In this post, we will see how to define a Form, using Template-drive forms approach, for creating new users in our usual angular project.
First of all, we create another component called newusertd and then, we will add it in app-routing and topmenu:
ng g c newusertd

[APP-ROUTING.MODULES.TS]
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ListusersComponent } from './listusers/listusers.component';
import { HomepageComponent} from './homepage/homepage.component';
import { NewuserComponent} from './newuser/newuser.component';
import { NewusertdComponent } from './newusertd/newusertd.component';
const routes: Routes = [
{ path: '', pathMatch: 'full', redirectTo: 'homepage'},
{ path: 'homepage', component: HomepageComponent },
{ path: 'listusers', component: ListusersComponent },
{ path: 'newuser', component: NewuserComponent },
{ path: 'newuser2', component: NewusertdComponent },
{ path: '**', pathMatch: 'full', redirectTo: 'homepage' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
[TOPMENU.COMPONENT.HTML]
<nav class="navbar navbar-dark bg-dark mb-5">
<a class="navbar-brand" routerLink="homepage">[Manage Users]</a>
<div class="navbar-expand mr-auto">
<ul class="nav navbar-nav" routerLinkActive="active">
<li class="nav-item"><a class="nav-link" routerLink="/listusers">List Users</a></li>
<li class="nav-item"><a class="nav-link" routerLink="/newuser">Create User</a></li>
<li class="nav-item"><a class="nav-link" routerLink="/newuser2">Create User Template</a></li>
</ul>
</div>
</nav>
Now, in order to use the Form with Template Drive, we have to add the component FormsModule in app.module.ts:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
import { FormsModule } from '@angular/forms';
import { ReactiveFormsModule } from '@angular/forms';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { ListusersComponent } from './listusers/listusers.component';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { TopmenuComponent } from './topmenu/topmenu.component';
import { HomepageComponent } from './homepage/homepage.component';
import { NewuserComponent } from './newuser/newuser.component';
import { NewusertdComponent } from './newusertd/newusertd.component';
@NgModule({
declarations: [
AppComponent,
ListusersComponent,
TopmenuComponent,
HomepageComponent,
NewuserComponent,
NewusertdComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
BrowserAnimationsModule,
ReactiveFormsModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Finally, we have to modify three files:
[NEWUSERTD.COMPONENT.TS]
import { Component, OnInit } from '@angular/core';
import { User } from '../user';
import { WebapiuserService } from '../webapiuser.service';
import { Router } from '@angular/router';
@Component({
selector: 'app-newusertd',
templateUrl: './newusertd.component.html',
styleUrls: ['./newusertd.component.css']
})
export class NewusertdComponent implements OnInit {
// Like the React Form, I put in the code the list of
// typeUser.
// In a real application it could be better using a
// Web service in order to get the list
typeUser: Array<string> = [
'Admin',
'Reader',
'Writer'
];
// Definition of the object that will use into the Form
objUser: User = new User();
constructor(private serviceuser: WebapiuserService, private router: Router) { }
ngOnInit() {
}
onSubmit()
{
// call the method to create a new user
this.serviceuser.createUser(this.objUser)
.subscribe(
// if it's ok, call the method newUserOk
data => this.newUserOK(),
error => console.log("Error", error)
