In this post, we will see how to use Code Snippets and how to create a custom one.
But first of all, what is a Code Snippet?
From Microsoft web site:
“Code snippets are ready-made snippets of code you can quickly insert into your code. For example, the for
code snippet creates an empty for
loop.
You can insert a code snippet at the cursor location, or insert a surround-with code snippet around the currently selected code”
In a nutshell, a Code Snippets is simple “command” that can help us to be more productive during our develops.
We start opening Visual Studio, we create a Console application and then we open the file Program.cs for testing some Code Snippets:
creating a new class: class + Tab twice
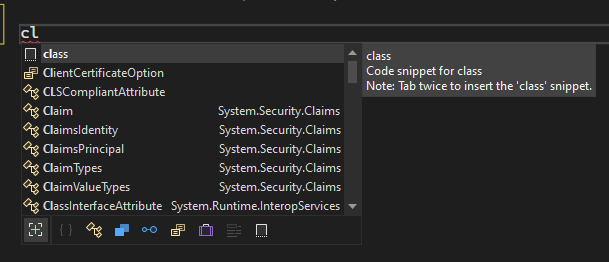
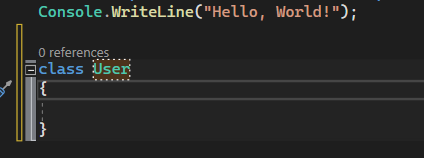
creating a constructor: ctor + Tab twice
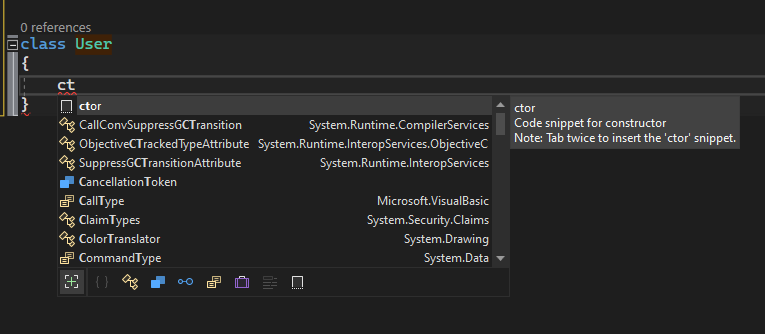
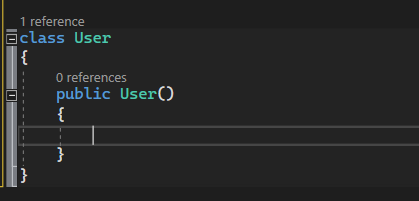
creating a public property: prop + Tab twice
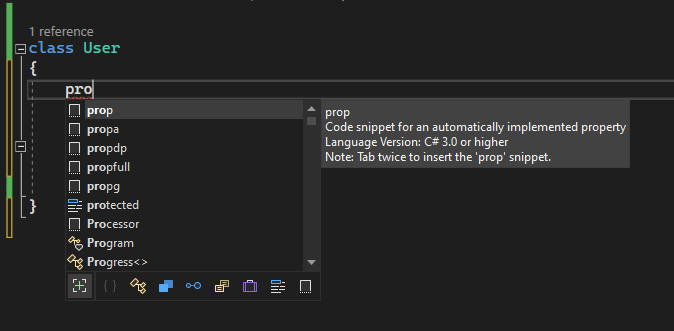
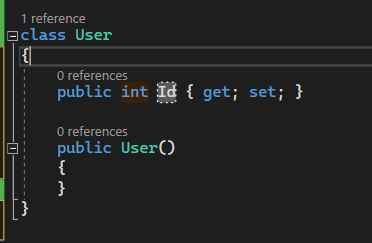
create a public/private property: propfull + Tab twice
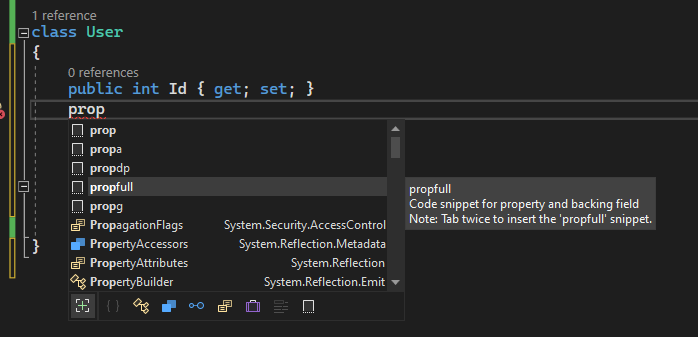
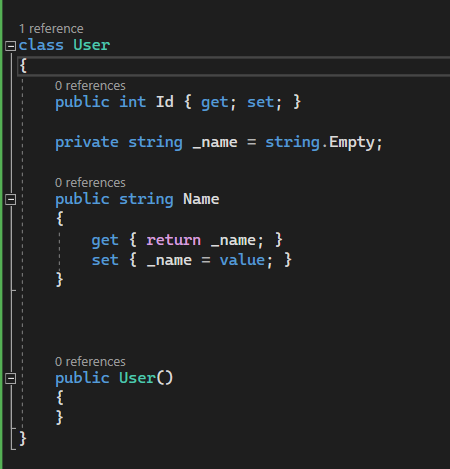
creating an interface: interface + Tab twice
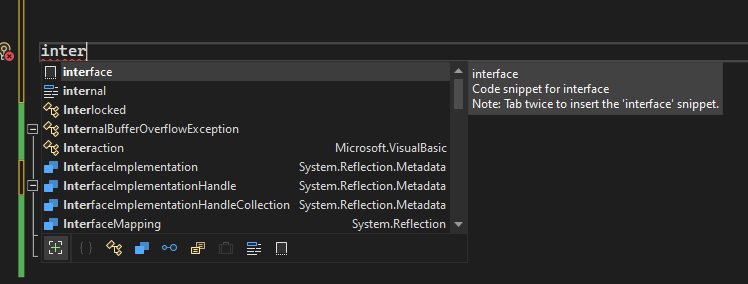
