In this post, we will see how to read a Configuration file that we usually use in our projects.
As we know, a Configuration file is used to store key value pairs or some configurable information that we could need in our projects. A config file is very useful because, if we need to change a value, we should just to modify the file without touching our code.
It is possible to use many formats to define a Configuration file but, in this post, we will see maybe the most used format: INI.
We start creating a file called settings.ini where, we will insert some parameters for a generic DB and other generic parameters:
[GenericDB]
host = localhost
user = userDamiano
password = $Password123
db = DBTest
[ApplicationParameters]
environment = Production
ftp = test.testsite.com
user = UserFTP
password = $FTPPass1
Then, we create a file called main.py used to read the settings.ini file:
# importing the module used to read a config file
import configparser
# definition of the object used to read the file
objConfigFile = configparser.ConfigParser()
objConfigFile.read("settings.ini")
# definition of variables used to read the GenericDB section
objGenericDB = objConfigFile["GenericDB"]
host = objGenericDB["host"]
userDb = objGenericDB["user"]
passwordDb = objGenericDB["password"]
database = objGenericDB["db"]
# definition of variables used to read the ApplicationParameters section
objApplicationParameters = objConfigFile["ApplicationParameters"]
environment = objApplicationParameters["environment"]
ftp = objApplicationParameters["ftp"]
user = objApplicationParameters["user"]
password = objApplicationParameters["password"]
# printing the parameters
print("### GENERICDB ###")
print(f"host => {host}")
print(f"user => {userDb}")
print(f"password => {passwordDb}")
print(f"databse => {database}")
print()
print("### APPLICATIONPARAMETERS ###")
print(f"environment => {environment}")
print(f"ftp => {ftp}")
print(f"user => {user}")
print(f"password => {password}")
We have done and now, if we run the application, this will be the result:
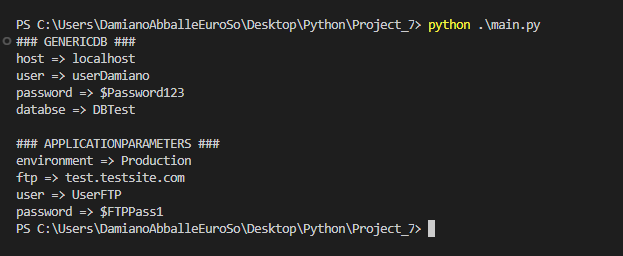