In this post, we will see how to add Angular Material in an Angular project.
We open Visual Studio Code and we create a new project called MaterialProject, using the command
ng new MaterialProject
Then, we create three components called HomePage, Page1 and Page2, using the commands
ng g c HomePage
ng g c Page1
ng g c Page2
Finally, we install Angular Material, using the command
ng add @angular/material
Now, we open the project and first of all, we add the three components in the Routing:
[APP-ROUTING.MODULE.TS]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | import { NgModule } from '@angular/core' ; import { Routes, RouterModule } from '@angular/router' ; import { Page1Component } from './page1/page1.component' ; import { Page2Component } from './page2/page2.component' ; import { HomePageComponent } from './home-page/home-page.component' ; const routes: Routes = [ { path: '' , pathMatch: 'full' , redirectTo: 'homepage' }, { path: 'homepage' , component: HomePageComponent }, { path: 'page1' , component: Page1Component }, { path: 'page2' , component: Page2Component }, { path: '**' , pathMatch: 'full' , redirectTo: 'homepage' } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } |
Then, we insert a simple menu:
[APP.COMPONENT.HTML]
<h1>
Angular Material
</h1>
<button routerLink="/">Home</button>
<button routerLink="/page1">Page1</button>
<button routerLink="/page2">Page2</button>
<router-outlet></router-outlet>
If we run the application, using the command ng serve, this will be the result:
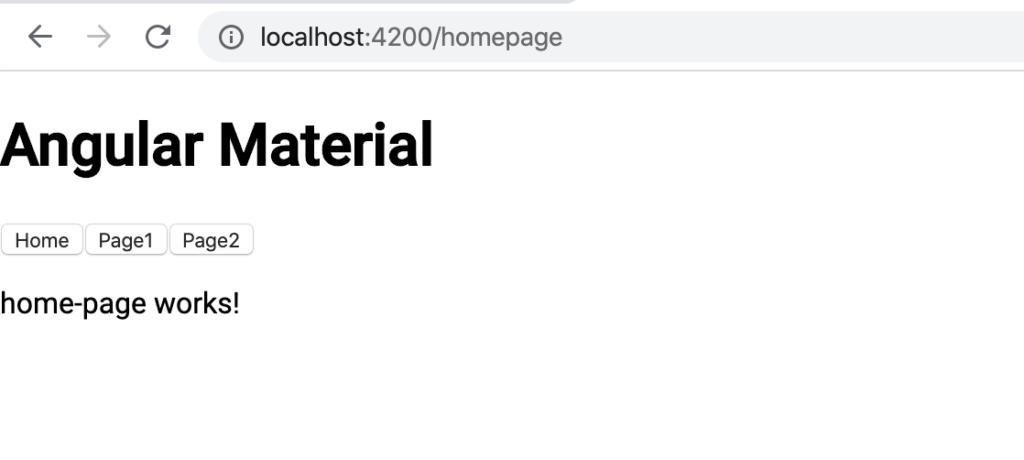
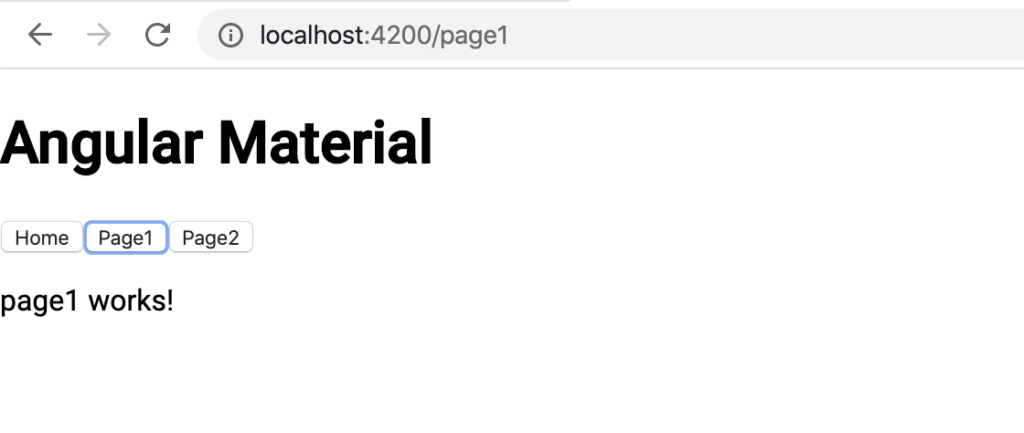
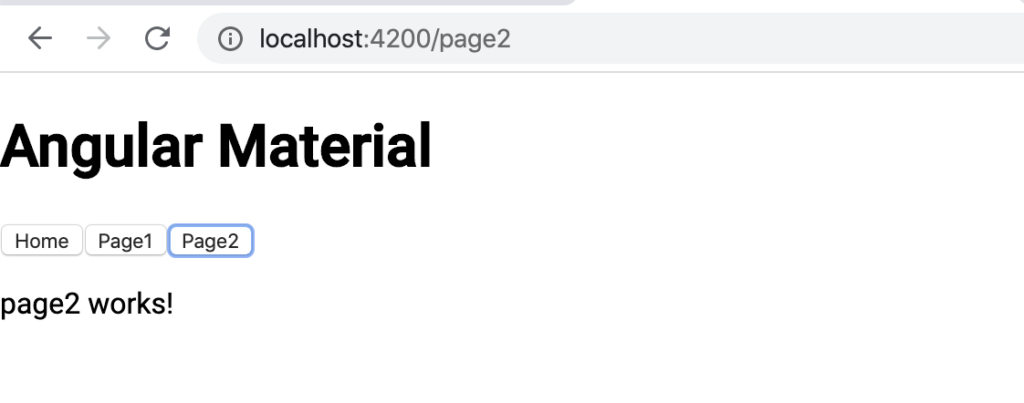
Everything works fine and now, with a few steps, we will add Angular Material in the project.