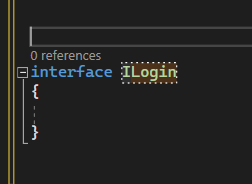
creating a for loop: for + Tab twice
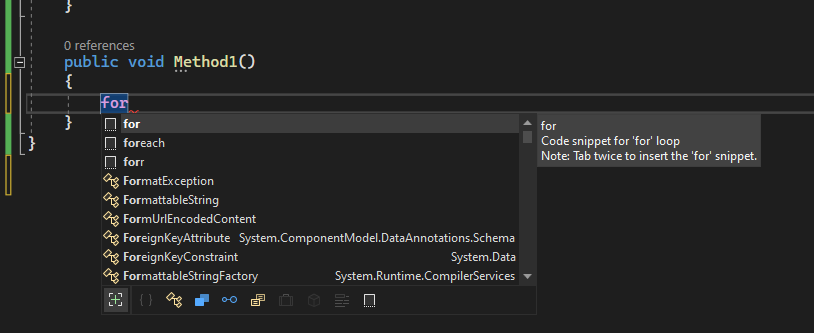
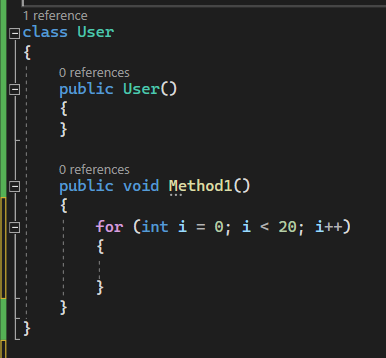
creating a foreach loop: foreach + Tab twice
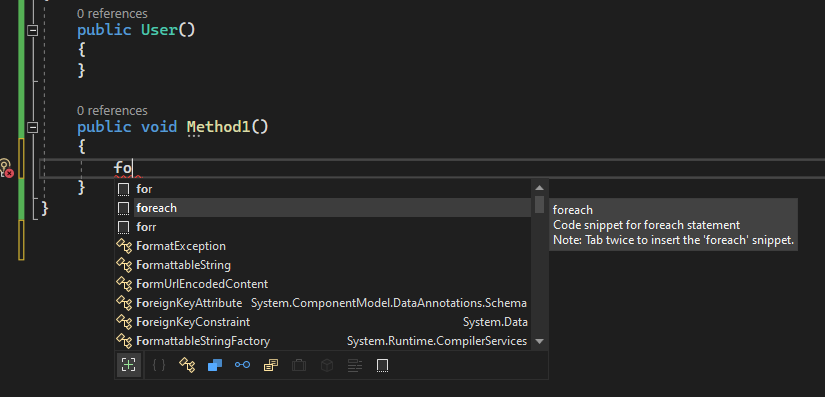
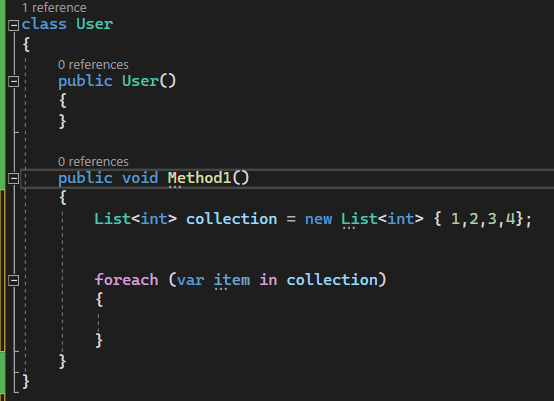
creating a switch block: switch + Tab twice
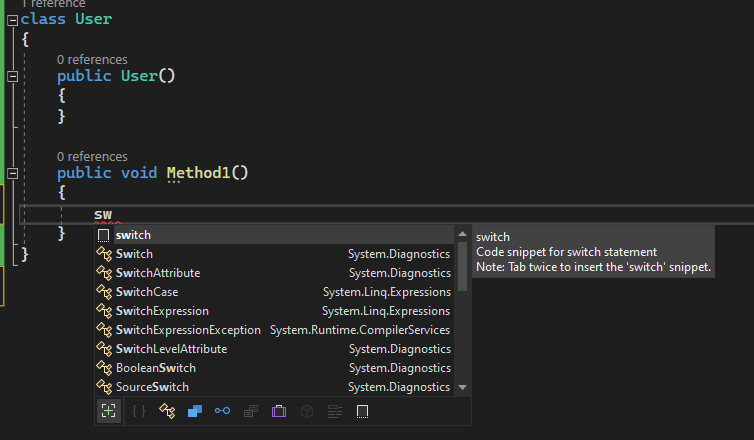
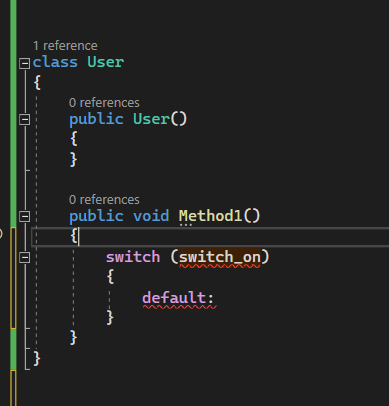
creating a try-catch block: try + Tab twice
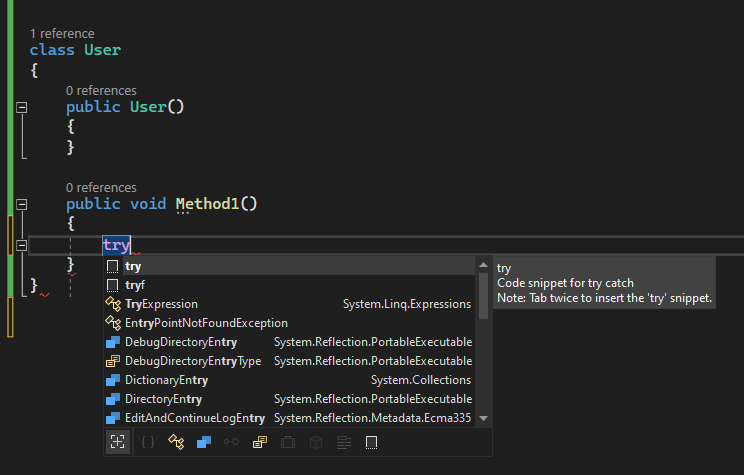
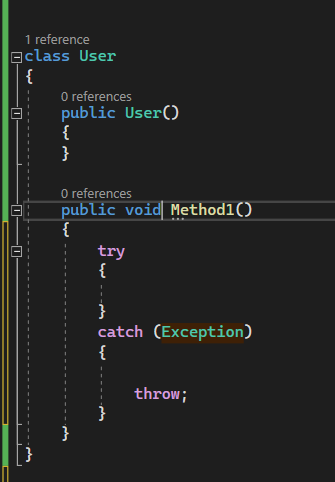
CREATING TWO CUSTOM CODE SNIPPETS
The first Code Snippet is used for creating a template of a class User.
