From Microsoft Web Site:
“Azure Storage is Microsoft’s cloud storage solution for modern data storage scenarios. Azure Storage offers a massively scalable object store for data objects, a file system service for the cloud, a messaging store for reliable messaging, and a NoSQL store.
Azure Storage includes these data services:
Azure Blobs: A massively scalable object store for text and binary data.
Azure Files: Managed file shares for cloud or on-premises deployments.
Azure Queues: A messaging store for reliable messaging between application components.
Azure Tables: A NoSQL store for schemaless storage of structured data.”
Azure Blob storage is Microsoft’s object storage solution for the cloud. Blob storage is optimized for storing massive amounts of unstructured data. Unstructured data is data that does not adhere to a particular data model or definition, such as text or binary data.
Azure Storage supports three types of blobs:
Block blobs: store text and binary data, up to about 4.7 TB. Block blobs are made up of blocks of data that can be managed individually.
Append blobs: are made up of blocks like block blobs, but are optimized for append operations. Append blobs are ideal for scenarios such as logging data from virtual machines.
Page blobs: store random access files up to 8 TB in size. Page blobs store virtual hard drive (VHD) files and serve as disks for Azure virtual machines.“
In this post, we will see how to save a Log file into a Blob Storage, using Append blobs.
First of all, we open a browser, go to Azure portal and we create a Storage Account:
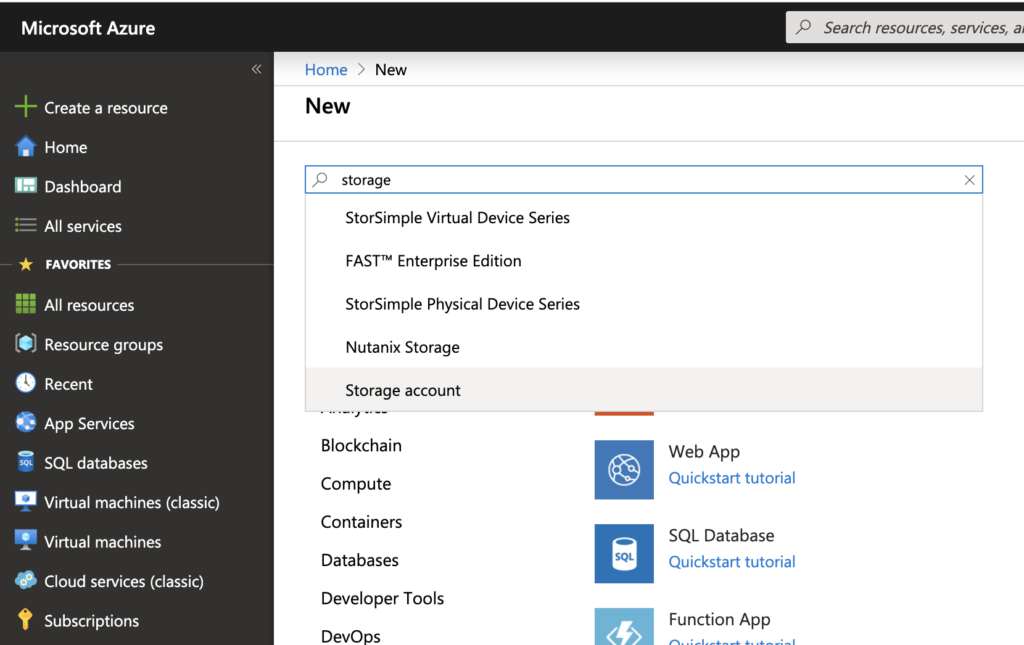
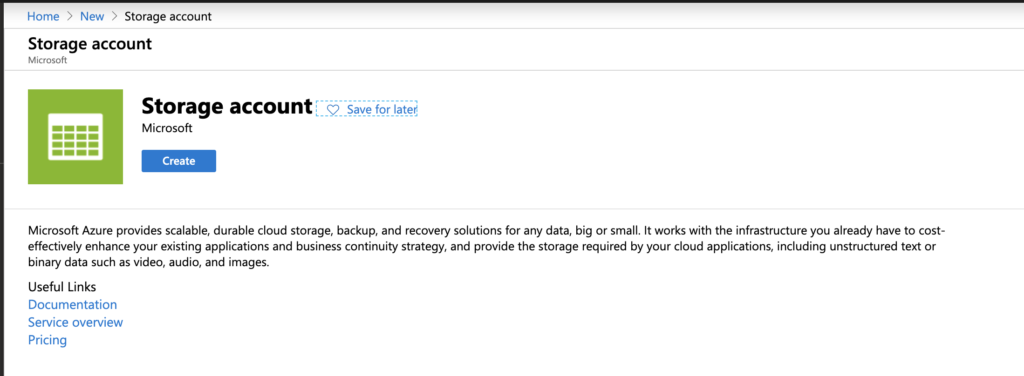
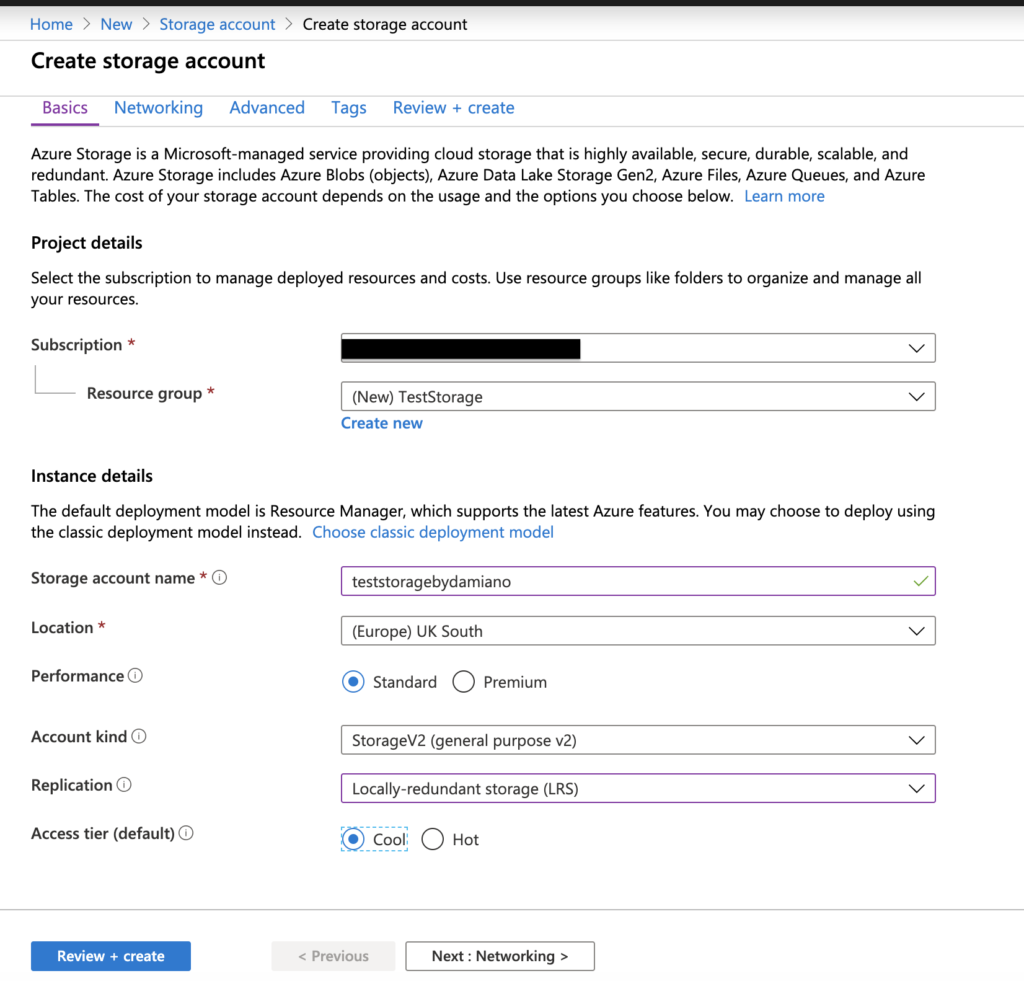
After the validation, we click on “Create” in order to create the Storage Account:
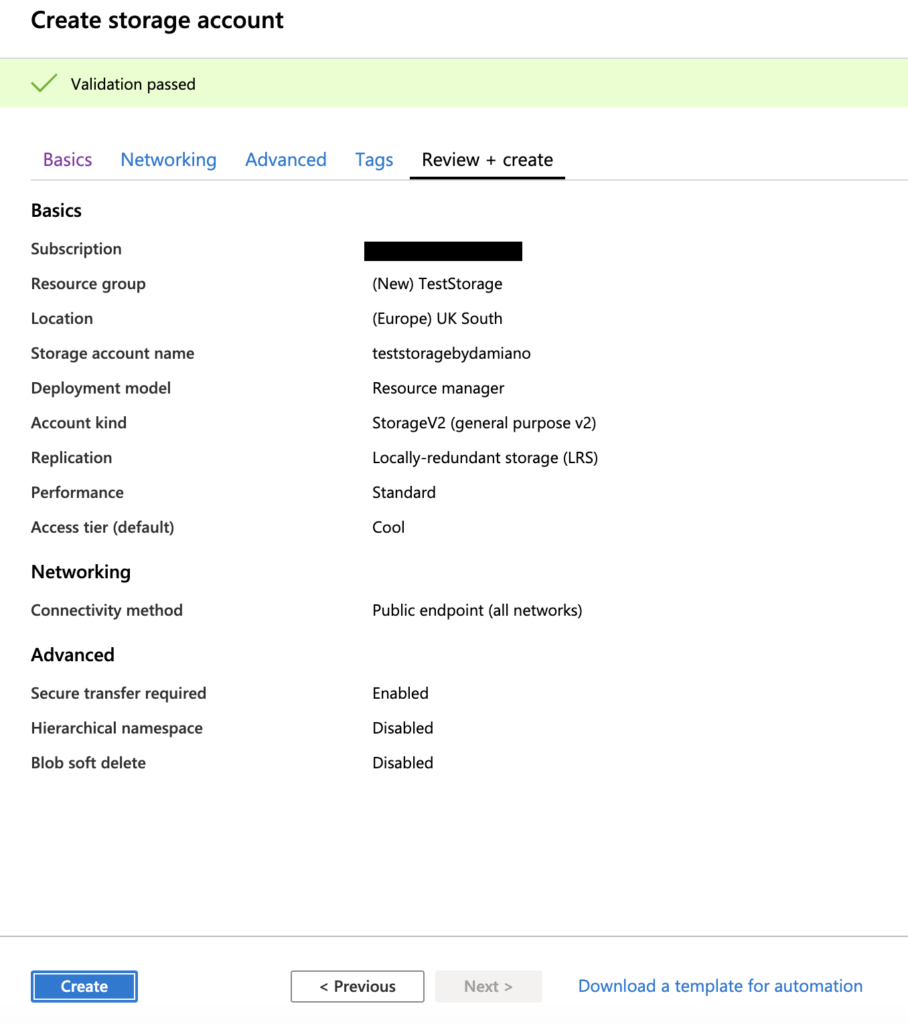
The Storage Account has been created:
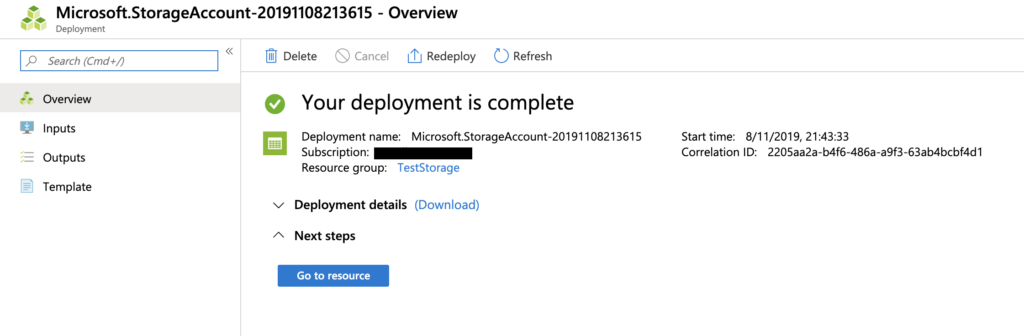
Now, we go to resource and then click on Containers:
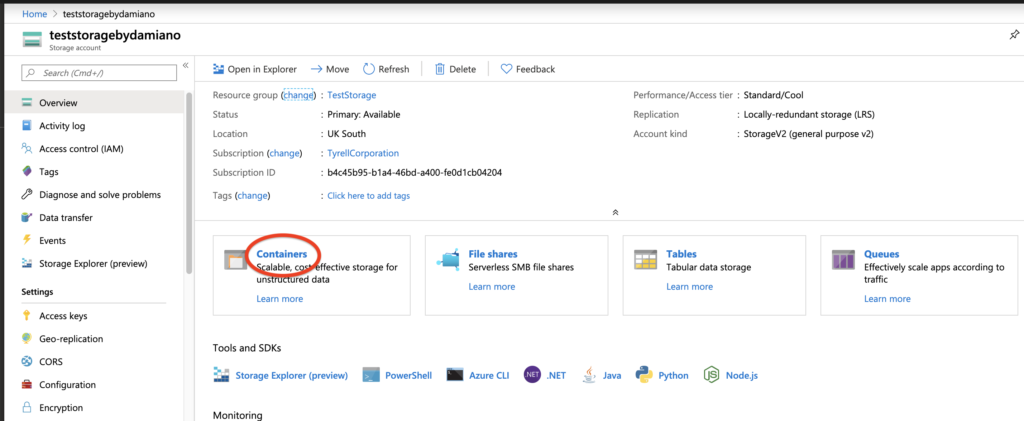
and we create a Container called “log”:
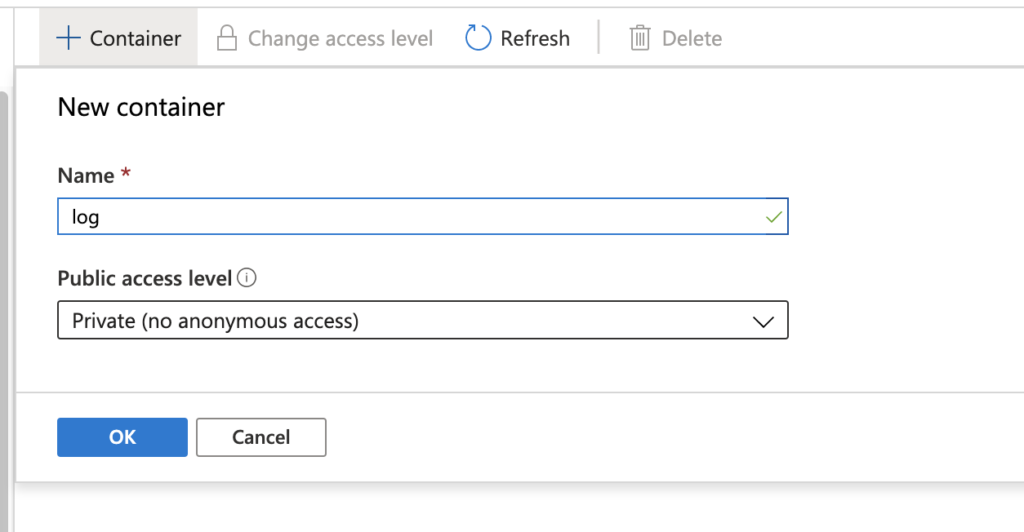
In order to get the Storage’s connection, we have to push on “Access Key” and take the value into “Connection string”:
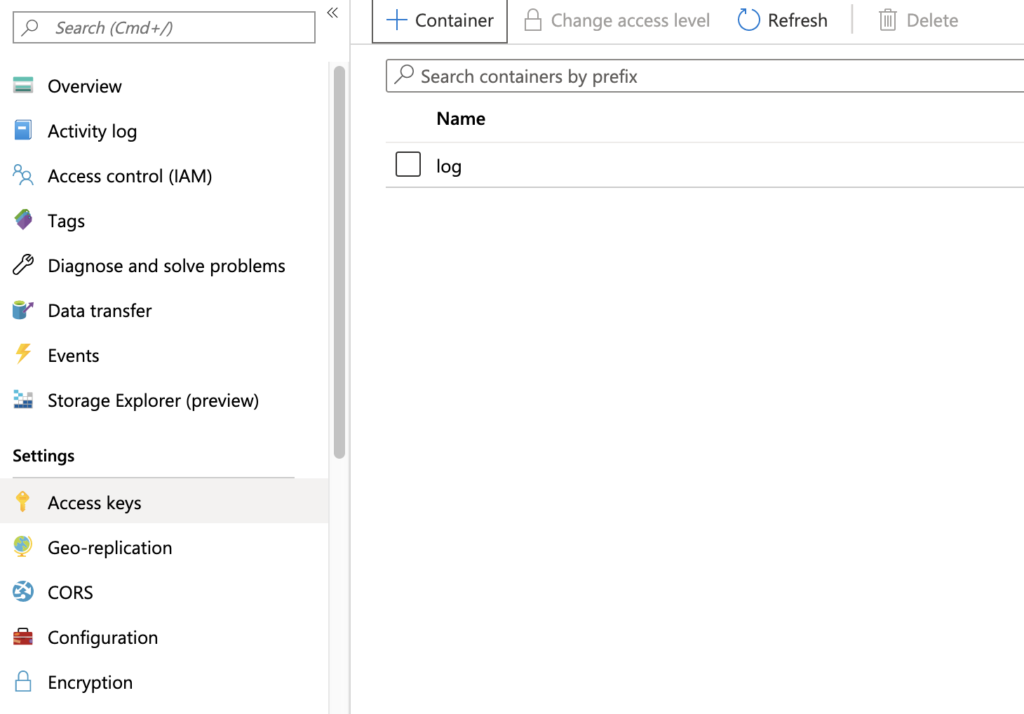
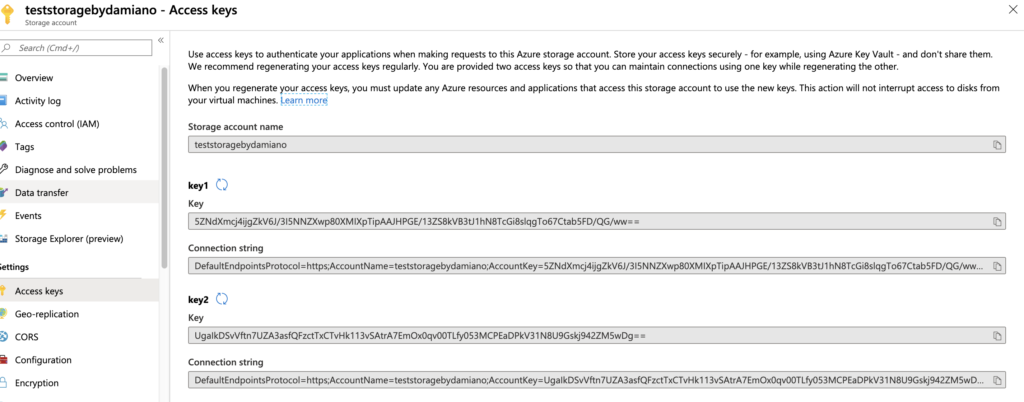
Now, we open Visual Studio 2019, create a Console Application and we write this code in the file Program.cs:
using Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Blob;
using System;
using System.Threading.Tasks;
namespace LogFile
{
class Program
{
static async Task Main(string[] args)
{
// define the connection string with the Storage
string connectString = "DefaultEndpointsProtocol=https;AccountName=teststoragebydamiano;AccountKey=5ZNdXmcj4ijgZkV6J/3I5NNZXwp80XMIXpTipAAJHPGE/13ZS8kVB3tJ1hN8TcGi8slqgTo67Ctab5FD/QG/ww==;EndpointSuffix=core.windows.net";
// define container's name
string containerName = "log";
// define log file's name
