In this post, we will see how to manage Functions in Swift.
WITHOUT PARAMETERS IN INPUT & WITHOUT RETURN VALUE
import UIKit
// [Without parameters in input]
// [Without return value]
func printDate()
{
let varDate = Date()
let formatDate = DateFormatter()
formatDate.dateFormat = "dd/MM/yyyy"
print(formatDate.string(for: varDate)!)
}
print("First function")
printDate()
print("")
WITH PARAMETERS IN INPUT & WITHOUT RETURN VALUE
// [With parameters in input]
// [Without return value]
func sumInt(val1:Int, val2:Int)
{
let result = val1 + val2
print("The result is: \(result)")
}
print("Second function")
sumInt(val1: 10, val2: 20)
print("")
WITH PARAMETERS IN INPUT & WITH RETURN VALUE
// [With parameters in input]
// [With return value]
func sumInt2(val1:Int, val2:Int) -> Int
{
let result = val1 + val2
return result
}
print("Third function")
let variable1 = sumInt2(val1: 10, val2: 20)
print("The sum of 10 with 20 is: \(variable1)")
print("")
WITH PARAMETERS IN INPUT & WITH RETURN A TUPLE
// [With parameters in input]
// [With return a tuple]
func findMaxMin(lstInt:[Int]) -> (Min: Int, Max: Int)
{
var min:Int = 0
var max:Int = 0
min = lstInt.min() ?? 0
max = lstInt.max() ?? 0
return(min, max)
}
print("Fourth function")
let variable2 = findMaxMin(lstInt: [1,22,3,40,5,68,7])
print("The Min value is \(variable2.Min) and the Max value is: \(variable2.Max)")
print("")
WITH DEFAULT PARAMETERS IN INPUT & WITH RETURN A VALUE
// [With default parameter in input]
// [With return a value]
func sumInt3(val1:Int, val2:Int = 100) -> Int
{
let result = val1 + val2
return result
}
print("Fifth function")
let variable3 = sumInt3(val1: 10, val2: 20)
print("The sum of 10 with 20 is: \(variable1)")
let variable4 = sumInt3(val1: 10)
print("The sum is: \(variable4)")
If we run all code in Playground, this will be the result:
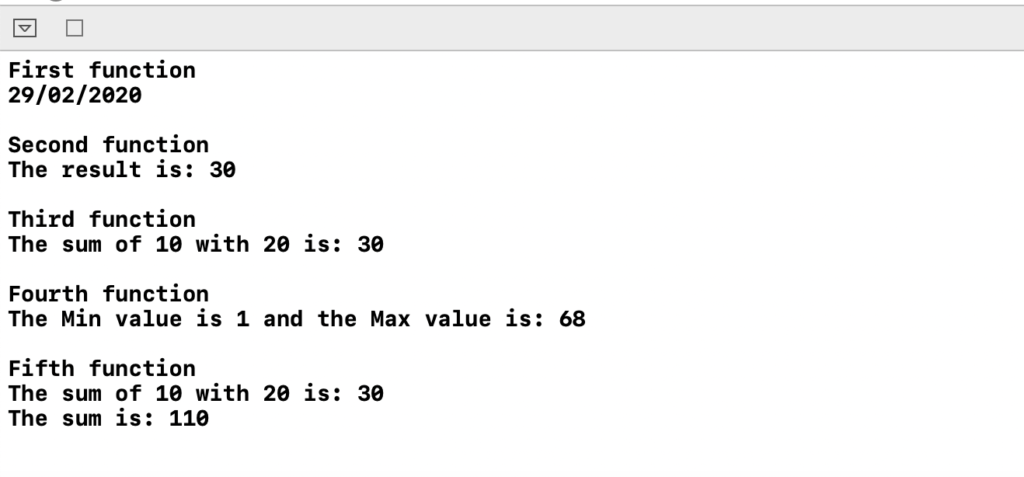