We create a file xml called CompleteUserClass where we will insert this code:
[COMPLETEUSERCLASS.XML]
<?xml version="1.0" encoding="utf-8"?>
<CodeSnippets xmlns="http://schemas.microsoft.com/VisualStudio/2005/CodeSnippet">
<CodeSnippet Format="1.0.0">
<Header>
<Title>User Class</Title>
<Author>Damiano Abballe</Author>
<Description>Template for a User class</Description>
<Shortcut>user</Shortcut>
</Header>
<Snippet>
<Code Language="CSharp">
<![CDATA[
public class User
{
private Guid _id = Guid.Empty;
private string _username = string.Empty;
private string _password = string.Empty;
public User(string Username, string Password)
{
_username = Username;
_password = Password;
_id = Guid.NewGuid();
}
public Guid GetUserId()
{
return _id;
}
}
]]>
</Code>
</Snippet>
</CodeSnippet>
</CodeSnippets>
Then, we have to save the file with the snippet extension:
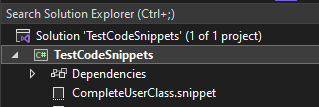
Now, we go to Tools/Code Snippets Manager:
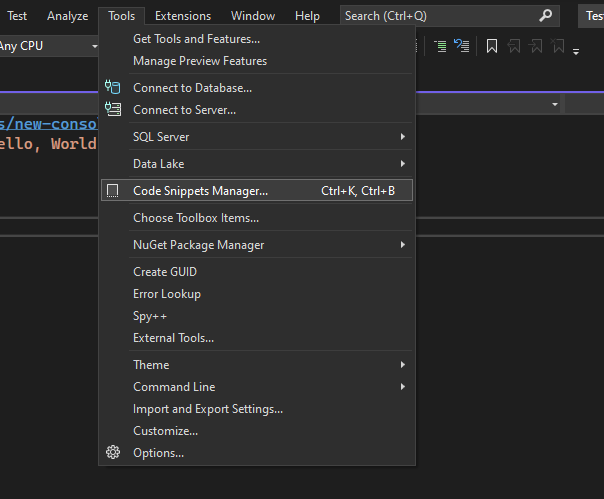
We select “Import”:
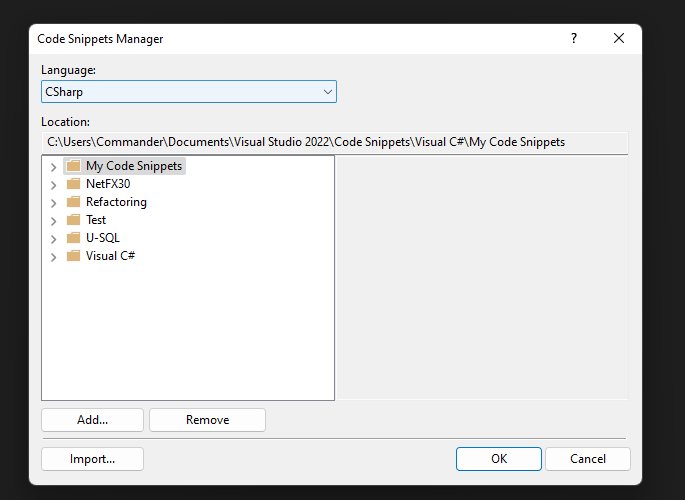
We select our snippet file:
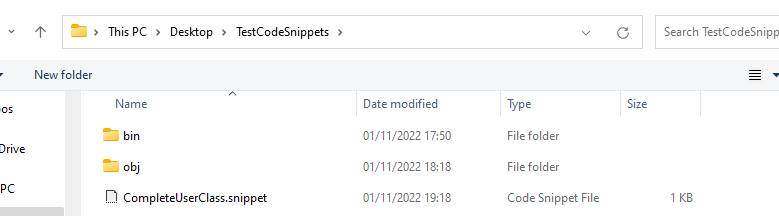
Finally, we click on “Finish”
