In this post, we will continue to see how to create and consume GraphQL query using .NET Core.
This is the continue of the post: C# – GraphQL API in ASP.NET Core (Part I)
FILTERING
In order to filter a query in GraphQL, we have to do add the attribute [UseFiltering] in Query class and then, we have to add AddFiltering() in the Startup file:
[QUERY.CS]
using HotChocolate;
using HotChocolate.Data;
using SchoolsService.DataAccess.Commands;
using SchoolsService.Model;
using System.Linq;
namespace SchoolsService.GraphQL
{
public class Query
{
[UseProjection]
[UseFiltering]
[UseSorting]
public IQueryable<Student> AllStudents([Service] IStudentCore studentCore)
{
return studentCore.GetAllStudents();
}
[UseProjection]
[UseFiltering]
[UseSorting]
public IQueryable<School> AllSchool([Service] ISchoolCore schoolCore)
{
return schoolCore.GetAllSchools();
}
}
}
[STARTUP.CS]
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ServiceDbContext>(opt => opt.UseInMemoryDatabase("DbService"));
services.AddScoped<IStudentCore, StudentCore>();
services.AddScoped<ISchoolCore, SchoolCore>();
services.AddControllers();
services.AddGraphQLServer()
.AddQueryType<Query>()
.AddProjections()
.AddSorting()
.AddFiltering();
}
Now, if we run some queries, these will be the results:
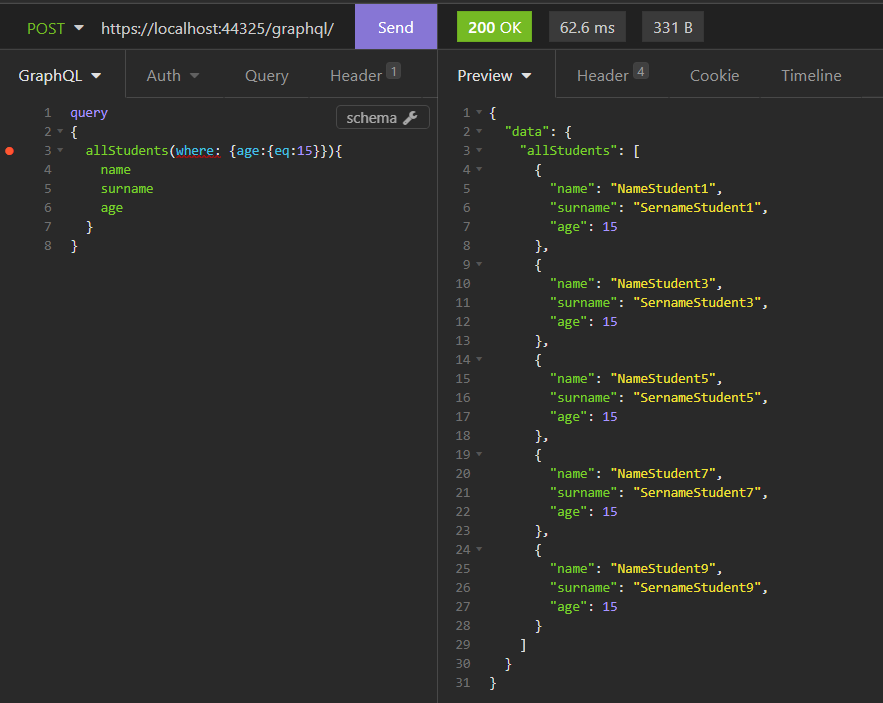
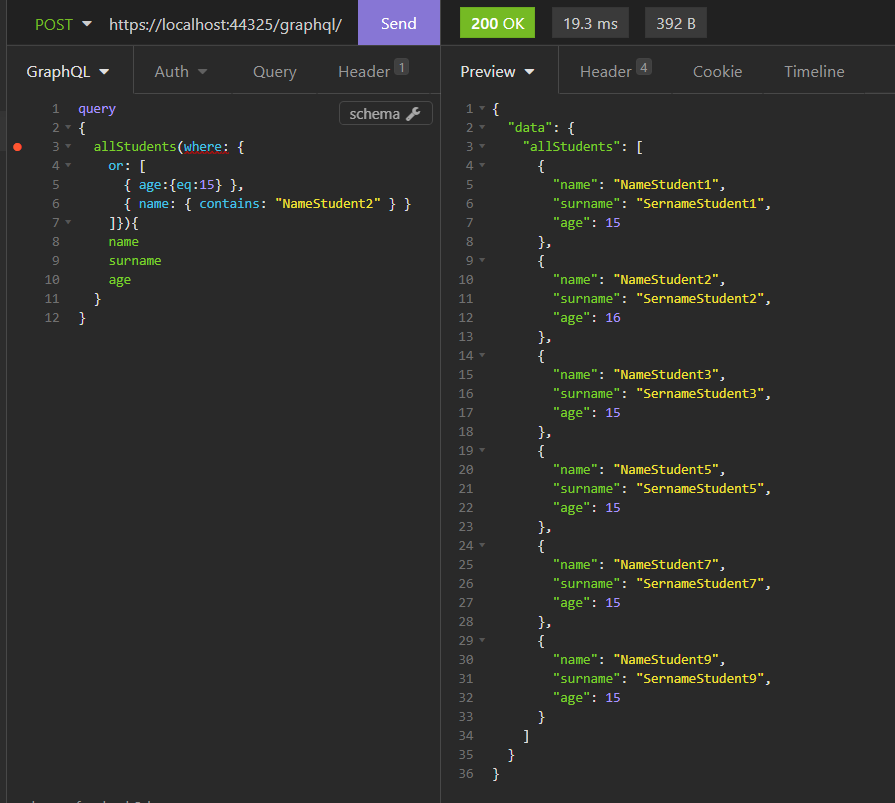
It works fine but, if we try to run this query, we will receive an error message:
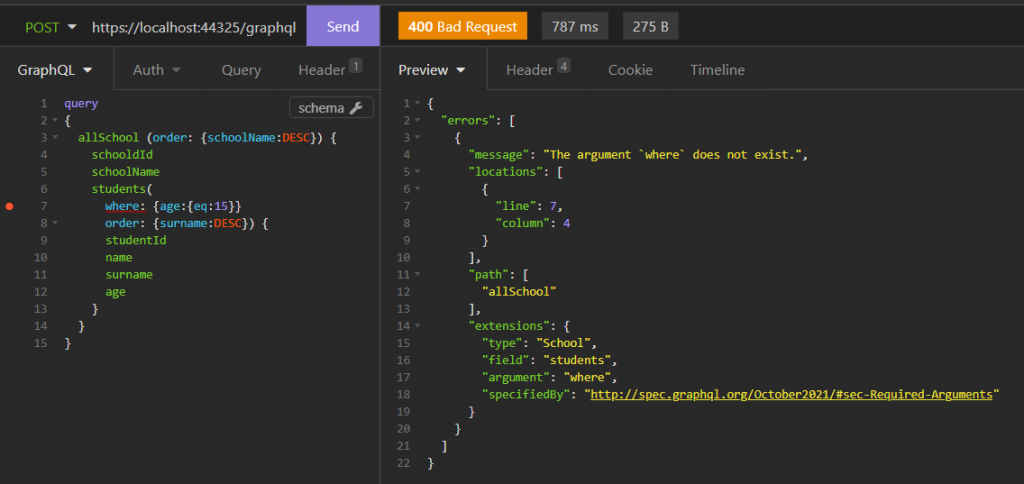
In order to fix this problem, we have to add the attribute [UseFiltering] in the School class as well:
using HotChocolate.Data;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace SchoolsService.Model
{
public class School
{
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int SchooldId { get; set; }
public string SchoolName { get; set; }
[UseSorting]
[UseFiltering]
public ICollection<Student> Students { get; set; }
}
}
Now, if we run again the query, this will be the result:
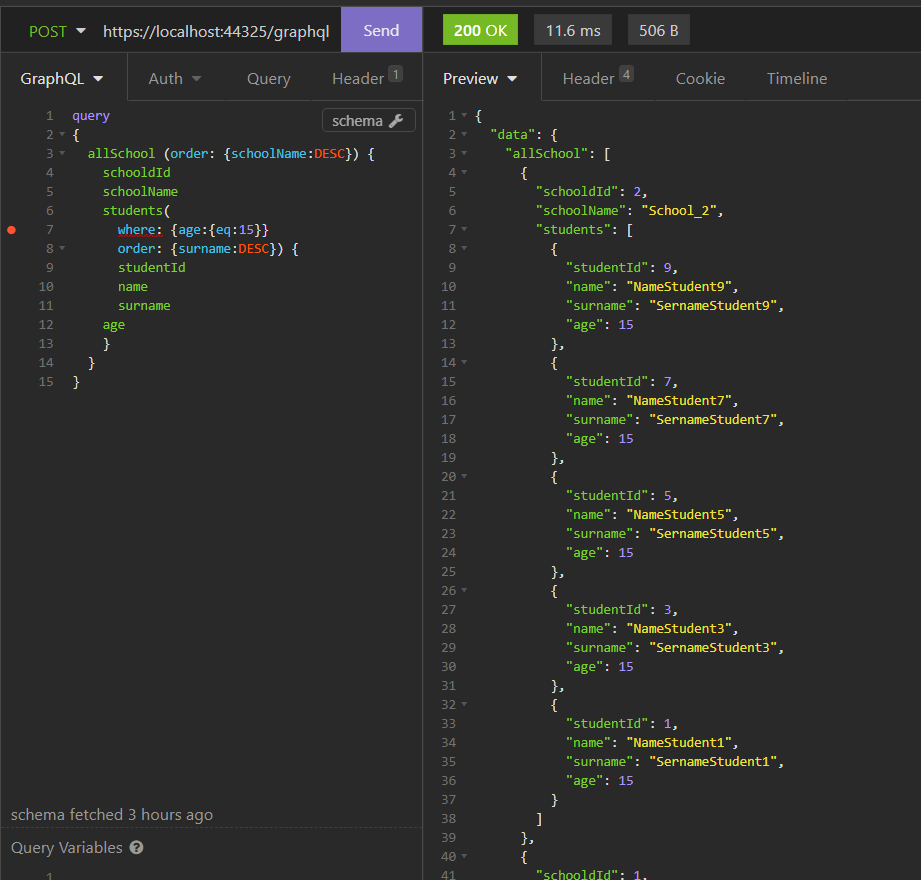
DOCUMENTATION
In order to add documentation about methods and entities, we can use the attribute [GraphQLDescription].
