In this post, we will see how to manage onClick event in React.
FUNCTION COMPONENT:
We create a function component called FunctionEvent:
[FUNCTIONEVENT.JS]
import React from 'react'
const FunctionEvent = () => {
function ShowAlert1()
{
alert("Test One Function")
}
function ShowAlert2()
{
alert("Test Two Function")
}
return(
<div>
<button onClick={ShowAlert1}>Show Alert 1</button>
<br/>
<button onClick={() => ShowAlert2()}>Show Alert 2</button>
</div>
)
}
export default FunctionEvent;
Then, we add this component into App.js:
[APP.JS]
import React from 'react';
import './App.css';
import FunctionEvent from './components/FunctionEvent';
function App() {
return (
<div className="App">
<header className="App-header">
<FunctionEvent></FunctionEvent>
</header>
</div>
);
}
export default App;
If we run the application, this will be the result:
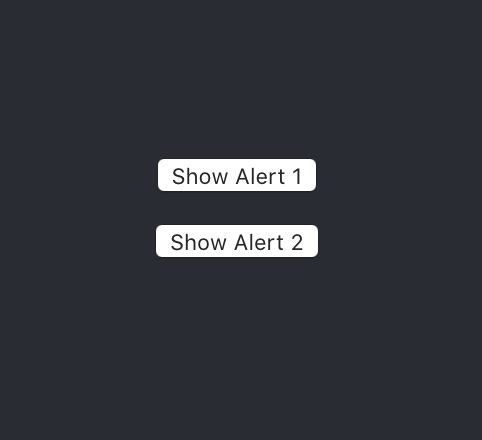
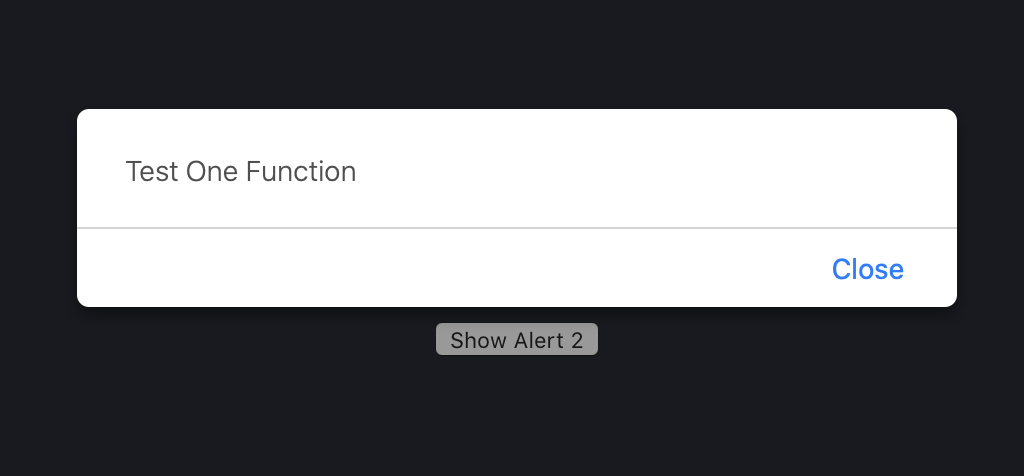