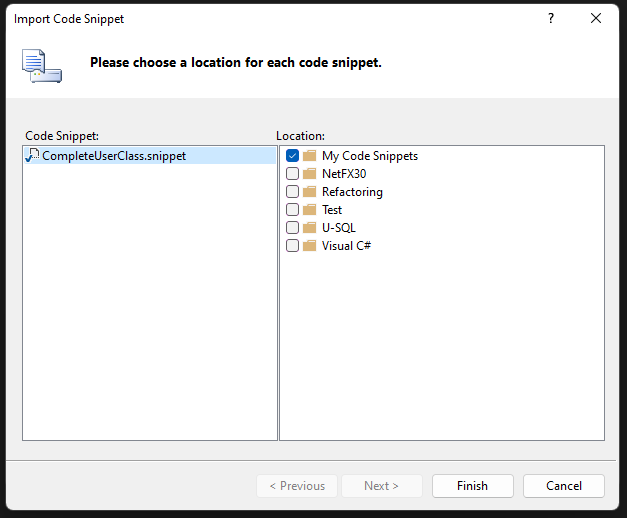
We have done and now, we can use this new Code Snippet with “user + Tab twice”:
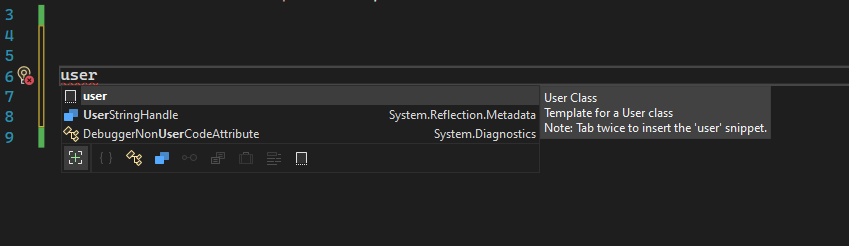
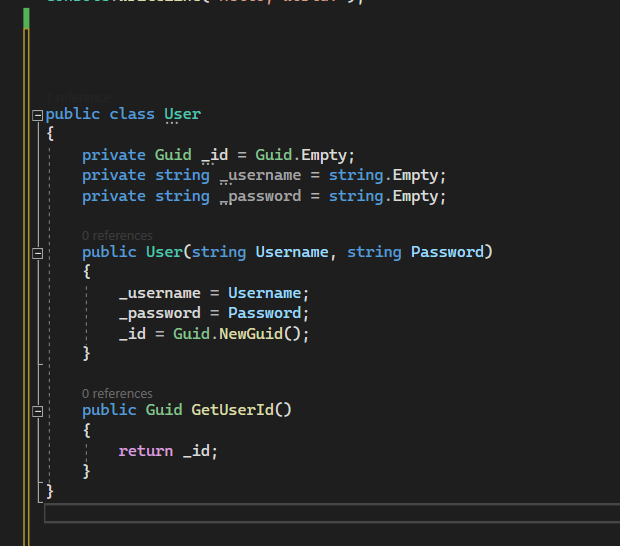
The second Code Snippet is used to calculate the square of a number.
We create a Code Snippet called Square.snippet, where we will insert this code:
[SQUARE.SNIPPET]
<?xml version="1.0" encoding="utf-8"?>
<CodeSnippets xmlns="http://schemas.microsoft.com/VisualStudio/2005/CodeSnippet">
<CodeSnippet Format="1.0.0">
<Header>
<Title>Square Operation</Title>
<Author>Damiano Abballe</Author>
<Description>Calculates the square of a number.</Description>
<Shortcut>square</Shortcut>
</Header>
<Snippet>
<Code Language="CSharp">
<![CDATA[double result= $value$ * $value$;]]>
</Code>
<Declarations>
<Literal>
<ID>value</ID>
<ToolTip>Choose the number you want the calcolate the square.</ToolTip>
<Default>1</Default>
</Literal>
</Declarations>
</Snippet>
</CodeSnippet>
</CodeSnippets>
Then, after we added it in the same way as above, we can use it with “square + Tab twice”:
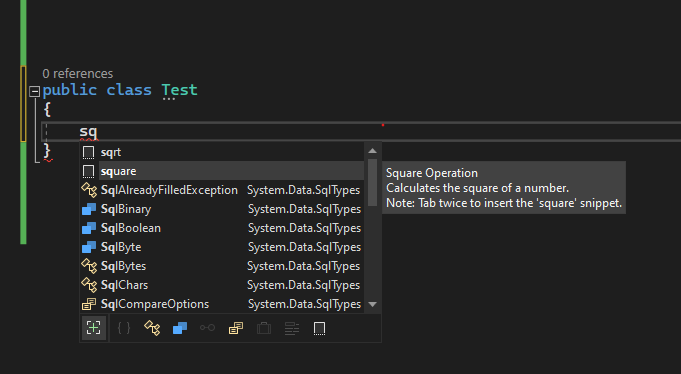
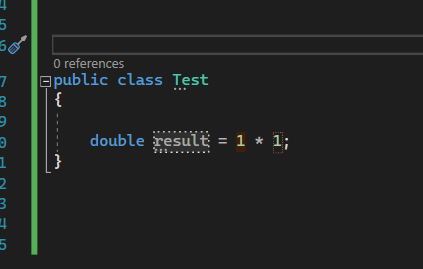