In this post, we will see how to create a static and a dynamic list in SwiftUI.
We will use the same project created in SwiftUI – Button and refresh UI and we will add a new SwiftUI View called “ContentViewList”:
[CONTENTVIEWLIST.SWIFT]
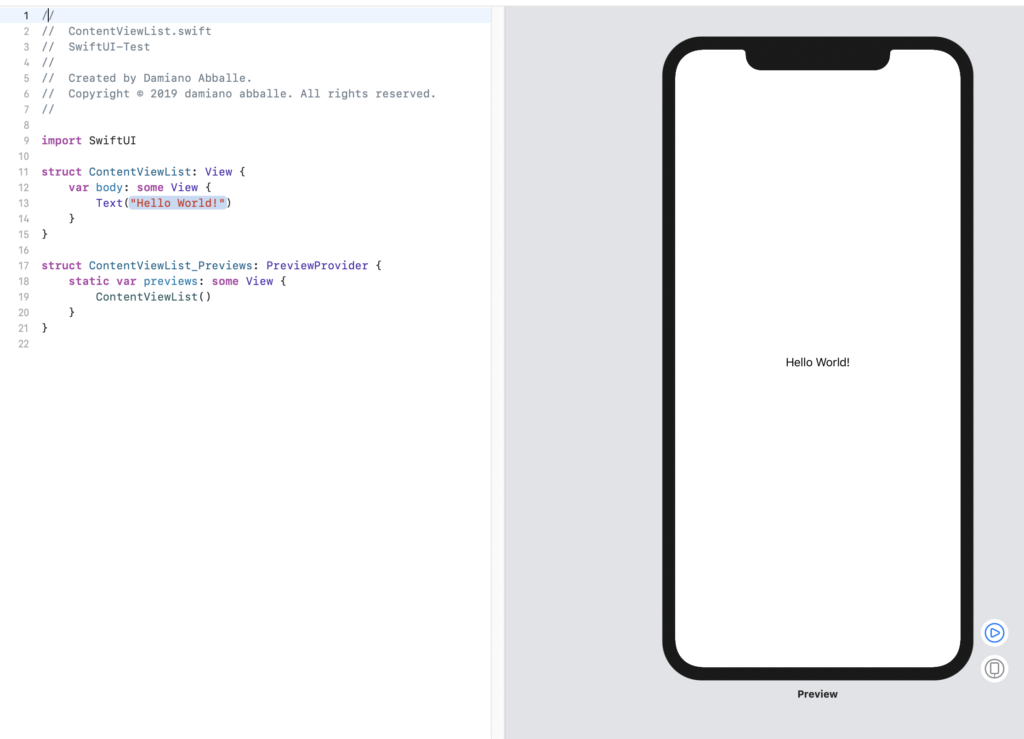
STATIC LIST
import SwiftUI
struct ContentViewList: View {
var body: some View {
// Defintion of a Navigation Controller
NavigationView{
// definition of a static list
List{
Text("Name1")
Text("Name2")
Text("Name3")
Text("Name4")
Text("Name5")
}.navigationBarTitle("Static List")
}
}
}
struct ContentViewList_Previews: PreviewProvider {
static var previews: some View {
ContentViewList()
}
}
In order to run the application with the new SwiftUI View, we open the file SceneDelegate.swift and we modify the function “scene”:
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) {
// We have to put here the new SwiftUI View
let contentView = ContentViewList()
// Use a UIHostingController as window root view controller.
if let windowScene = scene as? UIWindowScene {
let window = UIWindow(windowScene: windowScene)
window.rootViewController = UIHostingController(rootView: contentView)
self.window = window
window.makeKeyAndVisible()
}
}
Now, if we run the application, this will be the result:

DYNAMIC LIST
We create a swift file called StructUser.swift, where we will define a struct like that:
import Foundation
// When we use the identifiable protocol,
// we have to to implement a variable called id
struct User: Identifiable {
var id: Int
var Username:String
var Password:String
}
Then, we modify the file ContentViewList.swift, in order to define a Dynamic List:
import SwiftUI
struct ContentViewList: View {
// define an array of User called lstUser
let lstUser: [User] = [
.init(id: 1, Username: "Username1", Password: "Password1"),
.init(id: 2, Username: "Username2", Password: "Password2"),
.init(id: 3, Username: "Username3", Password: "Password3"),
.init(id: 4, Username: "Username4", Password: "Password4"),
.init(id: 5, Username: "Username5", Password: "Password5"),
.init(id: 6, Username: "Username6", Password: "Password6")
]
var body: some View {
// Defintion of a Navigation Controller
NavigationView{
// we put the array into a List
List(lstUser) { item in
HStack {
Text("ID: \(item.id)")
VStack {
Text(item.Username)
Text(item.Password)
}.padding(.leading, 20)
}.padding(.leading, 10)
}.navigationBarTitle("Dynamic List")
}
}
}
struct ContentViewList_Previews: PreviewProvider {
static var previews: some View {
ContentViewList()
}
}
Now, if we run the application, this will be the result:
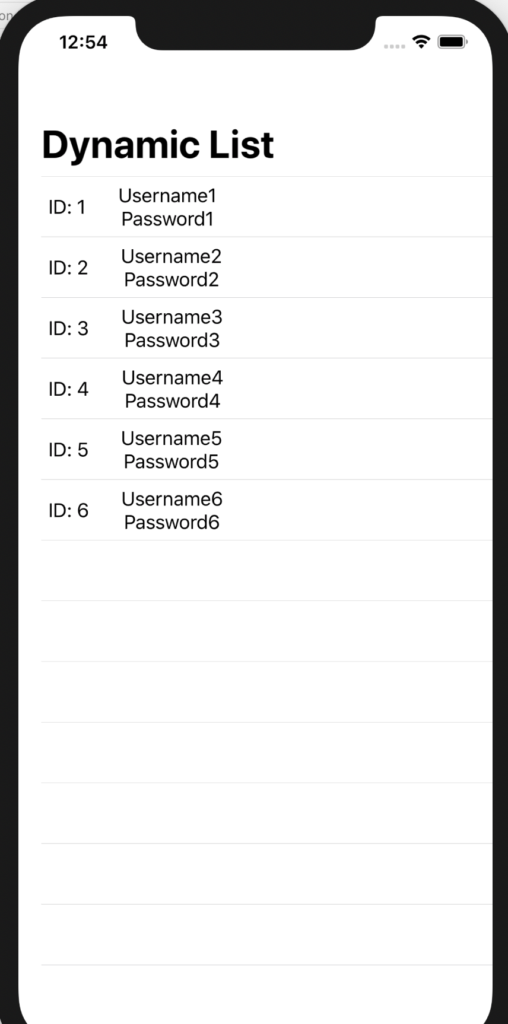