In this post, we will see some interesting methods to use with String.
HOW TO REVERSE A STRING
public static string ReverseWord(string strInput)
{
StringBuilder resultStr = new StringBuilder();
int startI = strInput.Length - 1;
for (int i = startI; i >= 0; i--)
{
resultStr.Append(strInput[i]);
}
return resultStr.ToString();
}
static void Main(string[] args)
{
string strInput = "Damiano Abballe";
Console.WriteLine(strInput);
Console.WriteLine(ReverseWord(strInput));
}
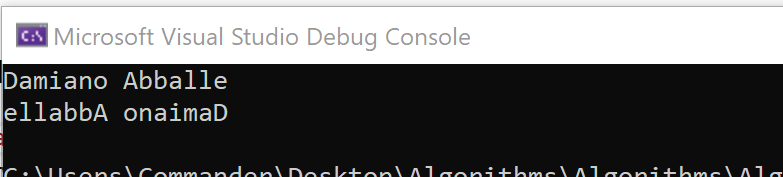
HOW TO REVERSE THE ORDER OF WORDS IN A GIVEN STRING
public static string ReverseString(string strInput)
{
StringBuilder resultStr = new StringBuilder();
var arrayInput = strInput.Split(' ');
int startI = arrayInput.Length - 1;
for (int i = startI; i >= 0; i--)
{
resultStr.Append($"{arrayInput[i]} ");
}
return resultStr.ToString().Trim();
}
static void Main(string[] args)
{
string strInput = "Welcome to my blog";
Console.WriteLine(strInput);
Console.WriteLine(ReverseString(strInput));
}
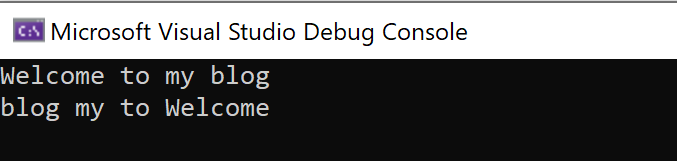
HOW TO COUNT THE OCCURRENCE OF EACH CHARACTER IN A STRING
public static void CountCharacters(string strInput)
{
// With a Regular Expressions, we will remove all space
strInput = Regex.Replace(strInput.ToUpper(), @"\s+", "");
var countChar = new Dictionary<char, int>();
int endI = strInput.Length - 1;
int oldCount = 1;
for (int i = 0; i <= endI; i++)
{
if (countChar.ContainsKey(strInput[i]))
{
oldCount = countChar[strInput[i]];
countChar[strInput[i]] = oldCount + 1;
}
else
{
countChar.Add(strInput[i], 1);
}
}
// Display the Counter
for (int i = 0; i < countChar.Count; i++)
{
Console.WriteLine($"Character: {countChar.ElementAt(i).Key}, Count: {countChar.ElementAt(i).Value}");
}
}
static void Main(string[] args)
{
string strInput = "hello world";
CountCharacters(strInput);
}
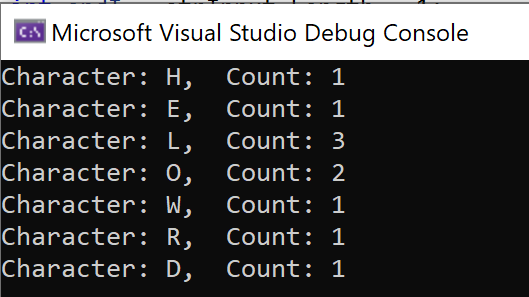
HOW TO FIND ALL POSSIBLE SUBSTRING OF A GIVEN STRING
public static void FindSubstring(string strInput)
{
int currentStart = 0;
int endI = strInput.Length - 1;
int endSubstring = 1;
StringBuilder result = new StringBuilder();
while (currentStart <= strInput.Length - 1)
{
for (int i = currentStart; i <= endI; i++)
{
result.Append($"{strInput.Substring(currentStart, endSubstring)},");
endSubstring++;
}
endSubstring = 1;
currentStart++;
}
Console.WriteLine(result.ToString());
}
static void Main(string[] args)
{
string strInput = "abcd";
Console.WriteLine(strInput);
FindSubstring(strInput);
}
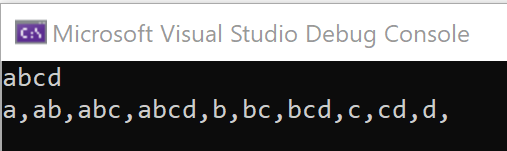
HOW TO REMOVE DUPLICATE CHARACTERS FROM A STRING
public static void RemoveDuplicareCharacters(string inputString)
{
StringBuilder result = new StringBuilder();
HashSet<char> noDuplicate = new HashSet<char>();
foreach (char item in inputString)
{
if (noDuplicate.Add(item))
{
result.Append(item);
}
}
Console.WriteLine(result.ToString());
}
static void Main(string[] args)
{
string inputStr = "Coffee";
Console.WriteLine(inputStr);
RemoveDuplicareCharacters(inputStr);
}
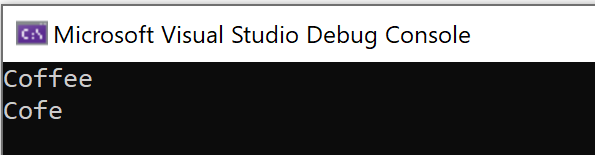