In this post, we will see how to use Hooks in React.
But, what are Hooks?
From React web site:
“Hooks are a new addition in React 16.8. They let you use state and other React features without writing a class.“
STATE HOOKS
We open Visual Studio Code and we create a file called State.js:
[STATE.JS]
// In order to use Hook, we have to import useState
import React, { useState } from 'react';
const State = () => {
// Definition of a variable called total that at the beginning values 0
// addValueTotal is a method used to change the value of total
const [total, addValueTotal] = useState(0);
return (
<div>
<p>Total clicks {total}</p>
<button onClick={() => addValueTotal(total + 1)}>
Push
</button>
</div>
)
}
export default State;
Now, if we run the application, after we have added the file in App.js, this will be the result:
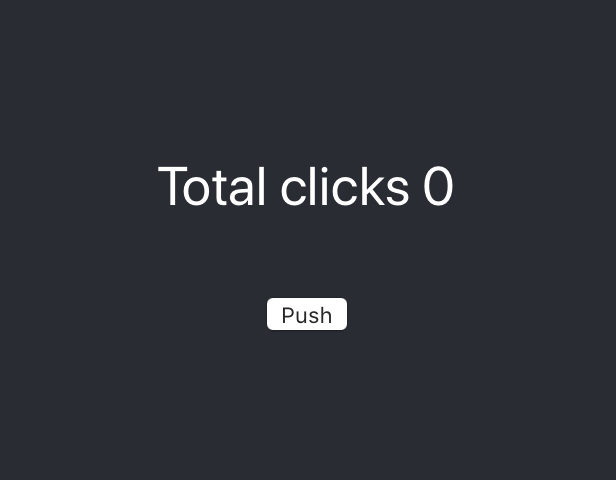
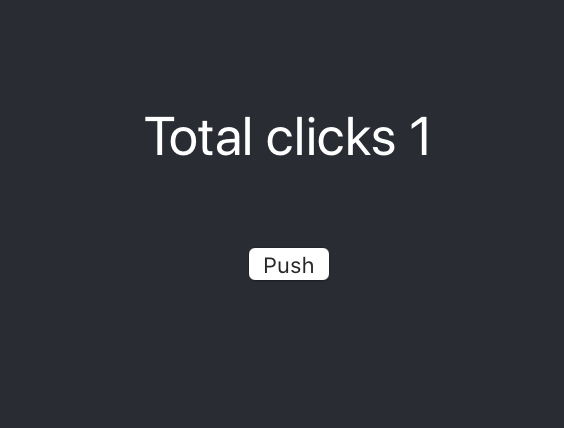
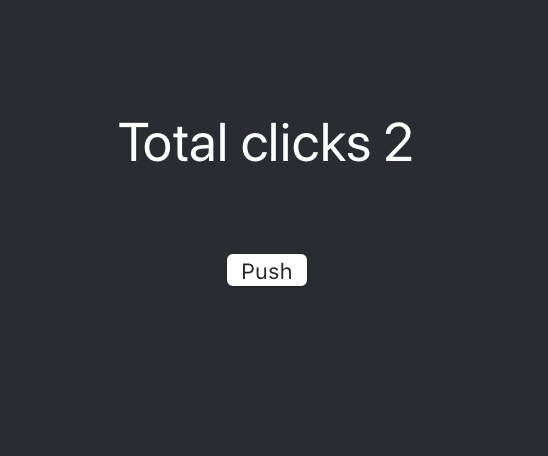
EFFECT HOOKS
Effect Hooks, help us when we want to run some additional code after React has updated the DOM.
We open Visual Studio Code and we create a file called PrintMessage:
[PRINTMESSAGE.JS]
// In order to use Hooks, we have to import useState and useEffect
import React, { useState, useEffect } from 'react';
const PrintMessage = () => {
// We define a variable called total that at the beginning the value is 0
// addValueTotal is a method used to change the value at the variable total
const [total, addValueTotal] = useState(0);
// Function used to write log
function WriteLog(val)
{
console.log("The value of total is: " + val.total);
}
// Everytime button is pressed, the function WriteLog is called
useEffect(() => WriteLog({total}));
return (
<div>
<p>Total clicks {total}</p>
<button onClick={() => addValueTotal(total + 1)}>
Push
</button>
</div>
)
}
export default PrintMessage
Now, if we run the application, after we have added the file in App.js, this will be the result:
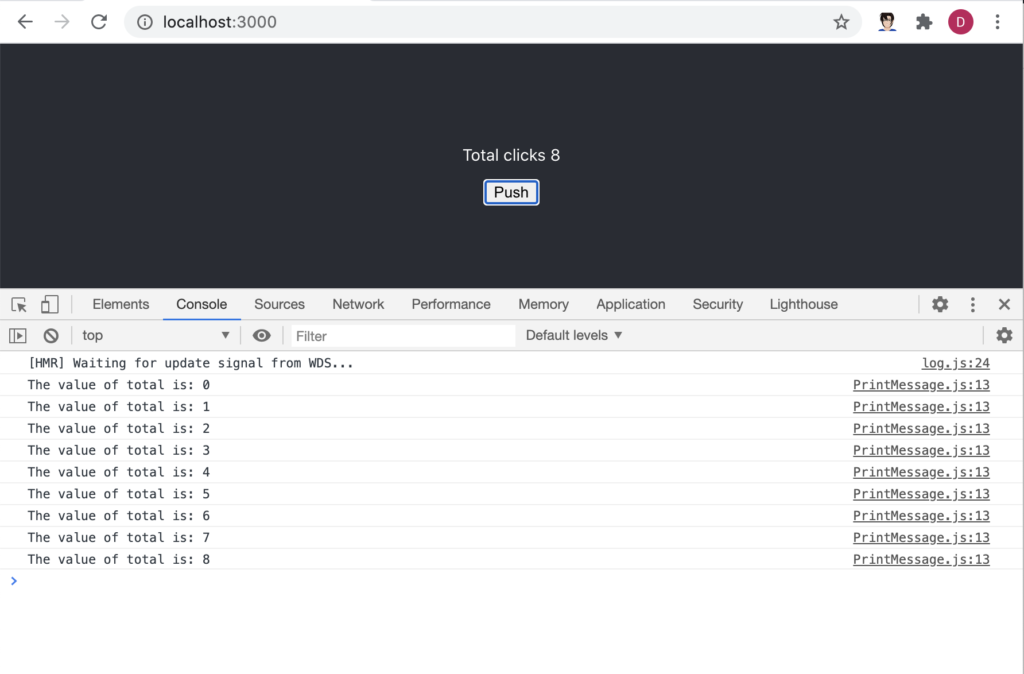
Perfect! Thanks!!!