In this post, we will see how to read and write a text file with Python.
First of all, we create a Text file called testpython.txt and write inside these five rows:
id:1;username:username1:datestart:01/01/1990
id:2;username:username2:datestart:06/08/1994
id:3;username:username3:datestart:15/03/2000
id:4;username:username4:datestart:23/08/2007
id:5;username:username5:datestart:30/12/2018
READ A FILE
We create a file called readfile.py:
[READFILE.PY]
# class userItem
class userItem:
def __init__(self, id, username):
self.Id = id
self.Username = username
def PrintInfo(self):
print(f"{self.Id} -> {self.Username}")
# definition of a function to read a text file lines by lines
def ReadLineByLine():
# open file and read the contents
readFile = open("testpython.txt", "r")
# read all lines and put them in a list of string
lines = readFile.readlines()
lst = []
for item in lines:
# we take only the values of Id and Username
idValue = (((item.strip().split(";"))[0]).split(":"))[1]
usernameValue = (((item.strip().split(";"))[1]).split(":"))[1]
# we save the values into a list of userItem
objUserItem = userItem(idValue, usernameValue)
lst.append(objUserItem)
# close the file
readFile.close()
# return the list of userItem
return lst
lstRows = ReadLineByLine()
for item in lstRows:
item.PrintInfo()
If we run the file, using the command python3 readfile.py, this will be the result:
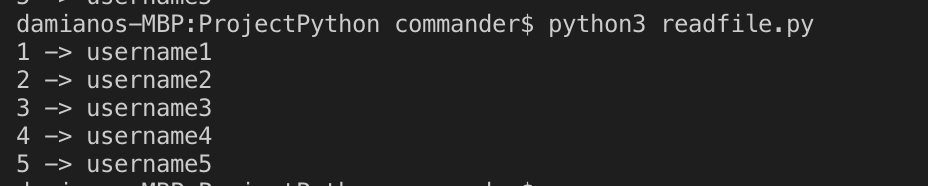
WRITE FILE
In order to write a file, we add a function called CreateFile that, it will create a Text file called newtestpython.txt.
def CreateFile(lstIem):
# Open file in append mode.
# If file does not exist, it will create a new file.
writefile = open("newtestpython.txt", "a")
for item in lstIem:
# we write the file line by line
writefile.writelines("Id:" + item.Id + ";Username:" + item.Username + "\n")
# close the file
writefile.close()
Finally, we have to call it at the end of readfile.py:
lstRows = ReadLineByLine()
for item in lstRows:
item.PrintInfo()
CreateFile(lstRows)
If we run the application, it will create the file newtestpython.txt:

If we try to run again, it will add the new rows at the end of newtestpython.txt:
