In this post, we will see how to pass values between two components, using the router state attribute (from Angular 7.0.2), creating a method to modify the username property in our usual project.
First of all, we add a link button in the listusers component for using a method called goedit to update the username property:
[LISTUSERS.COMPONENT.HTML]
<table class="table">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Id</th>
<th scope="col">Username</th>
<th scope="col">TypeUser</th>
<th scope="col">Create Date</th>
<th scope="col">Functions</th>
</tr>
</thead>
<tbody *ngFor="let item of lstUsers; index as i">
<tr>
<td>{{i+1}}</td>
<td>{{item.id}}</td>
<td>{{item.username}}</td>
<td>{{item.typeUserName}}</td>
<td>{{item.createDate}}</td>
<td>
<button type="button" class="btn btn-success" (click)="goedit(item.id)">Edit</button>
<button type="button" class="btn btn-primary" (click)="openModal(template, item.username, item.id)">Delete</button>
</td>
</tr>
</tbody>
</table>
[LISTUSERS.COMPONENT.TS]
goedit(id)
{
// go to the edit Component and pass the User ID selected in a variable called idUser
this.router.navigateByUrl('edit', { state: { idUser: id } });
}
}
Now, in the service “webapiuser”, we add the methods for getting and for updating User:
updateUser(inputUser: User)
{
return this.http.put<User>(this.webapiUserUrl + '/user', inputUser);
}
getUser(userId: number)
{
return this.http.get<User>(this.webapiUserUrl + "/user/" + userId);
}
Then, we create a component called edituser (ng g c edituser) where, we will write the code for updating the username:
[EDITUSER.COMPONENT.HTML]
<div class="jumbotron">
<div class="container">
<div class="row">
<div class="col-md-6 offset-md-3">
<h3>Modify User Name</h3>
<form [formGroup]="userForm" (ngSubmit)="onSubmit()">