string logFileName = "TestLog.log";
// define the Cloud Storage Account
var storageAccount = CloudStorageAccount.Parse(connectString);
// define the Cloud Blob Client used to manage the containers
var blobClient = storageAccount.CreateCloudBlobClient();
// define the new value to add into LogFile
string newValueAdd = $"Guid:{Guid.NewGuid().ToString()};ValueInt:{new Random().Next(1,1000)};Date:{DateTime.Now.ToLongTimeString()}";
// write log file
await WriteLog(containerName, logFileName, blobClient, newValueAdd);
}
static async Task WriteLog(string nameContainer, string nameLogFile, CloudBlobClient objBlobClient, string newValue)
{
// take Container's reference
var container = objBlobClient.GetContainerReference(nameContainer.ToString());
// take Blob's reference to modify
var blob = container.GetAppendBlobReference(nameLogFile);
// verify the existance of blob
bool isPresent = await blob.ExistsAsync();
// if blob doesn't exist, the system will create it
if (!isPresent)
{
await blob.CreateOrReplaceAsync();
}
// append the new value and new line
await blob.AppendTextAsync($"{newValue} \n");
}
}
}
Finally, in order to verify that everything worked fine, we run twice the application and then, from Azure portal, we download the Log File created by the application:
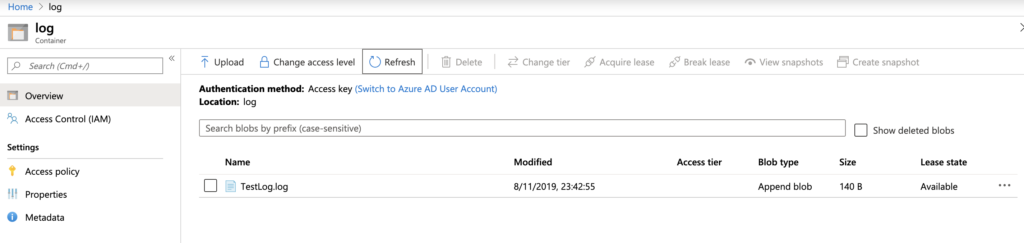
and the we open it:
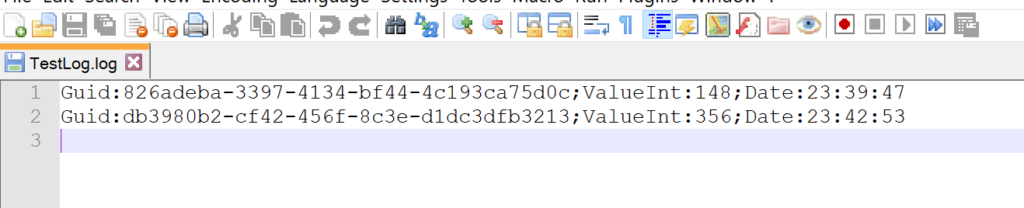
The application works fine, because there are two items inside the file.