)
}
newUserOK()
{
// The system will show a message
alert("New user " + this.objUser.username + "was created!");
// and will go to the List Users page
this.router.navigate(['/listusers']);
}
}
[NEWUSERTD.COMPONENT.HTML]
<div class="jumbotron">
<div class="container">
<div class="row">
<div class="col-md-6 offset-md-3">
<h3>New User with Template Driven</h3>
<form (ngSubmit)="onSubmit()" #userForm="ngForm">
<div class="form-group">
<label for="username">Username</label>
<input type="text" class="form-control" id="username" required [(ngModel)]="objUser.username" name="username" #username="ngModel">
<div [hidden]="username.valid || username.pristine" class="alert alert-danger">
Username is required
</div>
</div>
<div class="form-group">
<label>Type User Name</label>
<select class="form-control" name="typeUserName" id="typeUserName" required [(ngModel)]="objUser.typeUserName" #typeUserName="ngModel">
<option *ngFor="let item of typeUser" [ngValue]="item">{{item}}</option>
</select>
<div [hidden]="typeUserName.valid || typeUserName.pristine" class="alert alert-danger">
typeUserName is required
</div>
</div>
<button type="submit" class="btn btn-success" [disabled]="!userForm.form.valid">Register</button>
</form>
</div>
</div>
</div>
</div>
[NEWUSERTD.COMPONENT.CSS]
.ng-valid[required], .ng-valid.required
{
border-left: 5px solid #42A948; /* green */
}
.ng-invalid:not(form)
{
border-left: 5px solid #a94442; /* red */
}
Now, in order to verify that the Form works fine, we run (with the command ng serve) the application and we try to add a new user:
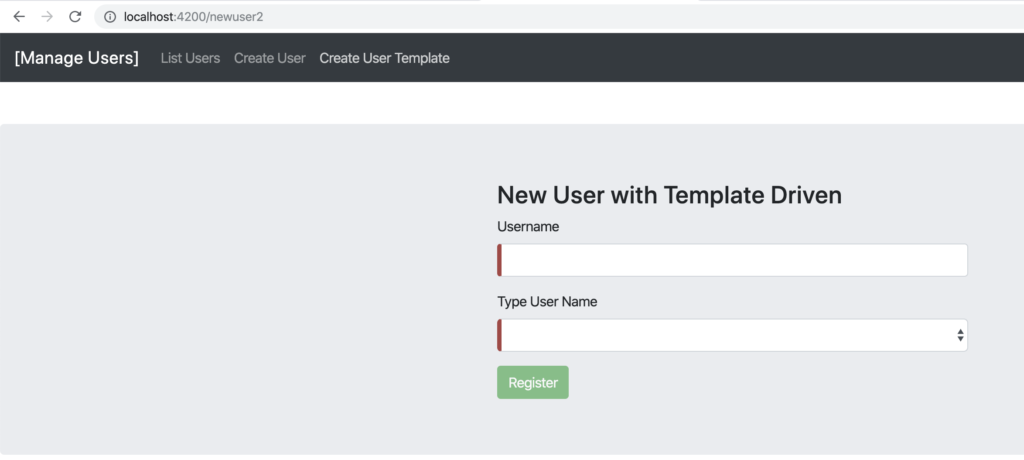
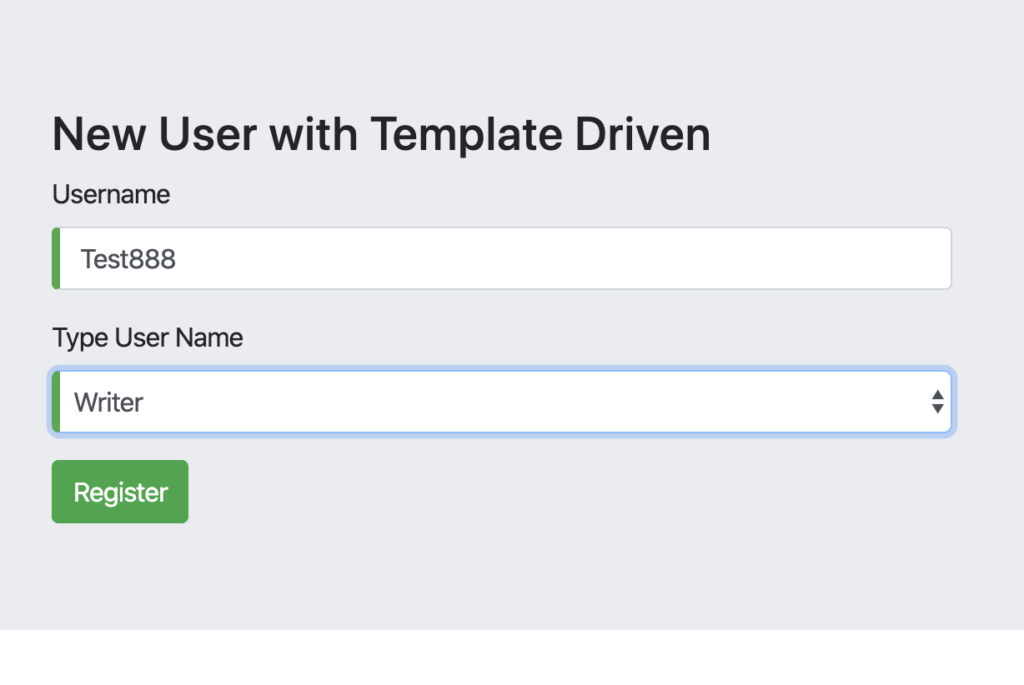
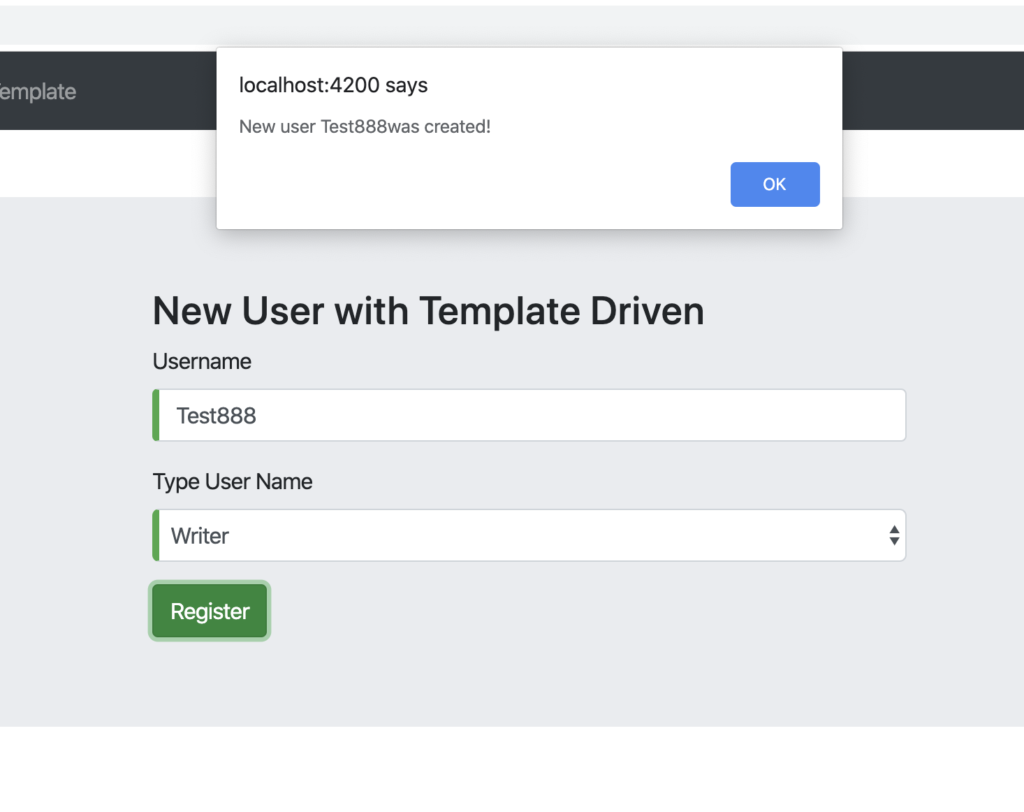
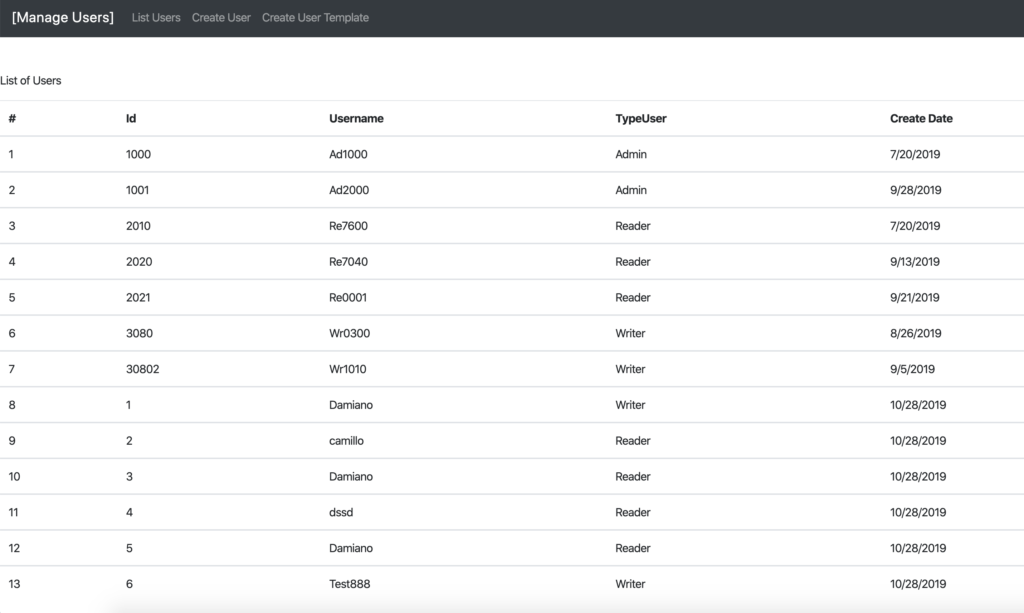