In this post, we will see how to call and use a web service with Python.
First of all, we need to install a library called “request“, in order to make a HTTP request from python script.
This library isn’t part of the standard Python library thus we need to install it, using the command pip3 install requests.
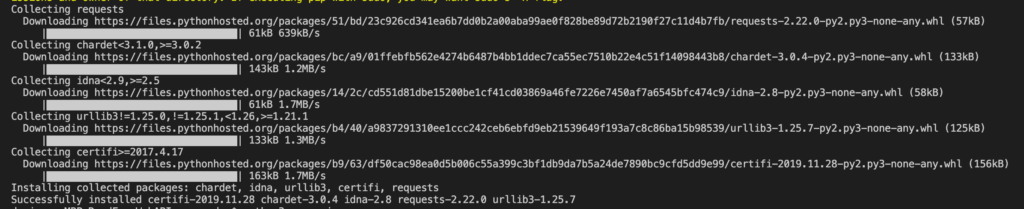
Now, we create two files called clsUpper.py and callWebApi.py:
[CLSUPPER.PY]
class User:
def __init__(self, id, userName, typeUserName, createDate):
self.Id = id
self.UserName = userName
self.TypeUserName = typeUserName
self.CreateDate = createDate
[CALLWEBAPI.PY]
# import the class User
from clsUser import User
# import the library requests
import requests
# put in a variable the url of the Web API
URLWebAPI = "https://firstwebapi001.azurewebsites.net/api/user"
def GetListUsers():
# call the WebApi
objListUser = requests.get(URLWebAPI)
# verify the request code
if (objListUser.status_code == 200):
# with json(), we will have the json-encoded of a response
print(objListUser.json())
else:
print(f"Attention! We received the error {objListUser.status_code} form the Web API")
# call the method used to get data from Web API service
GetListUsers()
If we run the script, with the command python3 callWebApi.py, this will be the result:

It works fine.
Now, we will change our Python code, in order to save the Web API’s result in a list of User:
# import json
import json
# import the class User
from clsUser import User
# import the library requests
import requests
# put in a variable the url of the Web API
URLWebAPI = "https://firstwebapi001.azurewebsites.net/api/user"
def GetListUsers():
# call the WebApi
callWebAPI = requests.get(URLWebAPI)
# define an empty list
lstUser = []
# verify the request code
if (callWebAPI.status_code == 200):
# with json(), we will have the json-encoded of a response
objListUsers = callWebAPI.json()
for itemService in objListUsers:
# create an User object
objUser = User(itemService["id"], itemService["username"], itemService["typeUserName"], itemService["createDate"])
# put the object into the list
lstUser.append(objUser)
else:
print(f"Attention! We received the error {callWebAPI.status_code} from the Web API")
return lstUser;
# call the method used to get data from Web API service
lstUserForAPI = GetListUsers()
for item in lstUserForAPI:
print(f"ID:{item.Id} - UserName:{item.UserName} - TypeUser:{item.TypeUserName} - Created:{item.CreateDate}")
Now, if we run the script, this will be the result:
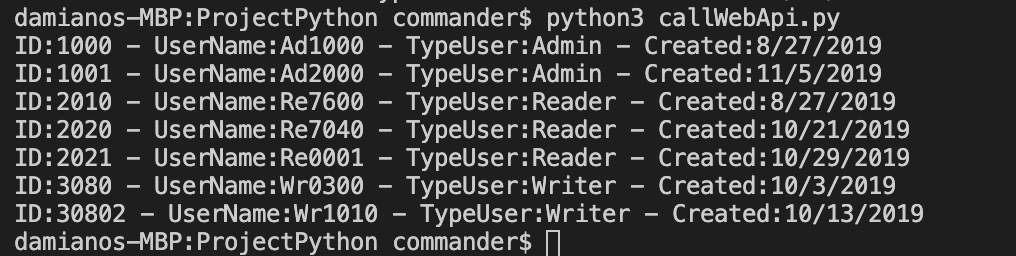