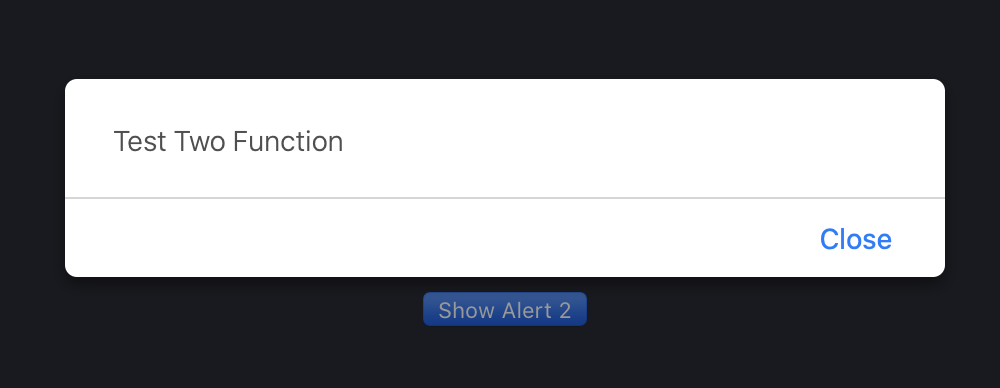
CLASS COMPONENT:
We create a Class component called ClassEvent
[CLASSEVENT.JS]
import React, { Component } from 'react'
class ClassEvent extends Component {
ShowAlert3()
{
alert("Test Three Class")
}
ShowAlert4()
{
alert("Test Four Class")
}
render() {
return (
<div>
<button onClick={() => this.ShowAlert3()}>Show Alert 3</button>
<br/>
<button onClick={this.ShowAlert4}>Show Alert 4</button>
</div>
)
}
}
export default ClassEvent
Then, we add this component into App.js:
[APP.JS]
import React from 'react';
import './App.css';
import ClassEvent from './components/ClassEvent';
function App() {
return (
<div className="App">
<header className="App-header">
<ClassEvent></ClassEvent>
</header>
</div>
);
}
export default App;
If we run the application, this will be the result:
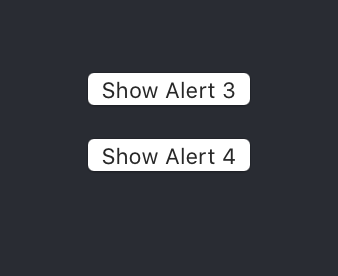
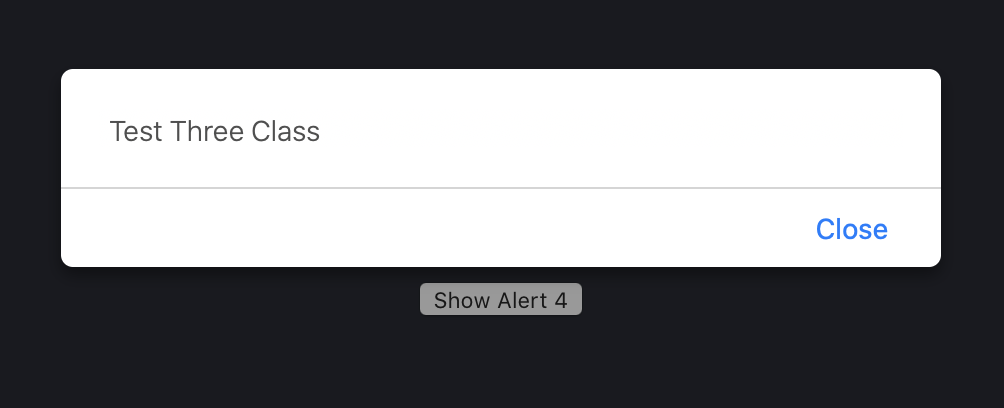
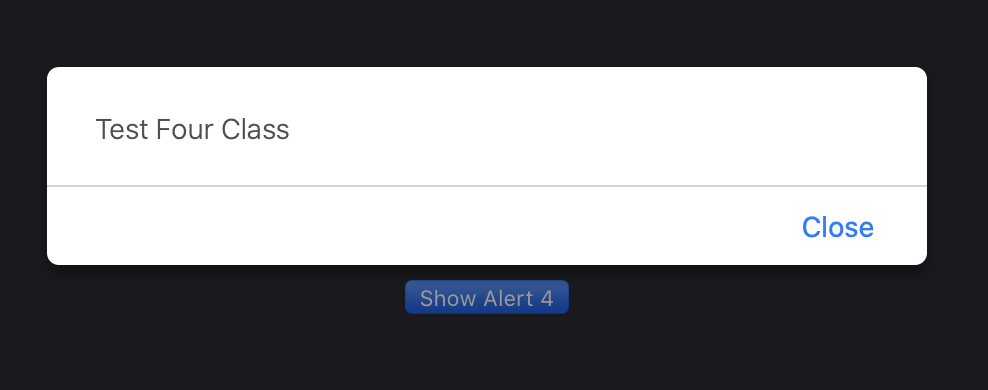