In this post, we will see how to send message in a queue using Azure Service Bus.
But first of all, what is Azure Service Bus?
“Microsoft Azure Service Bus is a fully managed enterprise message broker with message queues and publish-subscribe topics.
If two applications want to communicate with each other with less dependency, then Azure service bus can used to send and receive the messages between them. It can provide your application decoupling and asynchronization with great flexibility as two applications have less dependency with each other. “
We can read all information in the Microsoft Web Site.
Now, we will create a Web API application used to send a message in a queue and a Console application used to read the message.
We go to Azure portal, we select Service Bus and we click the button Create:
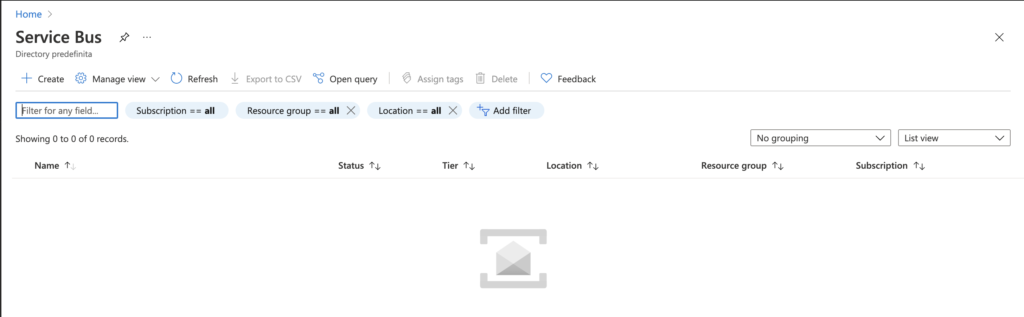
Then, we insert all information to create the Namespace:
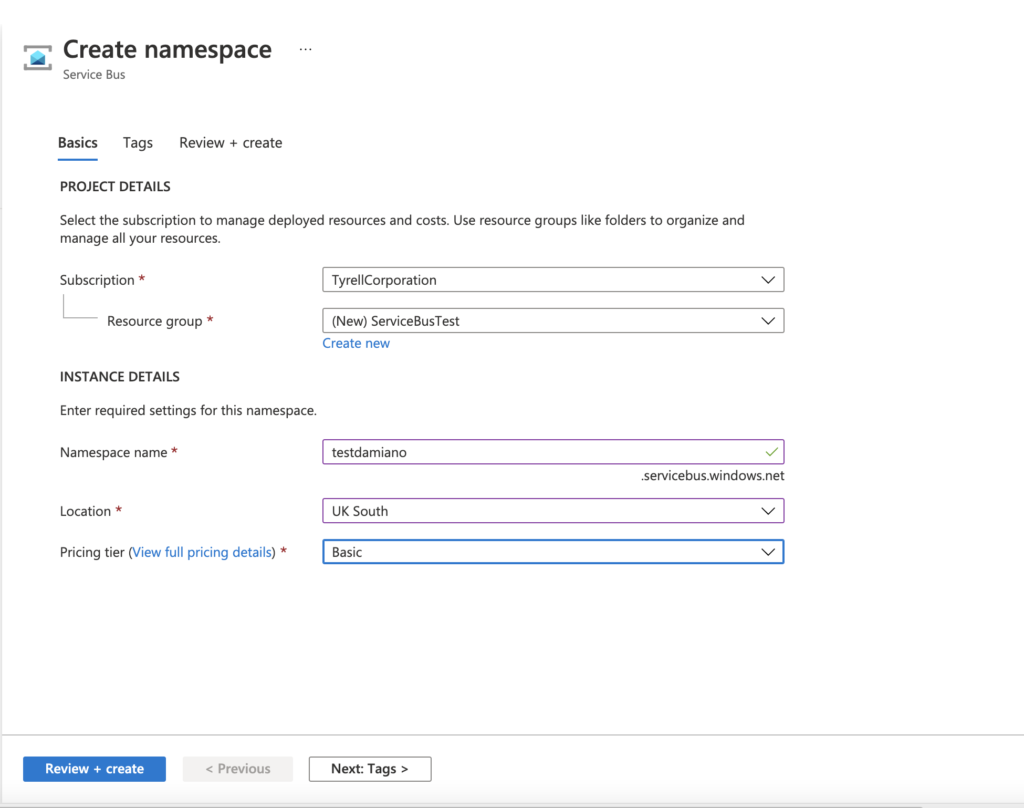
After the creation of Namespace, we go in Entities and we create the Queue:


At the end, we should have a result like that:
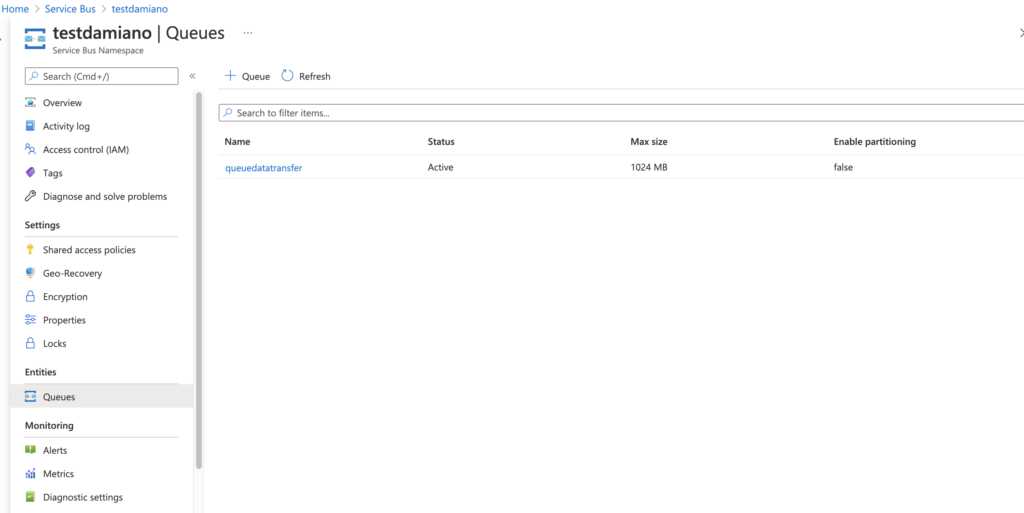
The last thing to do, before start to develop the code, it is to go in Shared access policies and taking the connection string that we will use in our code:

Now, we will create a Web API project used to send message in the queue:
[SECONDCONTROLLER.CS]
using Azure.Messaging.ServiceBus;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace Service.Controllers
{
[Route("[controller]")]
[ApiController]
public class SendController : ControllerBase
{
[HttpGet]
public async Task<ActionResult> WriteQueue(int id)
{
// Definition of Connectionstring and QueueName
string connectionString = "Endpoint=sb://testdamiano.servicebus.windows.net/;SharedAccessKeyName=RootManageSharedAccessKey;SharedAccessKey=mT7/vw25vA8GITCL095g/pVlwTjcQcZpEy230fPjnMA=";
