In this post, we will see what a Dynamic Type is and how to use it.
Dynamic Type was introduced in C# 4.0 and it avoids compile-time type checking.
It escapes type checking at compile-time and it resolves type a run time.
A dynamic type variable is defined using the dynamic keyword.
Let see some examples:
DEFINING A DYNAMIC TYPE:
static void Main(string[] args)
{
// we define the value1 as a string
dynamic value1 = "hello";
Console.WriteLine($"The first variable is {value1} and it is a {value1.GetType().ToString()}");
// now, we save an integer
value1 = 3;
Console.WriteLine($"The first variable is {value1} and it is a {value1.GetType().ToString()}");
// finally, we save a boolean
value1 = false;
Console.WriteLine($"The first variable is {value1} and it is a {value1.GetType().ToString()}");
}
If we run this code, this will be the result:
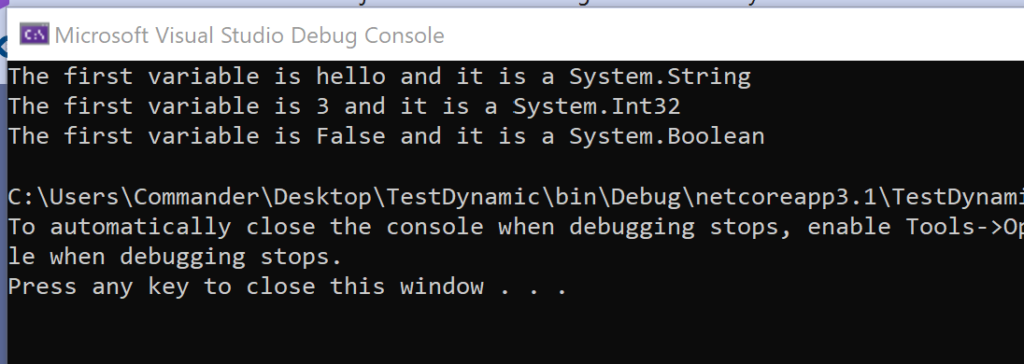
PASSING A DYNAMIC TYPE IN A FUNCTION:
static void Main(string[] args)
{
// we define the value1 as a string
dynamic value1 = "hello";
Console.WriteLine($"The variable is {value1} and it is a {CheckType(value1)}");
// now, we save an integer
value1 = 3;
Console.WriteLine($"The variable is {value1} and it is a {CheckType(value1)}");
// finally, we save a boolean
value1 = false;
Console.WriteLine($"The variable is {value1} and it is a {CheckType(value1)}");
}
private static string CheckType(dynamic inputValue)
{
var valueType = inputValue.GetType();
if (valueType.Equals(typeof(int)))
{
return "Integer";
}
else if (valueType.Equals(typeof(string)))
{
return "string";
}
else
{
return "bool";
}
}
If we run the code, this will be the result:
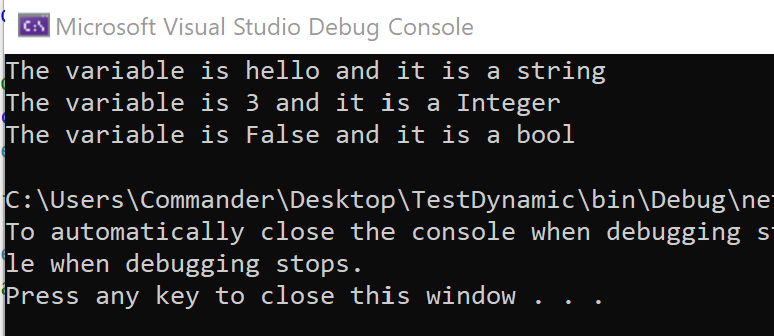
USING A DYNAMIC TYPE AS RETURN TYPE:
static void Main(string[] args)
{
// we define the value1 and value2 as string
dynamic value1 = "hello";
dynamic value2 = "world";
Console.WriteLine($"{Add(value1, value2)}");
// we define the value1 and value2 as integer
value1 = 5;
value2 = 6;
Console.WriteLine($"{Add(value1, value2)}");
// we define the value1 and value2 as decimal
value1 = 12.6;
value2 = 18.1;
Console.WriteLine($"{Add(value1, value2)}");
}
private static dynamic Add(dynamic val1, dynamic val2)
{
dynamic result = val1 + val2;
return result;
}
If we run the code, this will be the result:
