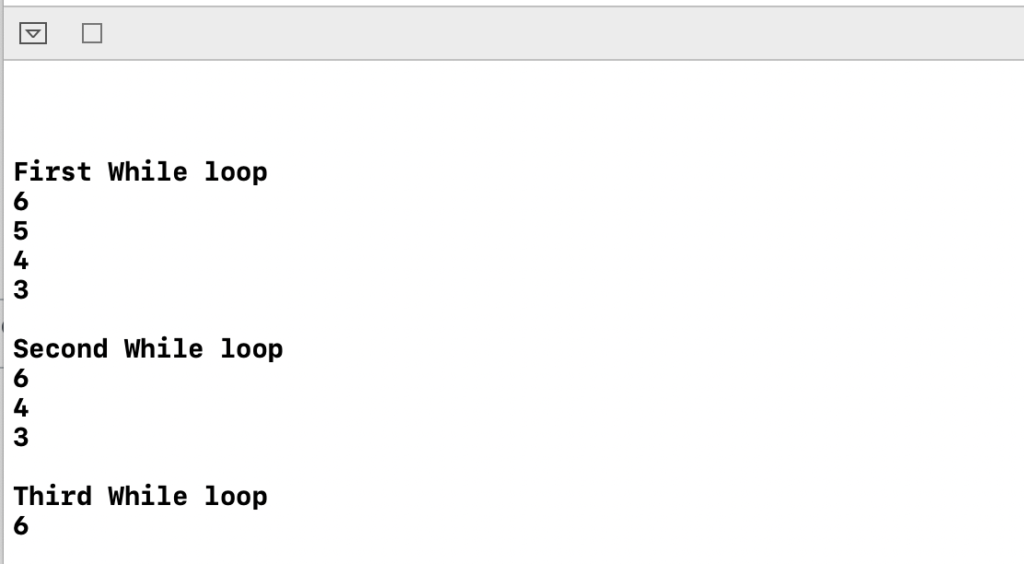