In this post, we will see how to add a Picker for selecting Sex in the form created in: How to create a Form
First of all, we define an array with all the items to display in the picker and then we define a variable where we will save the Picker’s item chosen:
// definition of Picker's items
let sexTypes = ["Select","Female", "Male"]
// definition of the variable where we will save the value selected
@State private var sexType = 0
Then, we add the Picker into the User Info section:
// Definition of Picker
Picker("Sex?", selection: $sexType)
{
// Picker's items
ForEach(0..<self.sexTypes.count)
{
Text(self.sexTypes[$0])
}
}
Finally, we add another condition in the disabledForm variable:
// The form will disabled until all filed are filled
var disabledForm: Bool{
firstName.isEmpty || lastName.isEmpty || email.isEmpty || password.isEmpty || sexType==0
}
If we run the application, this will be the result:
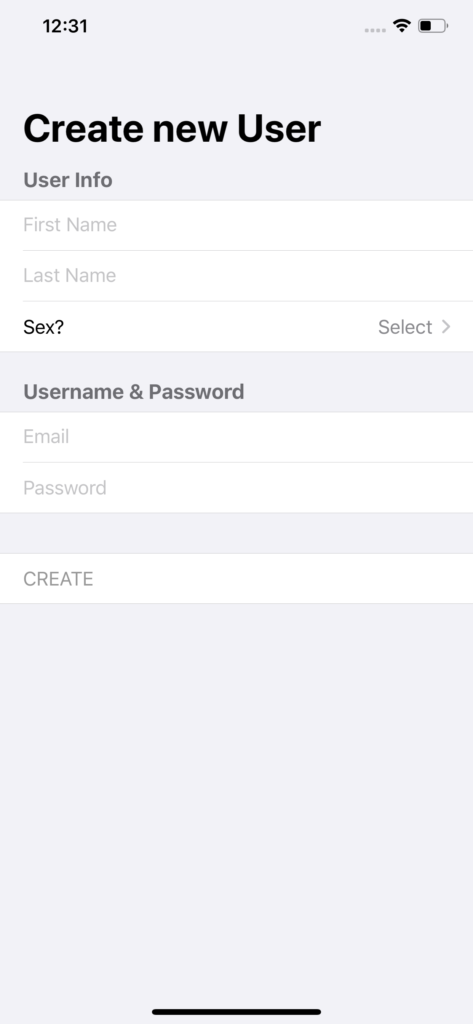
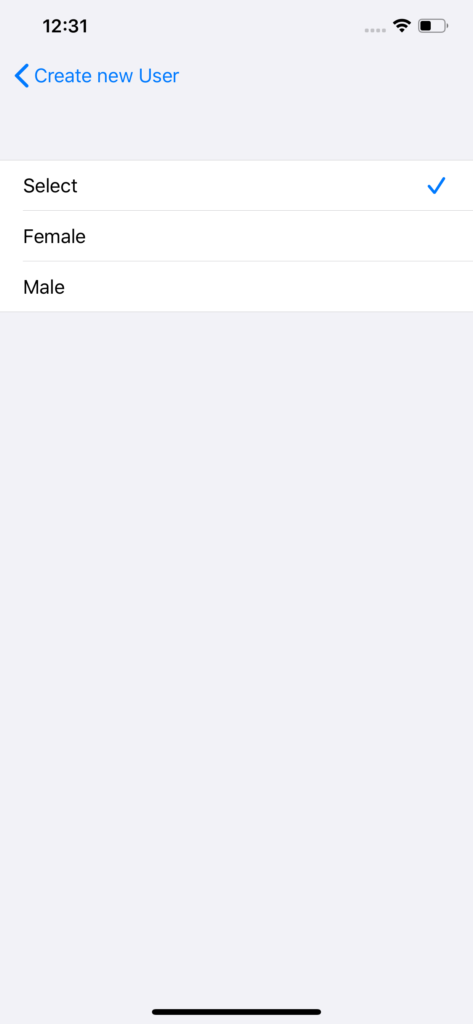
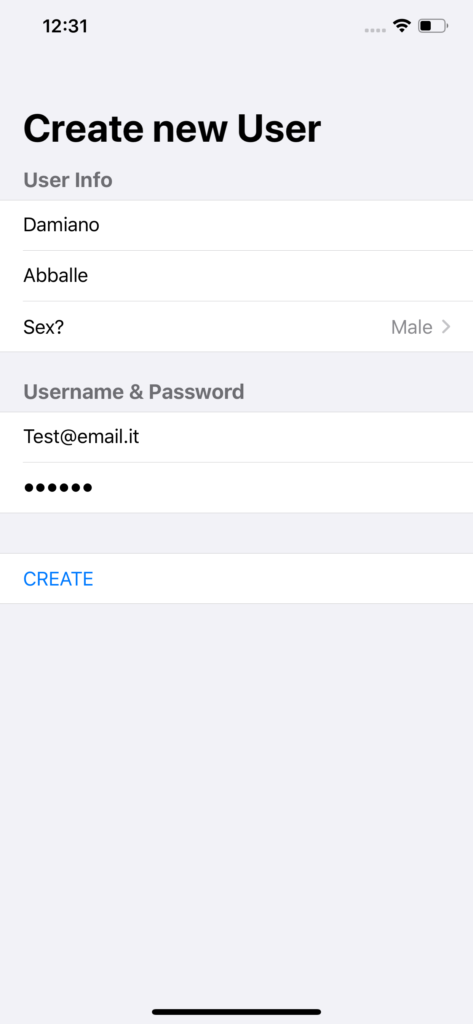
[PAGEFORM.SWIFT]
import SwiftUI
struct PageForm: View {
// definition of variables
@State private var email:String = ""
@State private var password: String = ""
@State private var firstName:String = ""
@State private var lastName:String = ""
@State private var showingSummary = false
// definition of Picker's items
let sexTypes = ["Select","Female", "Male"]
// definition of the variable where we will save the value selected
@State private var sexType = 0
// definition of the "SendData" function, called
// when the button "Create" is pushed
func SendData(){
showingSummary = true
}
// The form will disabled until all filed are filled
var disabledForm: Bool{
firstName.isEmpty || lastName.isEmpty || email.isEmpty || password.isEmpty || sexType==0
}
var body: some View {
NavigationView {
// Form definition
Form {
// Definition of User Info section
Section(header: Text("User Info").fontWeight(.bold).font(.system(size: 18))) {
TextField("First Name", text: $firstName)
TextField("Last Name", text: $lastName)
// Definition of the Picker
Picker("Sex?", selection: $sexType)
{
// Picker's items
ForEach(0..<self.sexTypes.count)
{
Text(self.sexTypes[$0])
}
}
}
// Definition of Username & Password section
Section(header: Text("Username & Password").fontWeight(.bold).font(.system(size: 18))) {
TextField("Email", text: $email)
SecureField("Password", text: $password)
}
Section {
Button(action: SendData) {
Text("CREATE")
}
} // by default the section is disabled
.disabled(disabledForm)
}.navigationBarTitle(Text("Create new User"))
// when Form is enabled, and Enter button clicked, the system will
// show an alert
.alert(isPresented: $showingSummary) {
Alert(title: Text("Your Password is"), message: Text("\(password)"), dismissButton: .default(Text("OK")))
}
}
}
}
struct PageForm_Previews: PreviewProvider {
static var previews: some View {
PageForm()
}
}