In this post, we will see how to pass values to a Component in React.
This is possible using the Props, that is kind of global variable or object, using them like HTML attributes.
First of all, we create a file called User.js:
[USER.JS]
import React from 'react'
const User = () => {
return(
<h1>Welcome User</h1>
)
}
export default User;
Then, we import and use this component in the file App.js:
[APP.JS]
import React from 'react';
import './App.css';
import User from './components/user'
function App() {
return (
<div className="App">
<header className="App-header">
<User></User>
<br></br>
<User></User>
</header>
</div>
);
}
export default App;
If we run the application, using the command npm start, this will be the result:

Now, we will use Props in order to display an Username.
We start to change User.js:
[USER.JS]
import React from 'react'
const User = (props) => {
return(
<h1>Welcome User {props.Username}</h1>
)
}
export default User;
Then, we modify App.js in order to pass the value in the component:
[APP.JS]
import React from 'react';
import './App.css';
import User from './components/user'
function App() {
return (
<div className="App">
<header className="App-header">
<User Username="Damiano"></User>
<br></br>
<User Username="Dorian"></User>
</header>
</div>
);
}
export default App;
Now, if we run the application, this will be the result:
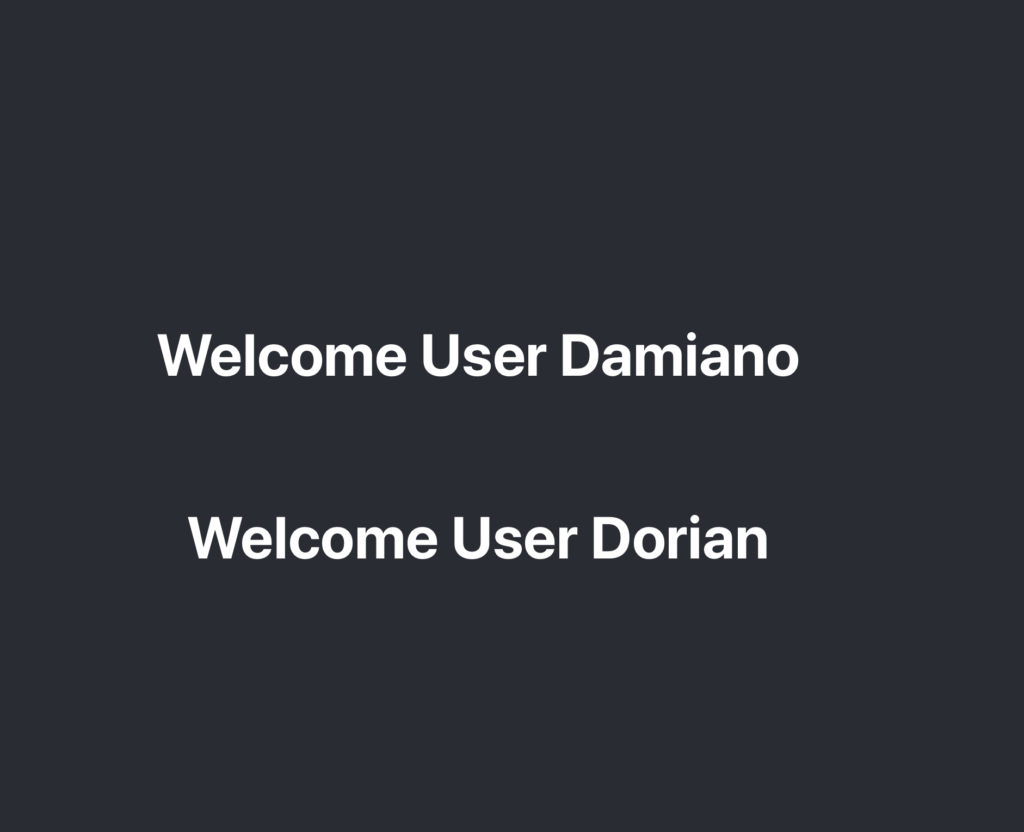
It is important to understand that Props is read-only and so, we cannot change the value.
We try to change the value of Props in User.js:
[USER.JS]
import React from 'react'
const User = (props) => {
props.Username = 'john';
return(
<h1>Welcome User {props.Username}</h1>
)
}
export default User;
If we run the application, we will have an error like that:

Obviously, we can take the value of Props an create another variable:
[USER.JS]
import React from 'react'
const User = (props) => {
var newUser = props.Username + ' John';
return(
<h1>Welcome User {newUser}</h1>
)
}
export default User;
If we run the application, this will be the result:
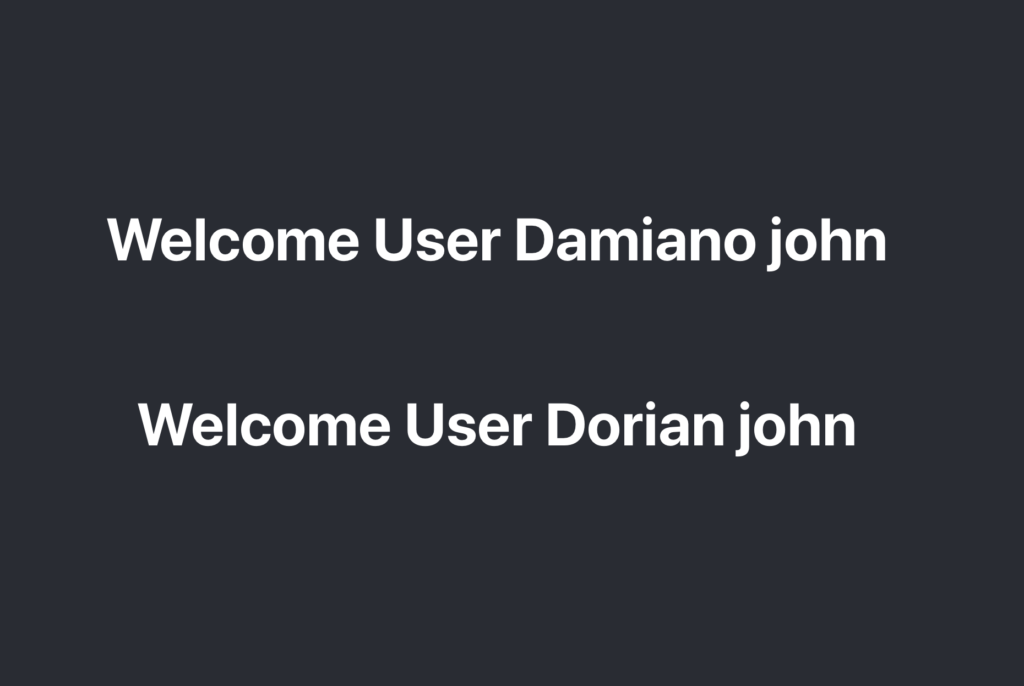
Another case where we need to use Props, is when we want to render what there is between the opening and closing tags of a component.
We create a component called TestChildren:
[TESTCHILDREN.JS]
import React from 'react'
const TestChildren = () =>{
return(
<div>
<h1>Hello World</h1>
<h2>Hello World</h2>
</div>
)
}
export default TestChildren;
Finally, we add the component in the App.js:
[APP.JS]
import React from 'react';
import './App.css';
import TestChildren from './components/TestChildren'
function App() {
return (
<div className="App">
<header className="App-header">
<TestChildren>
<b>Can you see it?</b>
</TestChildren>
</header>
</div>
);
}
export default App;
If we run the application, this will be the result:
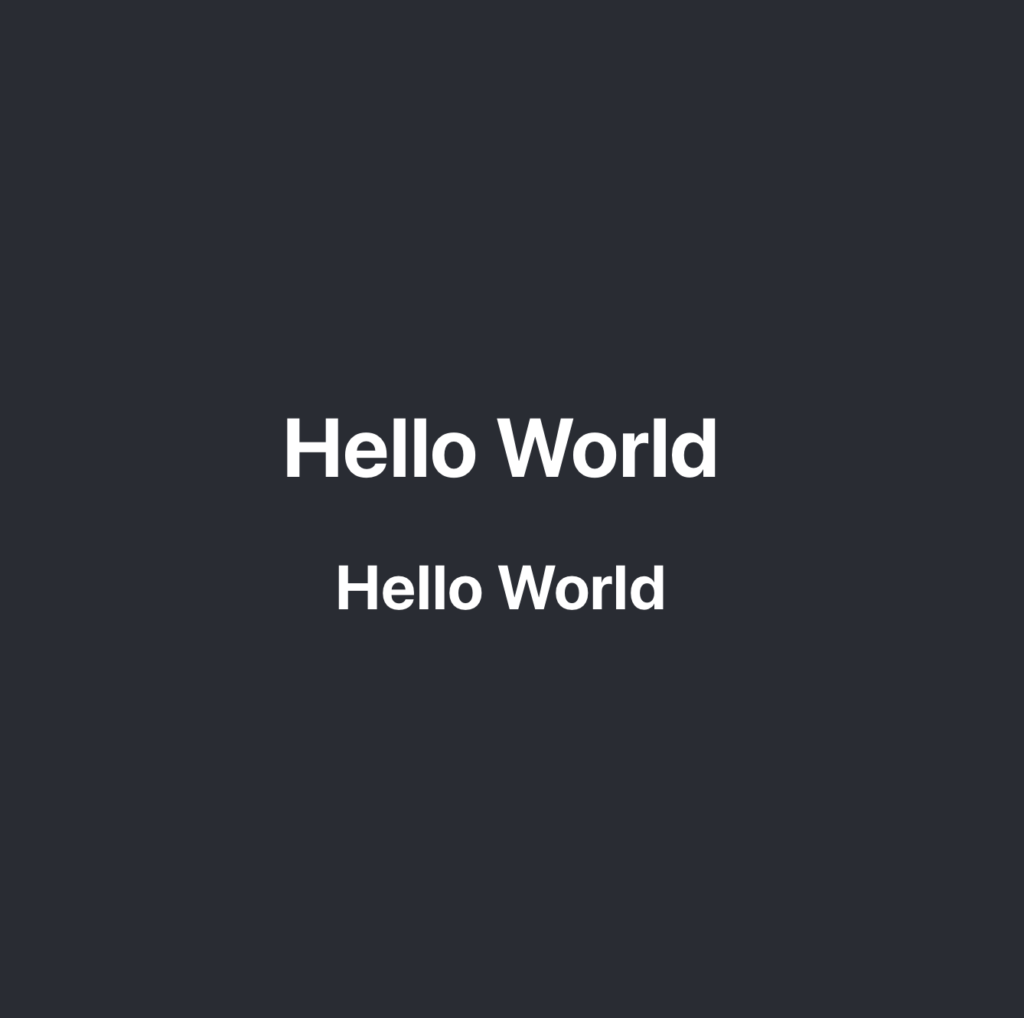
There is a problem: we cannot see the text “Can you see it”.
In order to fix this problem, we have to modify the component TestChildren.js, using Props:
[TESTCHILDREN.JS]
import React from 'react'
const TestChildren = (props) =>{
return(
<div>
<h1>Hello World</h1>
<h2>Hello World</h2>
{props.children}
</div>
)
}
export default TestChildren;
Now, if we run the application, this will be the result:
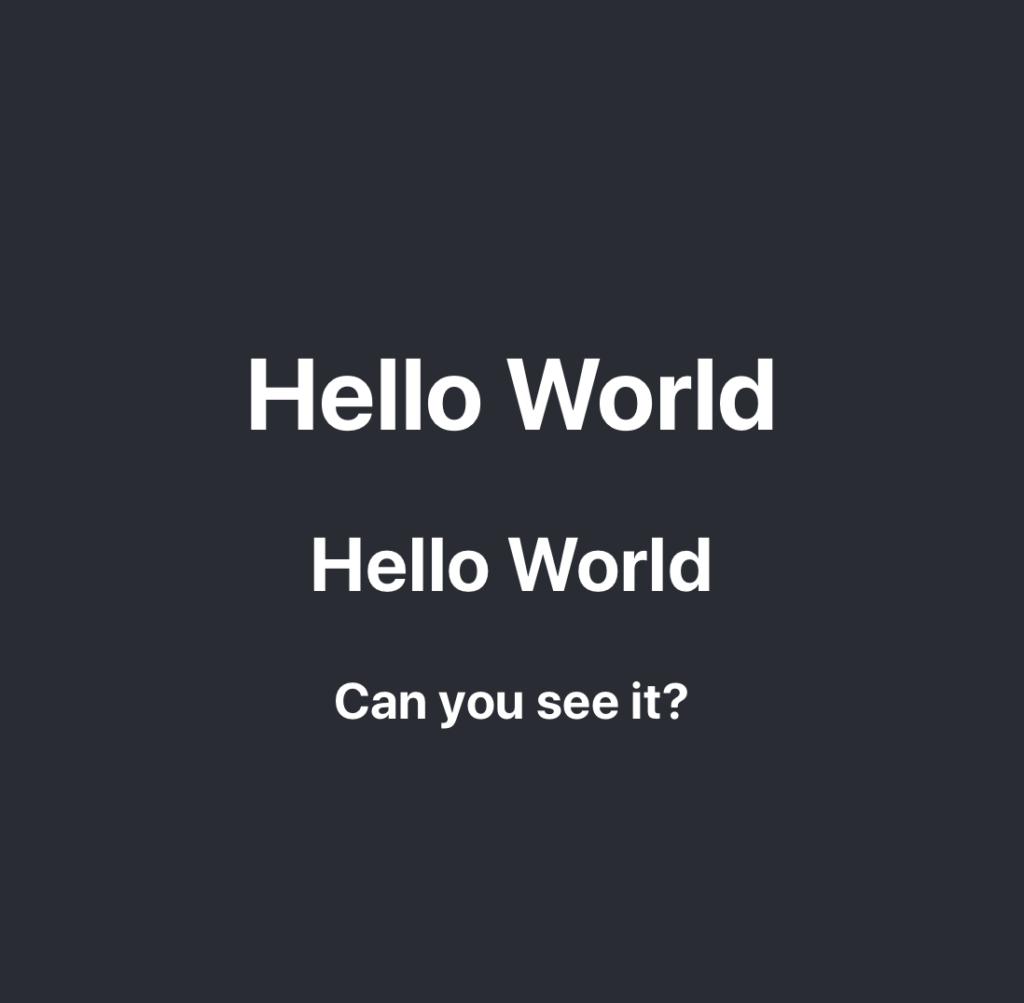