string queueName = "queuedatatransfer";
// Definition of ServiceBus Client
await using var client = new ServiceBusClient(connectionString);
// The sender is responsible for publishing messages to the queue.
ServiceBusSender sender = client.CreateSender(queueName);
ServiceBusMessage message = new ServiceBusMessage(id.ToString());
await sender.SendMessageAsync(message);
return Ok();
}
}
}
We run the WebAPI and, using Postman, we will insert three values (id) in the queue:
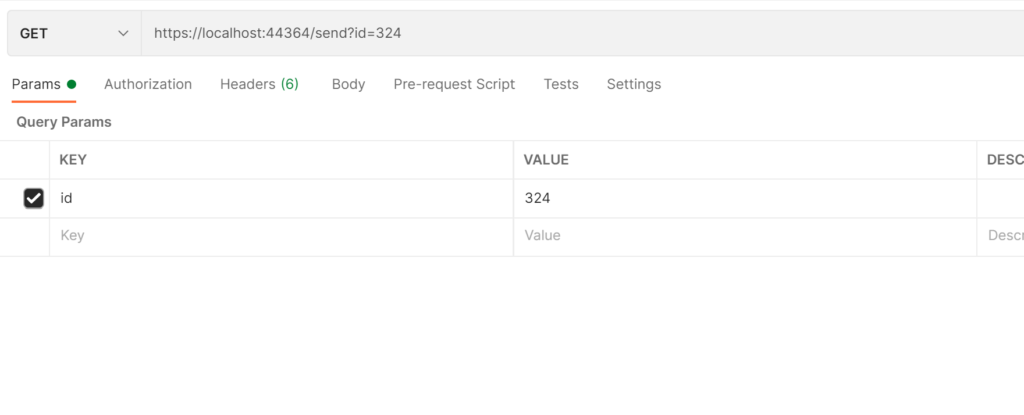
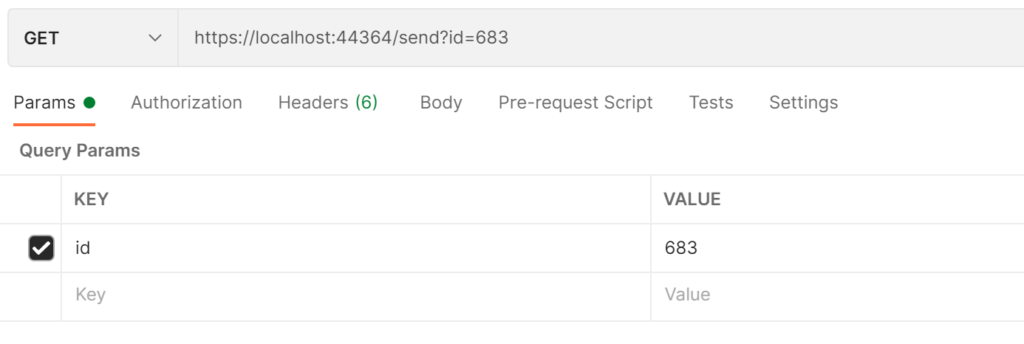

In order to check the queue, we go to Azure portal, we select the queue, click on “Service Bus Explorer” and finally we click on the tab “Receive”:
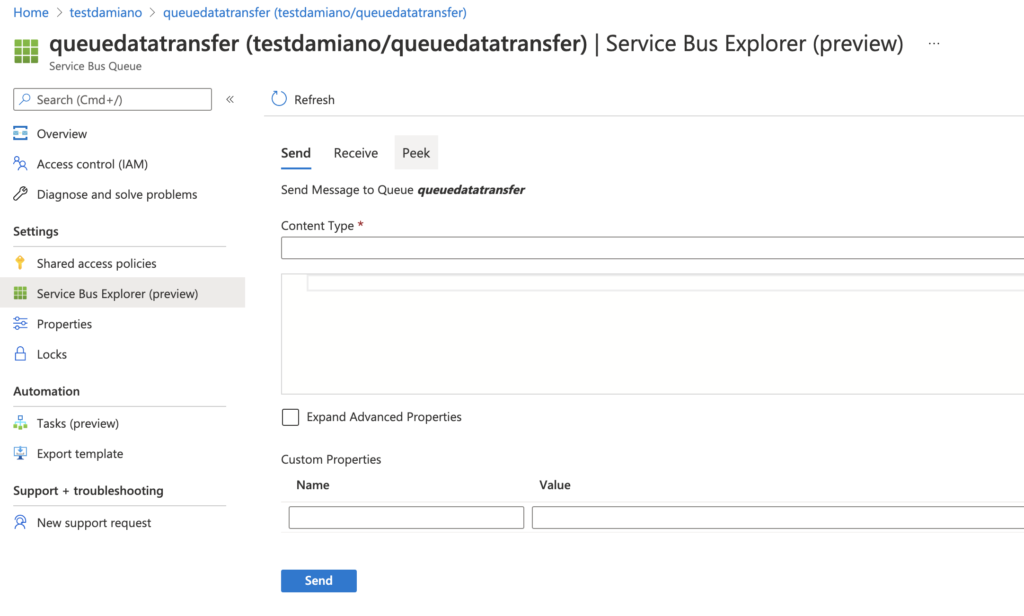
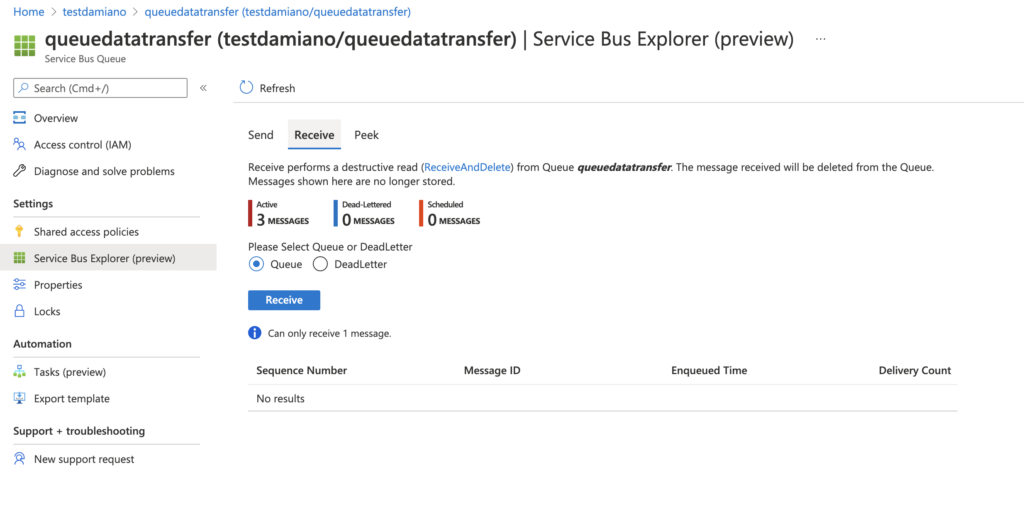
We can see that there are three items.