For example, if we want to add information about AllStudents method, we have to add this attribute in the Query class:
[QUERY.CS]
using HotChocolate;
using HotChocolate.Data;
using SchoolsService.DataAccess.Commands;
using SchoolsService.Model;
using System.Linq;
namespace SchoolsService.GraphQL
{
public class Query
{
[UseProjection]
[UseFiltering]
[UseSorting]
[GraphQLDescription("Method used to get list of all Students")]
public IQueryable<Student> AllStudents([Service] IStudentCore studentCore)
{
return studentCore.GetAllStudents();
}
[UseProjection]
[UseFiltering]
[UseSorting]
public IQueryable<School> AllSchool([Service] ISchoolCore schoolCore)
{
return schoolCore.GetAllSchools();
}
}
}
Instead, if we want to add information about School entity, we have to add the property in the School class:
[SCHOOL.CS]
using HotChocolate;
using HotChocolate.Data;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace SchoolsService.Model
{
[GraphQLDescription("School entity")]
public class School
{
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int SchooldId { get; set; }
public string SchoolName { get; set; }
[UseSorting]
[UseFiltering]
[GraphQLDescription("List of school's students")]
public ICollection<Student> Students { get; set; }
}
}
Now, if we run the application and we go to /graphql, this will be the result:
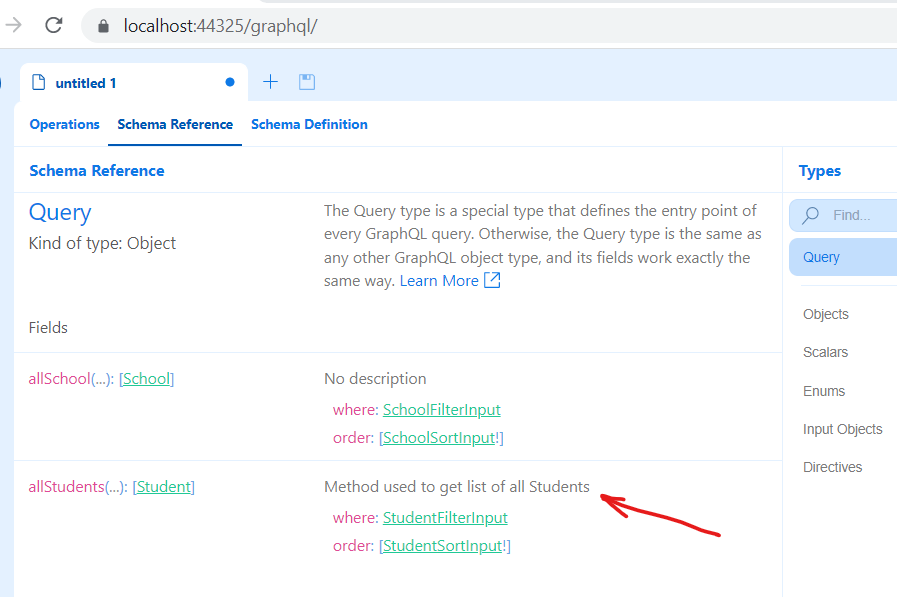
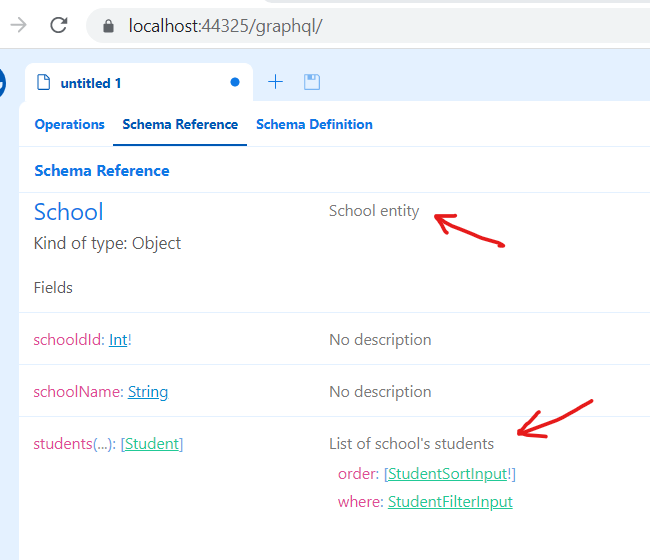
GRAPHQL – CODE
https://github.com/ZoneOfDevelopment/GraphQL