<div class="form-group">
<label>User Name</label>
<input type="text" formControlName="username" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.username.errors }" />
<div *ngIf="submitted && f.username.errors" class="invalid-feedback">
<div *ngIf="f.username.errors.required">User Name is required</div>
</div>
</div>
<div class="form-group">
<label>Type User Name</label>
<input type="text" formControlName="typeUserName" class="form-control" />
</div>
<div class="form-group">
<button class="btn btn-primary">Save</button>
</div>
</form>
</div>
</div>
</div>
</div>
[EDITUSER.COMPONENT.TS]
import { Component, OnInit } from '@angular/core';
import { User } from '../user';
import { Router } from '@angular/router';
import { WebapiuserService } from '../webapiuser.service';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-edituser',
templateUrl: './edituser.component.html',
styleUrls: ['./edituser.component.css']
})
export class EdituserComponent implements OnInit {
constructor(private formBuilder: FormBuilder, private serviceuser: WebapiuserService, public router: Router) {
this.userForm = this.formBuilder.group({
'username': ['', Validators.required],
'typeUserName': ['', Validators.required]
});
}
objUser: User;
idUserGet: number;
userForm: FormGroup;
submitted = false;
// easy access to form fields
get f() { return this.userForm.controls; }
ngOnInit() {
// We read the 'User ID' from browser’s history object
this.idUserGet = history.state.idUser;
// call the service to take the user
this.serviceuser.getUser(this.idUserGet).subscribe(data => {
this.objUser = data;
// put the values in the form
this.userForm.get('username').setValue(this.objUser.username);
this.userForm.get('typeUserName').setValue(this.objUser.typeUserName);
// disable the typeUserName