Now, we will create a Console Application used to read the messages in the queue:
[PROGRAM.CS]
using Microsoft.Azure.ServiceBus;
using System;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
namespace ReadQueue
{
class Program
{
static void Main(string[] args)
{
// Definition of Connectionstring and QueueName
string connectionString = "Endpoint=sb://testdamiano.servicebus.windows.net/;SharedAccessKeyName=RootManageSharedAccessKey;SharedAccessKey=mT7/vw25vA8GITCL095g/pVlwTjcQcZpEy230fPjnMA=";
string queueName = "queuedatatransfer";
var queueClient = new QueueClient(connectionString, queueName);
var messageHandlerOptions = new MessageHandlerOptions(OnException);
// read the queue
queueClient.RegisterMessageHandler(OnMessage, messageHandlerOptions);
Console.WriteLine("Listening, press any key");
Console.ReadKey();
}
// method used to read the message and delete it from the queue
static Task OnMessage(Message m, CancellationToken ct)
{
var messageText = Encoding.UTF8.GetString(m.Body);
Console.WriteLine("The ID in the queue is:");
Console.WriteLine(messageText);
Console.WriteLine($"Enqueued at {m.SystemProperties.EnqueuedTimeUtc}");
return Task.CompletedTask;
}
// method used in order to catch the error
static Task OnException(ExceptionReceivedEventArgs args)
{
Console.WriteLine("Got an exception:");
Console.WriteLine(args.Exception.Message);
Console.WriteLine(args.ExceptionReceivedContext.ToString());
return Task.CompletedTask;
}
}
}
If we run the application, this will be the result:
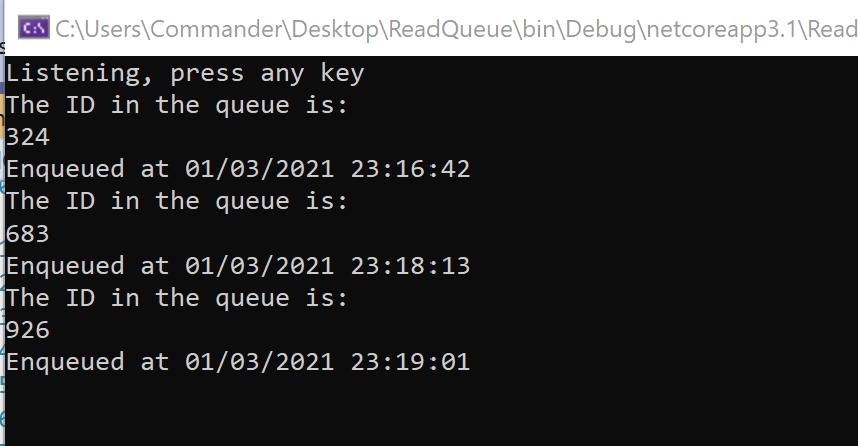
and, if we check again in the Azure portal, we will see that there is nothing in the queue:
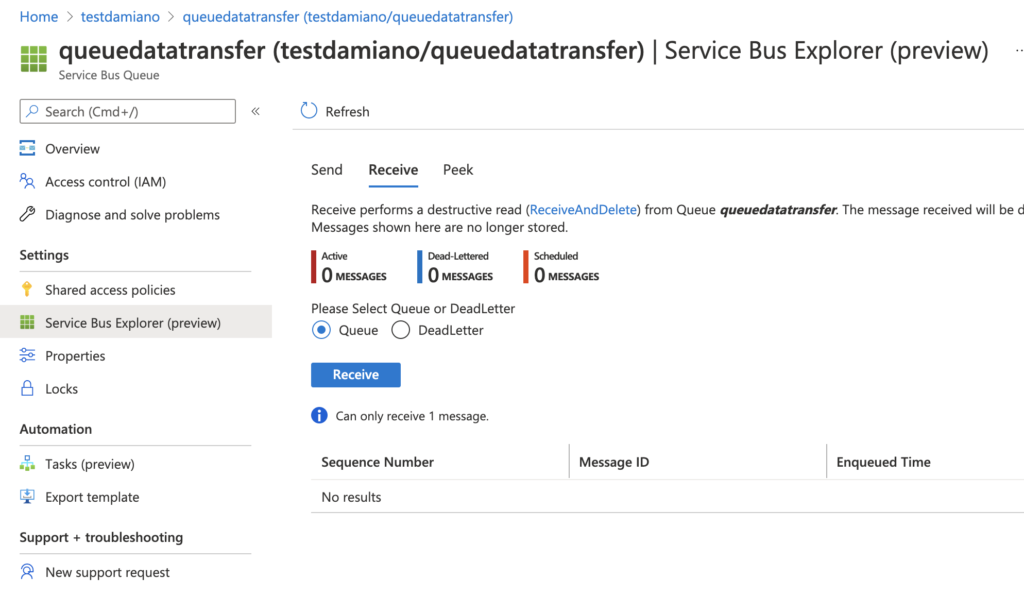