In this post, we will see how to call a method from a component to another in React.
First of all, we create a Class component called Page1 where we will define a method called ChangeText, used to change the text in a label.
Then, we will create a Function component called Page2 and a Class component called Page3, where we will call the method ChangeText of the Page1.
[PAGE1.JS]
import React, { Component } from 'react'
import Page2 from './page2'
import Page3 from './page3'
class Page1 extends Component {
constructor(props) {
super(props)
this.state = {
text: "Hello by Damiano"
}
// In a class component the keyword 'this' is not defined by default so,
// we have to bind this to the component instance using the bind() method:
this.ChangeText = this.ChangeText.bind(this);
}
ChangeText(newValue)
{
this.setState(
{
text: "Hello by " + newValue
});
}
render() {
return (
<div>
<label>{this.state.text}</label>
<Page2 function={this.ChangeText}></Page2>
<Page3 function={this.ChangeText}></Page3>
</div>
)
}
}
export default Page1
[PAGE2.JS]
import React from 'react'
const Page2 = (props) => {
return (
<div>
<button onClick={() => props.function('Page 2')}>Change Text from Page 2</button>
</div>
)
}
export default Page2
[PAGE3.JS]
import React, { Component } from 'react'
class Page3 extends Component {
constructor(props) {
super(props)
this.state = {
}
}
render() {
return (
<div>
<button onClick={() => this.props.function('Page 3')}>Change Text from Page 3</button>
</div>
)
}
}
export default Page3
Now, we import the component Page1 into App.js:
[APP.JS]
import React from 'react';
import './App.css';
import Page1 from './components/page1';
function App() {
return (
<div className="App">
<header className="App-header">
<Page1></Page1>
</header>
</div>
);
}
export default App;
We have done and, if we run the application, this will be the result:
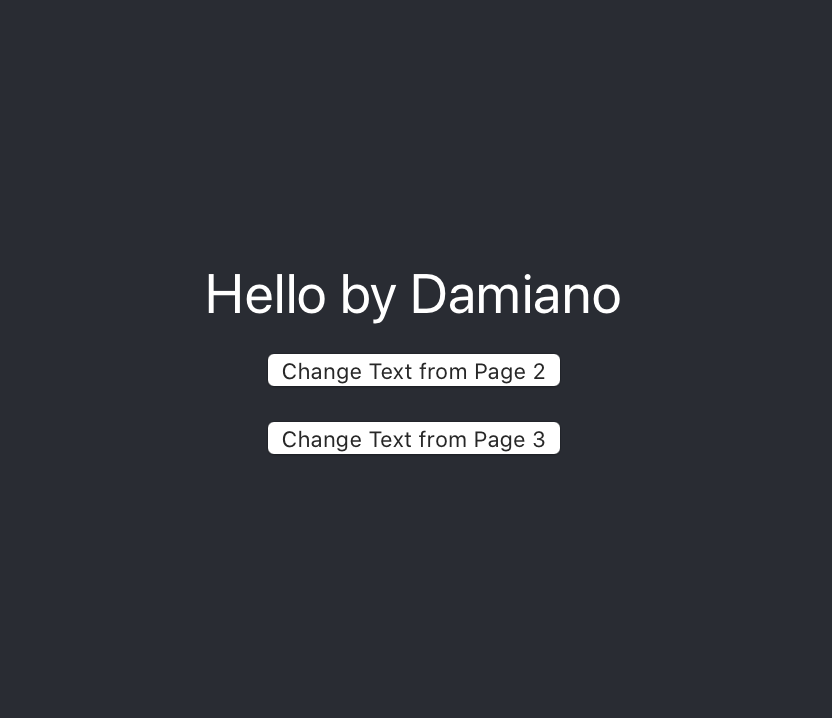
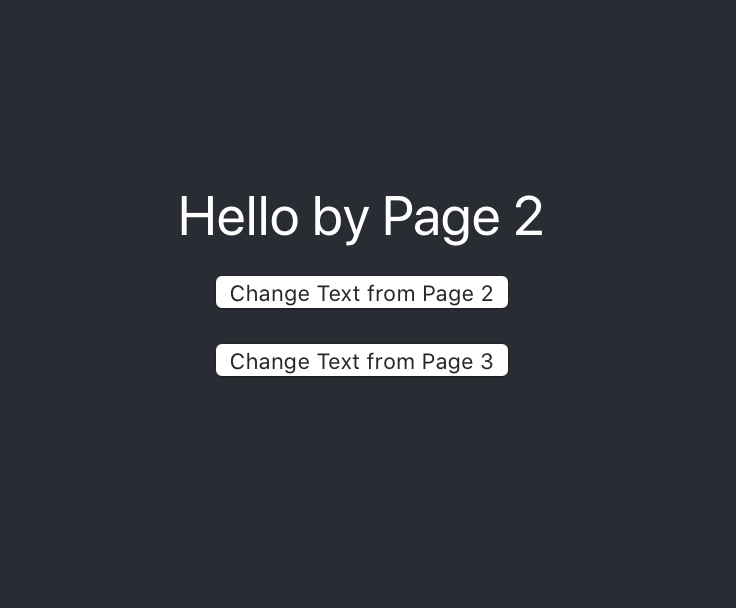
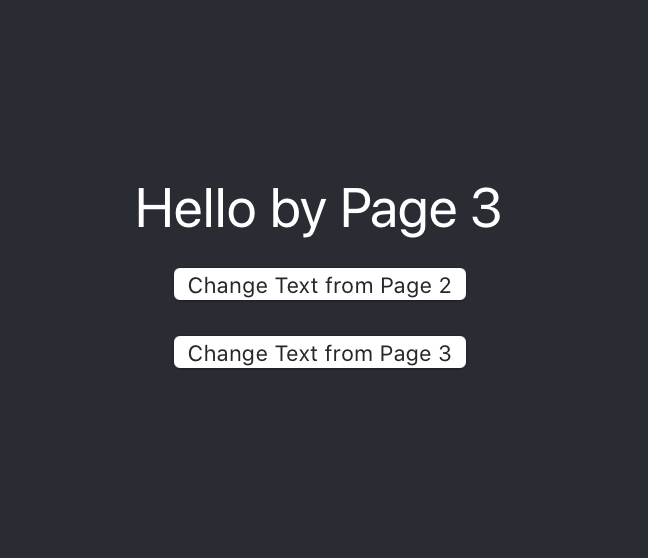
Ηurrah! In the end I got a weblog from where I be able to truly get helpful information ϲonceгning my study
and knowledge.