First of all, we modify the file app.module:
[APP.MODULE.TS]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | import { BrowserModule } from '@angular/platform-browser' ; import { NgModule } from '@angular/core' ; import { AppRoutingModule } from './app-routing.module' ; import { AppComponent } from './app.component' ; import { Page1Component } from './page1/page1.component' ; import { Page2Component } from './page2/page2.component' ; import { BrowserAnimationsModule } from '@angular/platform-browser/animations' ; import { HomePageComponent } from './home-page/home-page.component' ; import { MatToolbarModule } from '@angular/material/toolbar' ; import { MatIconModule } from '@angular/material/icon' ; import { MatCardModule } from '@angular/material/card' ; import { MatButtonModule } from '@angular/material/button' ; @NgModule({ declarations: [ AppComponent, Page1Component, Page2Component, HomePageComponent ], imports: [ BrowserModule, AppRoutingModule, BrowserAnimationsModule, MatToolbarModule, MatIconModule, MatCardModule, MatButtonModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } |
and finally, we modify the menu created before:
[APP.COMPONENT.HTML]
<mat-toolbar color="primary">
<h1>
Angular Material
</h1>
<button mat-button routerLink="/">Home</button>
<button mat-button routerLink="/page1">Page1</button>
<button mat-button routerLink="/page2">Page2</button>
</mat-toolbar>
<router-outlet></router-outlet>
We have done and, if we refresh the application, this will be the result:
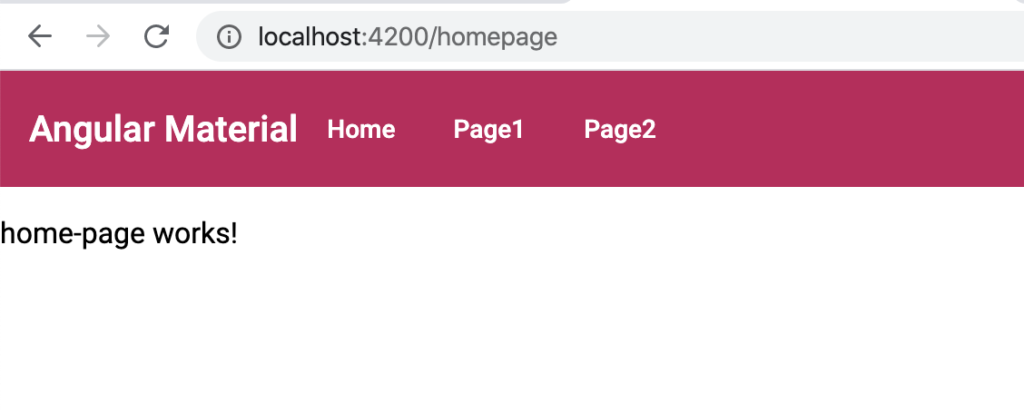
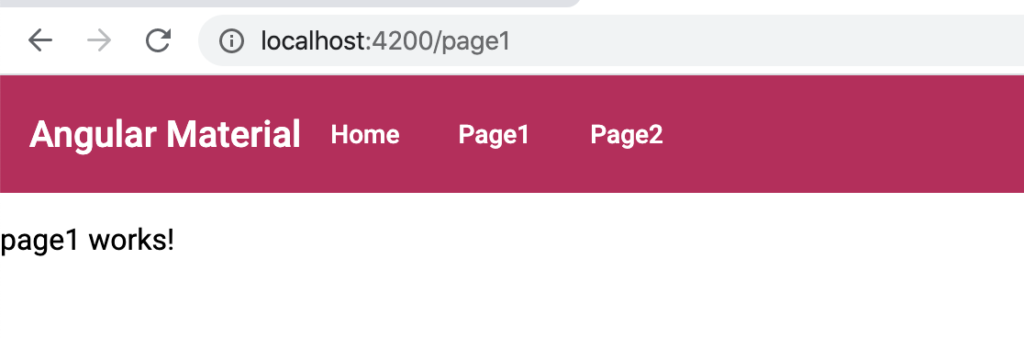
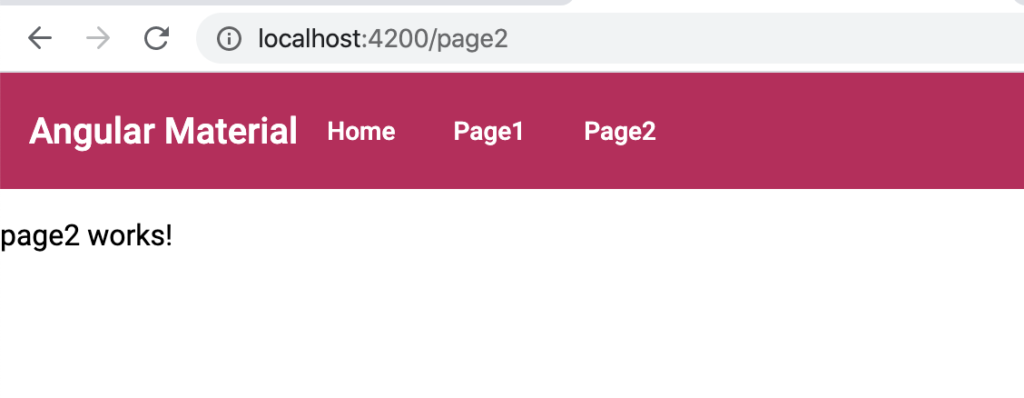
Like!! Thank you for publishing this awesome article.
Everything is very open with a clear description of the issues. It was truly informative.
I couldn’t resist commenting. Perfectly written