In this post, we will see how and when using Task.CompletedTask and Task.FromResult.
WHEN
We can use both of them when we need to return a Task object from a method that don’t have any async operations.
HOW
Task.CompletedTask
When we need to return a Task:
[CORE.CS]
namespace TestTaskCompleted
{
public class Core
{
public async Task PrintResult(int maxValue)
{
int result = 0;
for (int i = 1; i < maxValue; i++)
{
result = result + 10;
}
Console.WriteLine($"The result is {result}");
await Task.CompletedTask;
}
}
}
[PROGRAM.CS]
using TestTaskCompleted;
Core objCore = new Core();
await objCore.PrintResult(12);
If we run the application, this will be the result:
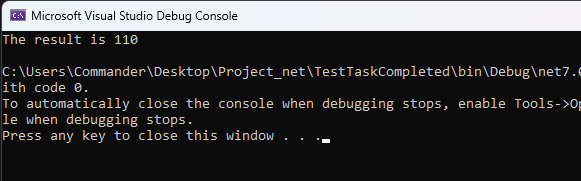
Task.FromResult
When we need to return a Task<T>:
[CORE.CS]
namespace TestTaskCompleted
{
public class Core
{
public async Task<int> SumAsync(int maxValue)
{
int result = 0;
for (int i = 1; i < maxValue; i++)
{
result = result + 10;
}
return await Task.FromResult<int>(result);
}
}
}
[PROGRAM.CS]
using TestTaskCompleted;
Core objCore = new Core();
var result = await objCore.SumAsync(12);
Console.WriteLine($"The result is: {result}");
If we run the application, this will be the result:
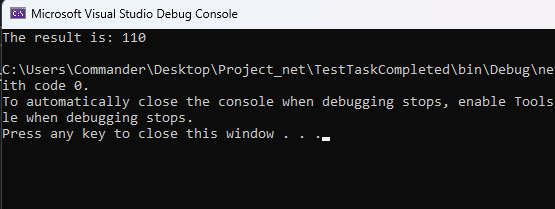