In this post, we will see how to add Logging in the project Api.Orders created in the post: Web API – How to use Polly library with Ocelot.
Logging is a built-in feature of ASP.NET Core and .NET Core Work Services and it is provided as part of the Microsoft.Extensions.Logging library.
For more information: Microsoft Web Site
First of all, we open the Api.Orders project and we install the Serilog.Extensions.Logging.File library that it will allow us to write the log in a file.
Then, we open the Startup file and we define the name of the log file:
[STARTUP.CS]
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, ILoggerFactory loggerFactory)
{
// Definition of the file where we will save the log.
loggerFactory.AddFile($"Logs/Orders-{DateTime.Now.ToLongDateString()}.txt");
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
Finally, we will add Logging in the method GetOrdersAsync of CoreOrders:
[COREORDERS.CS]
using Api.Orders.Core.Interfaces;
using Api.Orders.Db;
using Microsoft.Extensions.Logging;
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace Api.Orders.Core.Providers
{
public class CoreOrder : ICoreOrder
{
// Definition of Logger
private readonly ILogger<CoreOrder> logger;
private readonly IBooksService booksService;
private readonly ICustomersService customersService;
public CoreOrder(IBooksService inputBooksService, ICustomersService inputCustomersService, ILogger<CoreOrder> inputLogger)
{
booksService = inputBooksService;
customersService = inputCustomersService;
// With dependency injection, we create the logger<CoreOrder> object
logger = inputLogger;
}
public async Task<(bool IsSuccess, IEnumerable<Order> Orders, string ErrorMessage)> GetOrdersAsync()
{
List<Order> lstOrder = new List<Order>();
try
{
for (int i = 1; i < 6; i++)
{
var objOrder = new Order();
objOrder.Id = i;
objOrder.OrderDate = DateTime.Now.AddDays(-i);
var resultCustomerService = await customersService.GetCustomer(i);
if (resultCustomerService.IsSuccess)
{
objOrder.EmailCustomer = resultCustomerService.ObjCustomer.Email;
}
var lstOrderItems = new List<OrderItem>();
for (int y = i; y < (i + 3); y++)
{
var orderItem = new OrderItem();
var resultBookService = await booksService.GetBook(y);
if (resultBookService.IsSuccess)
{
// we add a log to know the workflow of the app
logger?.LogInformation($"Called with success BookService using id:{y}");
orderItem.BookTitle = resultBookService.ObjBook.Title;
orderItem.Price = resultBookService.ObjBook.Price;
}
else
{
// we add a log to know the workflow of the app
logger?.LogInformation($"BookService didn't give result. Id:{y}");
orderItem.BookTitle = "Book information is not available";
orderItem.Price = 0;
}
orderItem.Quantity = y;
objOrder.Total = objOrder.Total + (orderItem.Price * orderItem.Quantity);
lstOrderItems.Add(orderItem);
}
objOrder.Books = new List<OrderItem>();
objOrder.Books.AddRange(lstOrderItems);
lstOrder.Add(objOrder);
}
return (true, lstOrder, null);
}
catch(Exception ex)
{
// we add a log in case of exception
logger?.LogError(ex.ToString());
return (false, null, ex.Message);
}
}
}
}
We have done and, if we run the application, we will be able to read the log file:
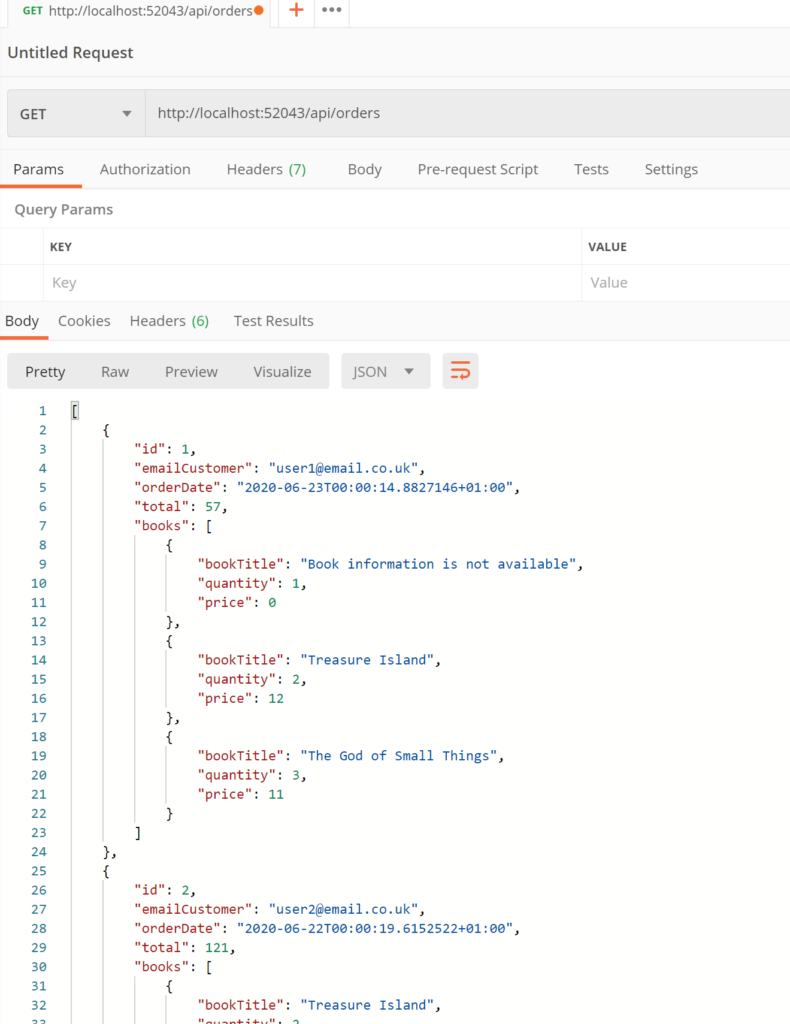