this.userForm.get('typeUserName').disable();
});
}
onSubmit()
{
this.submitted = true;
// stop here if form is invalid
if (this.userForm.invalid) {
return;
}
this.objUser.username = this.userForm.controls.username.value;
// call the method to update a user
this.serviceuser.updateUser(this.objUser)
.subscribe(
// if it's ok, call the method updateOK
data => this.updateOK(),
error => console.log("Error", error)
)
}
updateOK()
{
// go to User List
this.router.navigate(['/listusers']);
}
}
Finally, in order to use the new edituser component, we have to modify the routing file
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ListusersComponent } from './listusers/listusers.component';
import { HomepageComponent} from './homepage/homepage.component';
import { NewuserComponent} from './newuser/newuser.component';
import { NewusertdComponent } from './newusertd/newusertd.component';
import { EdituserComponent } from './edituser/edituser.component';
const routes: Routes = [
{ path: '', pathMatch: 'full', redirectTo: 'homepage'},
{ path: 'homepage', component: HomepageComponent },
{ path: 'listusers', component: ListusersComponent },
{ path: 'newuser', component: NewuserComponent },
{ path: 'newuser2', component: NewusertdComponent },
{ path: 'edit', component: EdituserComponent },
{ path: '**', pathMatch: 'full', redirectTo: 'homepage' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
In order to verify everything works fine, we run the application (ng serve), we open a browser and go to: http://localhost:4200/
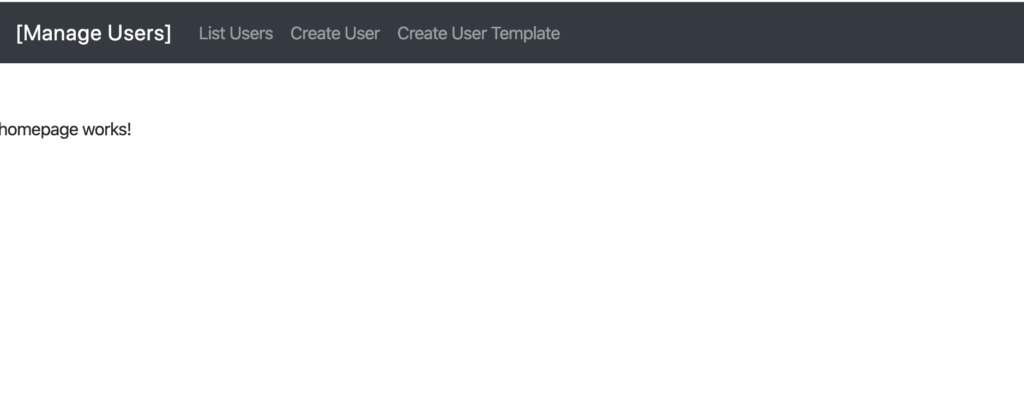
USER LIST
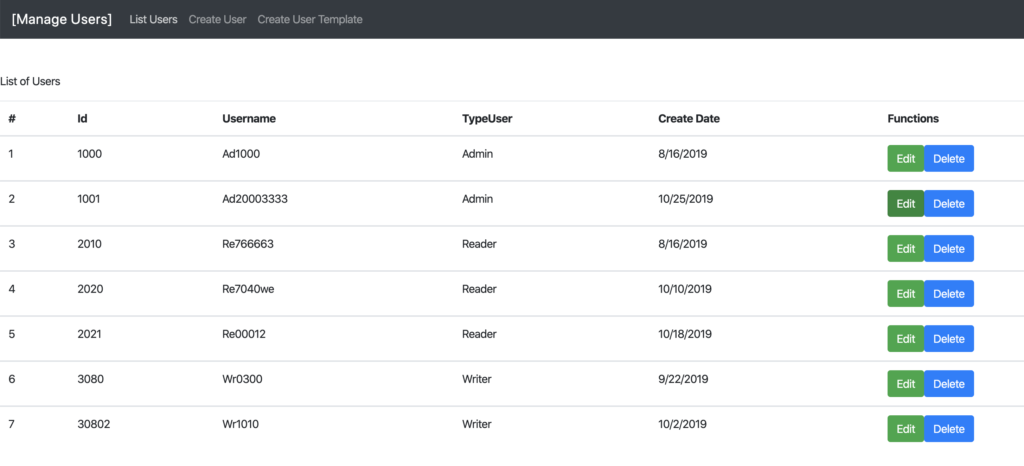
EDIT USER
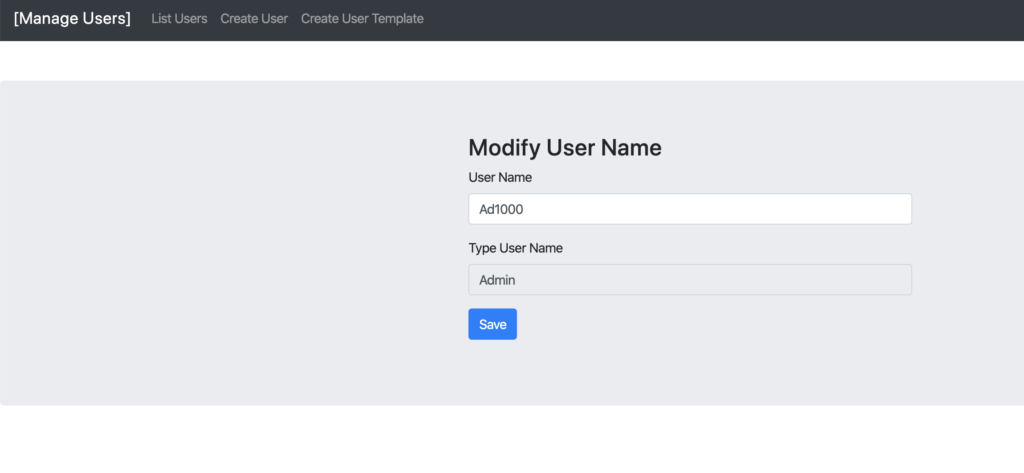
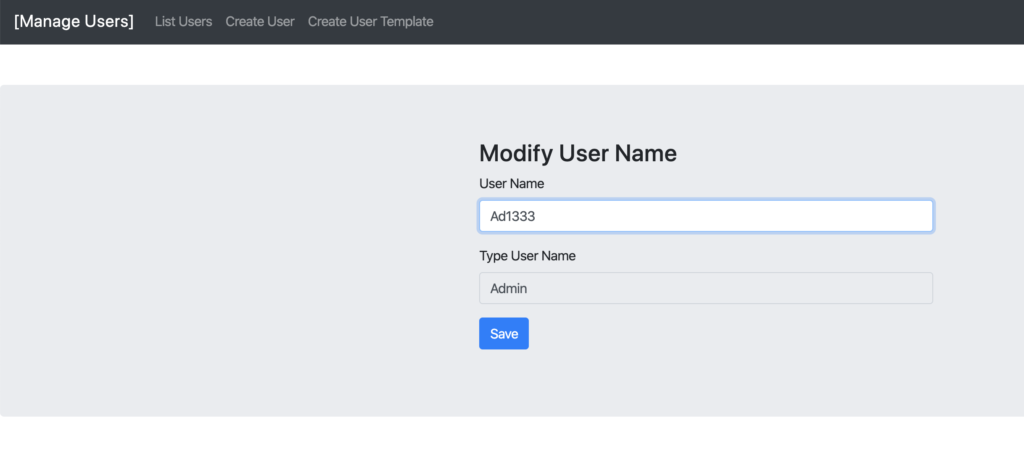
USER LIST
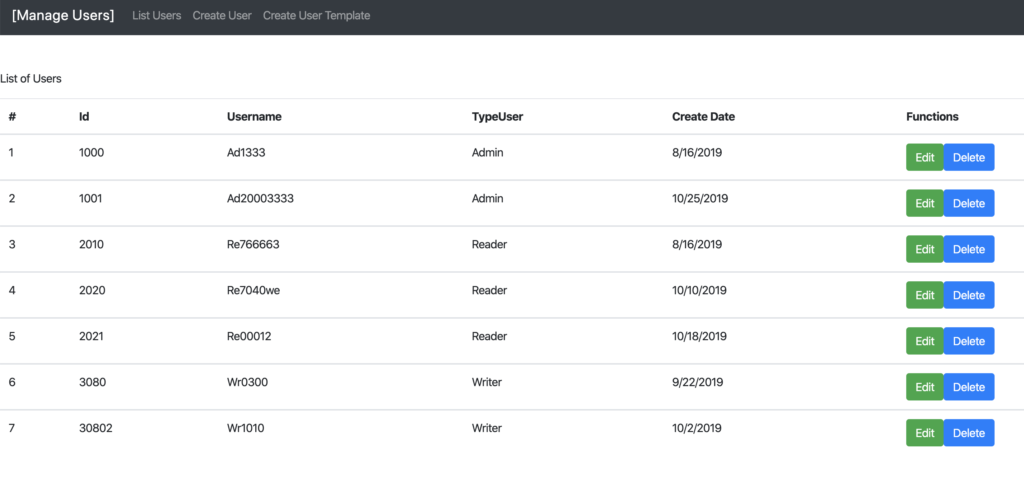