In this post, we will see how to fetch data in a Class component.
In the React web site, they advise to use the method componentDidMount() because,
“is invoked immediately after a component is mounted (inserted into the tree). Initialization that requires DOM nodes should go here. If you need to load data from a remote endpoint, this is a good place to instantiate the network request.“
We start creating a Class component called GetListUsers, where we will define the Web API’s url, that we will use in this post:
[GETLISTUSERS.JS]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | import React, { Component } from 'react' // definition of the Web API's url const APIUrl = "https://webapidocker.azurewebsites.net/users" ; class GetListUsers extends Component { render() { return ( <div> <h1>List users</h1> </div> ) } } export default GetListUsers |
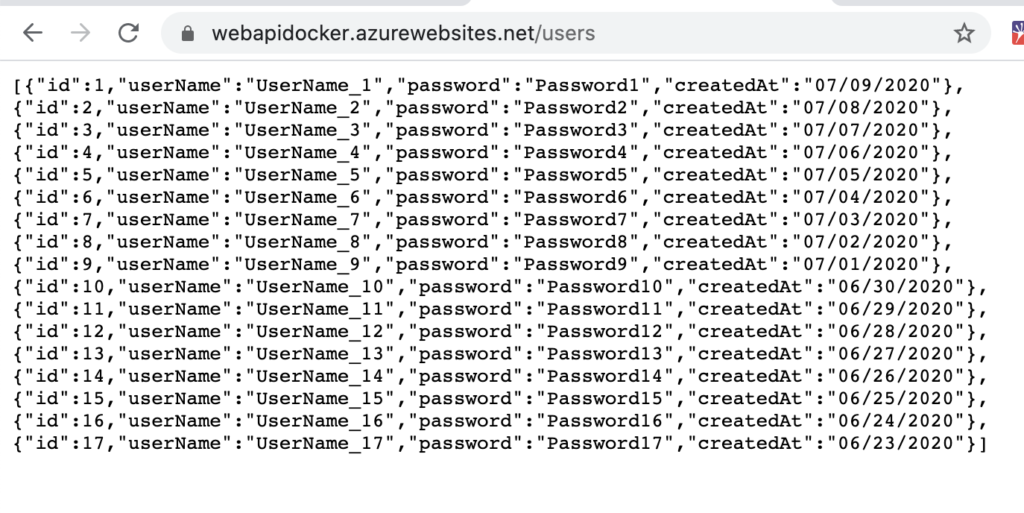
If we run the application, this will be the result:
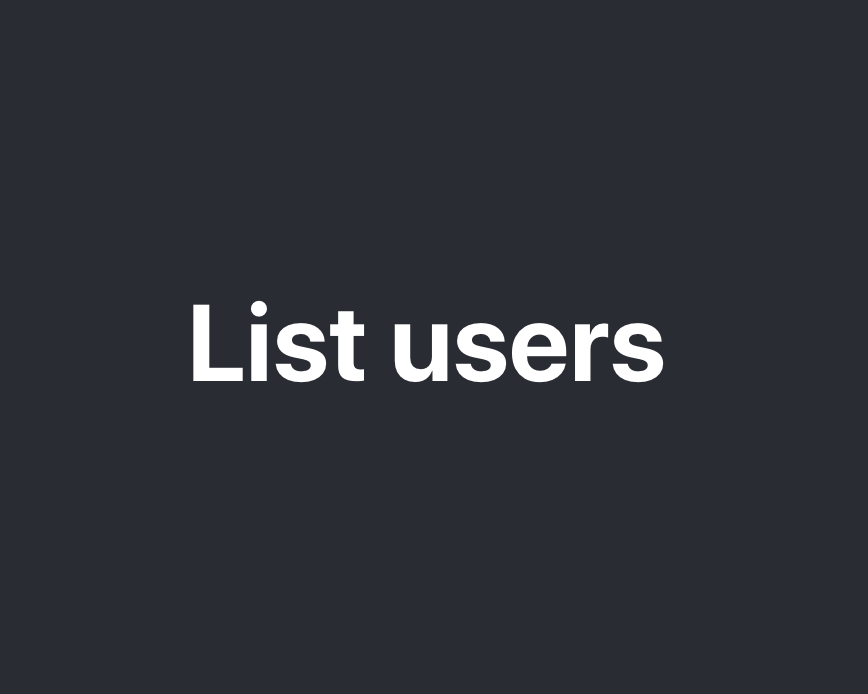
Now, we create a Function component called HTMLUserList, where we will define the code to display the list of users:
[HTMLUSERLIST.JS]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | import React from 'react' const HTMLUserList = ({users}) => { return ( <div style = {{width: "100%" }}> <table> <thead> <tr> <th>UserName</th> <th>Password</th> <th>Created At</th> </tr> </thead> <tbody> {users.map(user => <tr key={user.id}> <td>{user.userName}</td> <td>{user.password}</td> <td>{user.createdAt}</td> </tr> )} </tbody> </table> </div> ) } export default HTMLUserList; |
Then, we add in index.css, the css code used to define the table’s style:
[INDEX.CSS]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | .App { text-align : center ; } .App-logo { height : 40 vmin; pointer-events: none ; } @media (prefers-reduced-motion: no-preference) { .App-logo { animation : App-logo-spin infinite 20 s linear ; } } .App-header { background-color : #282c34 ; min-height : 100 vh; display : flex ; flex-direction : column; align-items : center ; justify-content : center ; font-size : calc( 10px + 2 vmin); color : white ; } .App-link { color : #61dafb ; } @keyframes App-logo-spin { from { transform : rotate ( 0 deg); } to { transform : rotate ( 360 deg); } } |
Finally, we modify the component GetListUsers, in order to fetch data and show the list of users:
[GETLISTUSERS.JS]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | import React, { Component } from 'react' import ViewListUsers from './HTMLUserList' ; // definition of the Web API's url const APIUrl = "https://webapidocker.azurewebsites.net/users44" ; class GetListUsers extends Component { // definition of variables constructor() { super (); this .state = { lstUsers: [], loading: true , error: null , }; } // definition of componentDidMount method componentDidMount() { fetch(APIUrl) // check the response .then(response => { if (response.ok) { return response.json(); } else { this .setState({ error: 'error', loading: true }); } }) .then(data => this .setState({ lstUsers: data, loading: false })) . catch (error => this .setState({ error: error, loading: false })); } render() { // Using the Destructuring assignment, we define three variables used in the component const { lstUsers, loading, error } = this .state; // in case of error, component will display an error message if (error) { return <h1 style = {{color: "white" }}>Attention! There are problems with the Web API.</h1>; } return ( <div> <h1 style = {{color: "white" }}>List users</h1> {loading ? <h2 style = {{color: "white" }}>Loading...</h2>: <ViewListUsers users={lstUsers}/> } </div> ) } } export default GetListUsers |
Now, if we run the application, this will be the result:
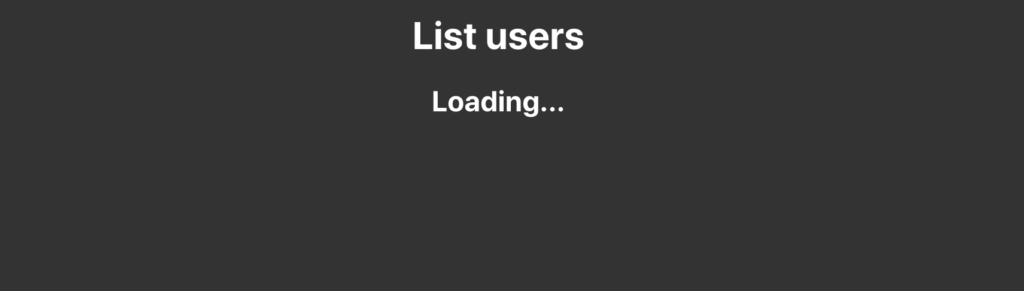
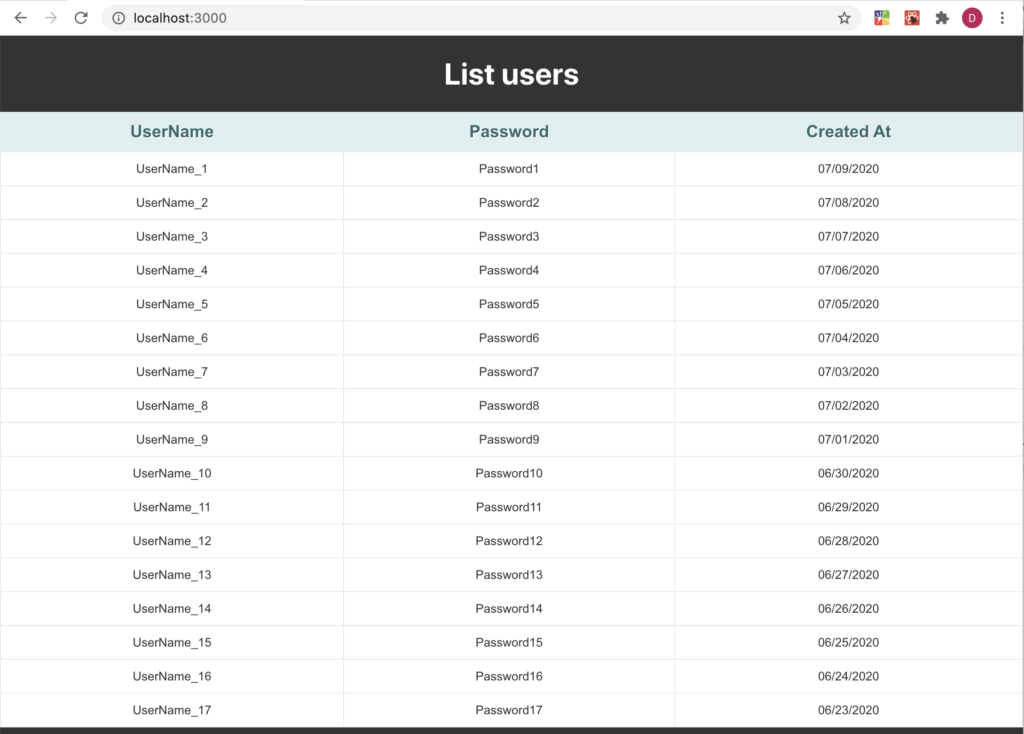