In this post, we will see how to create a Form in React in order to manage a login formed by Username, Password and UserType.
First of all, we create a file called reactform where we will define the form:
import React, { Component } from 'react'
class ReactForm extends Component {
render() {
return (
<form>
<div>
</div>
</form>
)
}
}
export default ReactForm
Then, we define two input HTML objects for handling Username and Password:
<div>
<label>Username: </label>
<input type="text"></input>
</div>
<div>
<label>Password: </label>
<input type="password"></input>
</div>
Finally, we define a Select HTML object for handling UserType:
<select>
<option>
</option>
</select>
Now, we define the variables and the methods used to manage the values in the HTML objects defined above:
constructor(props) {
super(props)
this.state = {
username:'',
password:'',
typeuser:'2',
lstoptions: [{id:0,text:'Admin'}, {id:1,text:'Tester'}, {id:2,text:'Reader'}]
}
}
ManageUsername = (event) =>
{
this.setState({
username: event.target.value
})
}
ManagePassword = (event) =>
{
this.setState({
password: event.target.value
})
}
ManageUserType = (event) =>
{
this.setState({
typeuser: event.target.value
})
}
Then, we add the variables and the methods in the HTML objects:
<div>
<label>Username: </label>
<input type="text" value={this.state.username} onChange={this.ManageUsername} ></input>
</div>
<div>
<label>Password: </label>
<input type="password" value={this.state.password} onChange={this.ManagePassword} ></input>
</div>
<div>
<select value={this.state.typeuser} onChange={this.ManageUserType}>
{
this.state.lstoptions.map(optionitem => (
<option key={optionitem.id} value={optionitem.id}>
{optionitem.text}
</option>
))}
</select>
</div>
Finally, we add the method used in the FormSubmited and a button to submit the data:
ViewForm = (event) =>
{
alert(this.state.username + " " + this.state.password + " " + this.state.typeuser)
// it is used to avoid lost data in the form after the submit
event.preventDefault()
}
<form onSubmit={this.ViewForm}>
<div>
<button type="submit">View Data</button>
</div>
</form>
Before to run the application, we have to add the reactform in App.js:
[APP.JS]
import React from 'react';
import './App.css';
import ReactForm from './components/reactform';
function App() {
return (
<div className="App">
<header className="App-header">
<ReactForm></ReactForm>
</header>
</div>
);
}
export default App;
Now, if we run the application, this will be the result:
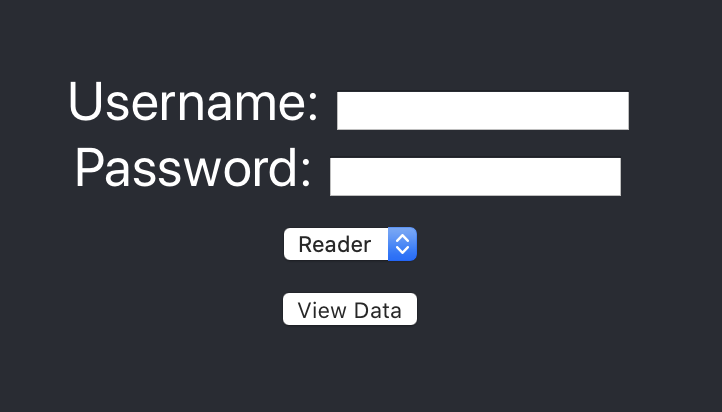


[REACTFORM.JS]
import React, { Component } from 'react'
class ReactForm extends Component {
constructor(props) {
super(props)
this.state = {
username:'',
password:'',
typeuser:'2',
lstoptions: [{id:0,text:'Admin'}, {id:1,text:'Tester'}, {id:2,text:'Reader'}]
}
}
ManageUsername = (event) =>
{
this.setState({
username: event.target.value
})
}
ManagePassword = (event) =>
{
this.setState({
password: event.target.value
})
}
ManageUserType = (event) =>
{
this.setState({
typeuser: event.target.value
})
}
ViewForm = (event) =>
{
alert(this.state.username + " " + this.state.password + " " + this.state.typeuser)
// it is used to avoid lost data in the form after the submit
event.preventDefault()
}
render() {
return (
<form onSubmit={this.ViewForm}>
<div>
<label>Username: </label>
<input type="text" value={this.state.username} onChange={this.ManageUsername} ></input>
</div>
<div>
<label>Password: </label>
<input type="password" value={this.state.password} onChange={this.ManagePassword} ></input>
</div>
<div>
<select value={this.state.typeuser} onChange={this.ManageUserType}>
{this.state.lstoptions.map(optionitem => (
<option
key={optionitem.id}
value={optionitem.id}
>
{optionitem.text}
</option>
))}
</select>
</div>
<div>
<button type="submit">View Data</button>
</div>
</form>
)
}
}
export default ReactForm