In this post, we will see how to ping an IP or Address with .net Core.
We start creating a Console application called CheckIP where, we will define a class called CheckService so defined:
[CHECKSERVICE.CS]
using System;
using System.Net.NetworkInformation;
namespace CheckIP
{
internal class CheckService
{
// A Tuple allows us to have more values in output
public (bool, string) IsReachable(string ipOrAddress)
{
// The using statement ensures the correct use of IDisposable objects.
using (var ping = new Ping())
{
try
{
bool result = true;
// In the method Send we can pass the IP or the Address
var checkPing = ping.Send(ipOrAddress);
if (checkPing.Status != IPStatus.Success)
{
result = false;
}
return (result, checkPing.Status.ToString());
}
catch(Exception ex)
{
return (false, ex.Message) ;
}
}
}
}
}
Finally, we modify Program.cs in order to use the class CheckService:
[PROGRAM.CS]
using System;
namespace CheckIP
{
internal class Program
{
static void Main(string[] args)
{
while(true)
{
Console.WriteLine("Check IP/Address");
Console.WriteLine("Insert the IP/Address to check: ");
var inputToCheck = Console.ReadLine().ToString();
CheckService objCheckService = new CheckService();
// We can define the variables names in the Tuple
(bool isOk, string message) checkResult = objCheckService.IsReachable(inputToCheck);
if (checkResult.isOk)
{
Console.WriteLine($"{inputToCheck} is reachable");
}
else
{
Console.WriteLine($"{inputToCheck} is not reachable for this reason: {checkResult.message}");
}
Console.WriteLine();
Console.WriteLine();
}
}
}
}
We have done and now, if we run the application, these will be the results:
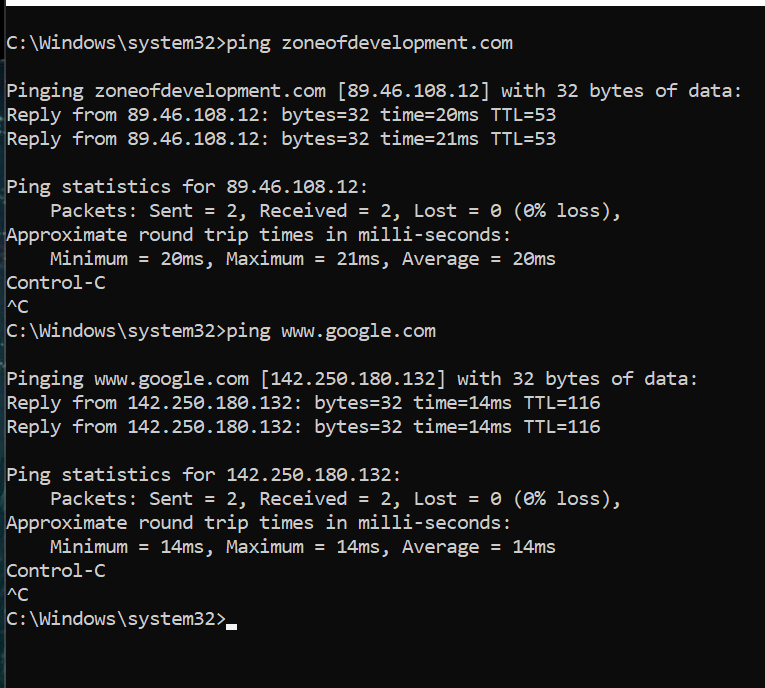
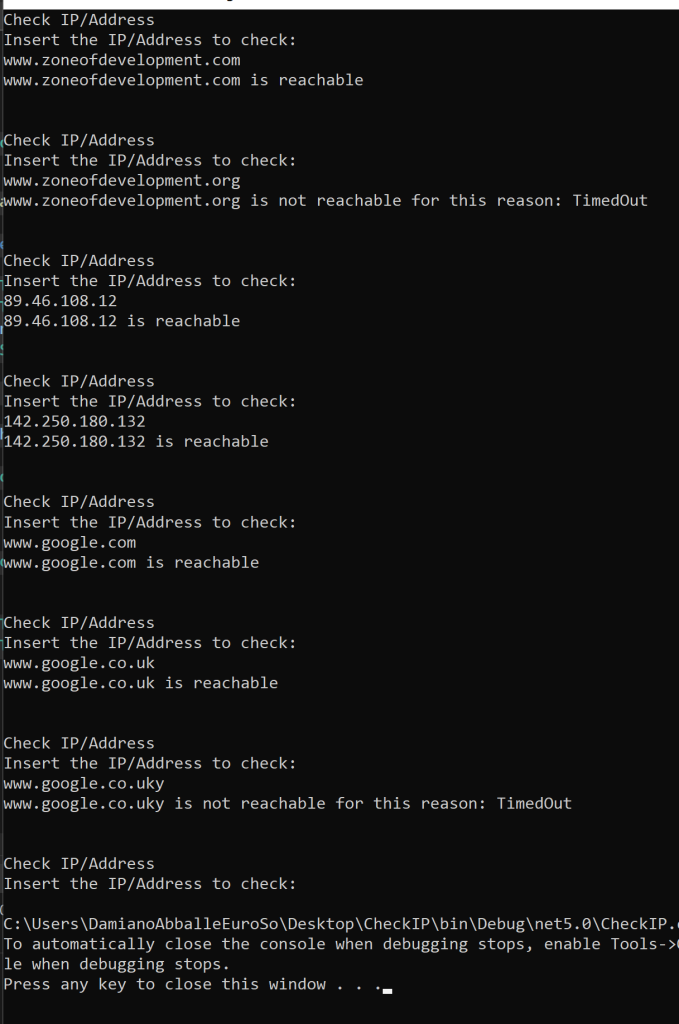