In this post, we will see how to manage Array and Dictionary in Swift.
ARRAY
import UIKit
// [Create Array]
var objArray = [1,2,3,4]
var objArray2 = [String]()
// [Add items]
objArray.append(5)
objArray2.append("one")
objArray2.append("two")
objArray2.append(contentsOf: ["three","five"])
objArray2.append("six")
// [Add in a specific position]
objArray.insert(0, at: 0)
objArray2.insert("four", at: 3)
// [Update a value]
objArray[1] = 11
print(objArray)
objArray2[0] = "zero"
print(objArray2)
// [Delete a value]
objArray.remove(at: 0)
print(objArray)
objArray2.remove(at: (objArray2.count-1))
print(objArray2)
// [Delete all]
objArray.removeAll()
objArray2 = []
If we run this code in Playground, this will be the result:
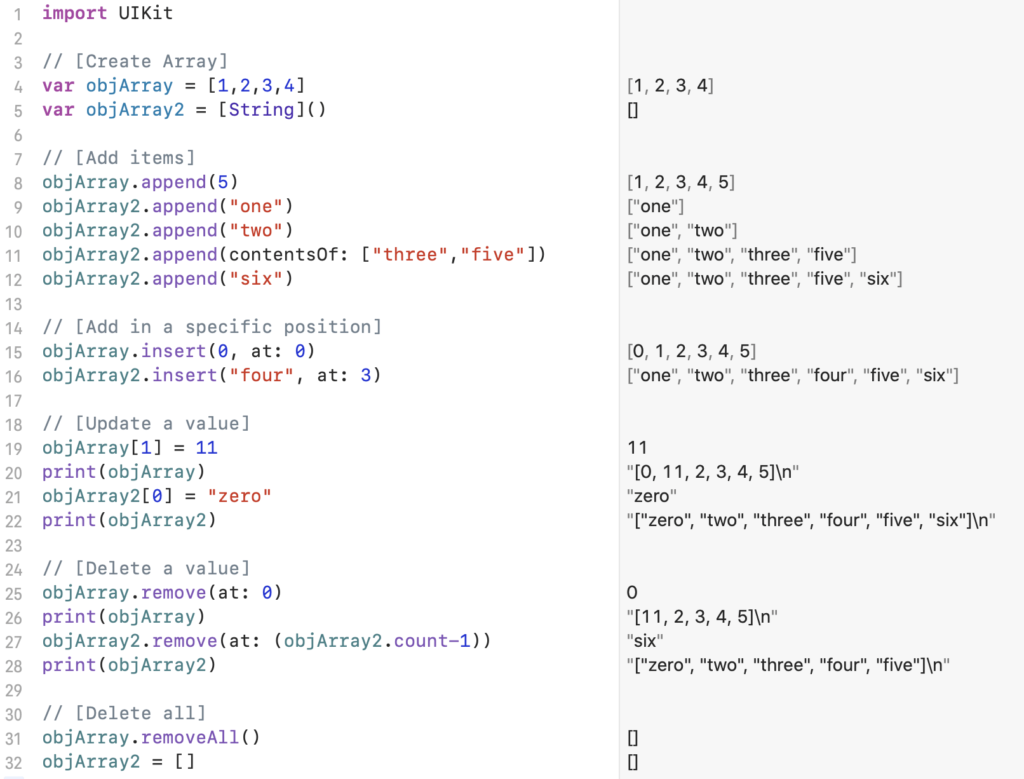
DICTIONARY
// [Create Dictionary]
var objDictionary = [1: "one", 2: "two", 3: "three"]
var objDictionary2 = [Int: String] ()
// [Add items]
objDictionary[4] = "four"
objDictionary2[10] = "ten"
objDictionary2[11] = "eleven"
print(objDictionary)
print(objDictionary2)
// [Update a value]
objDictionary[1] = "one1"
objDictionary2[10] = "ten10"
print(objDictionary)
print(objDictionary2)
// [Read]
print(objDictionary[3] ?? 0)
var objKey1 = [Int]()
for key in objDictionary.keys{
objKey1.append(key)
}
print(objKey1)
var objValue1 = [String]()
for value in objDictionary.values{
objValue1.append(value)
}
print(objValue1)
// [Delete an item]
objDictionary.removeValue(forKey: 4)
// [Delete all]
objDictionary.removeAll()
print(objDictionary)
print(objDictionary2)
If we run this code in Playground, this will be the result:
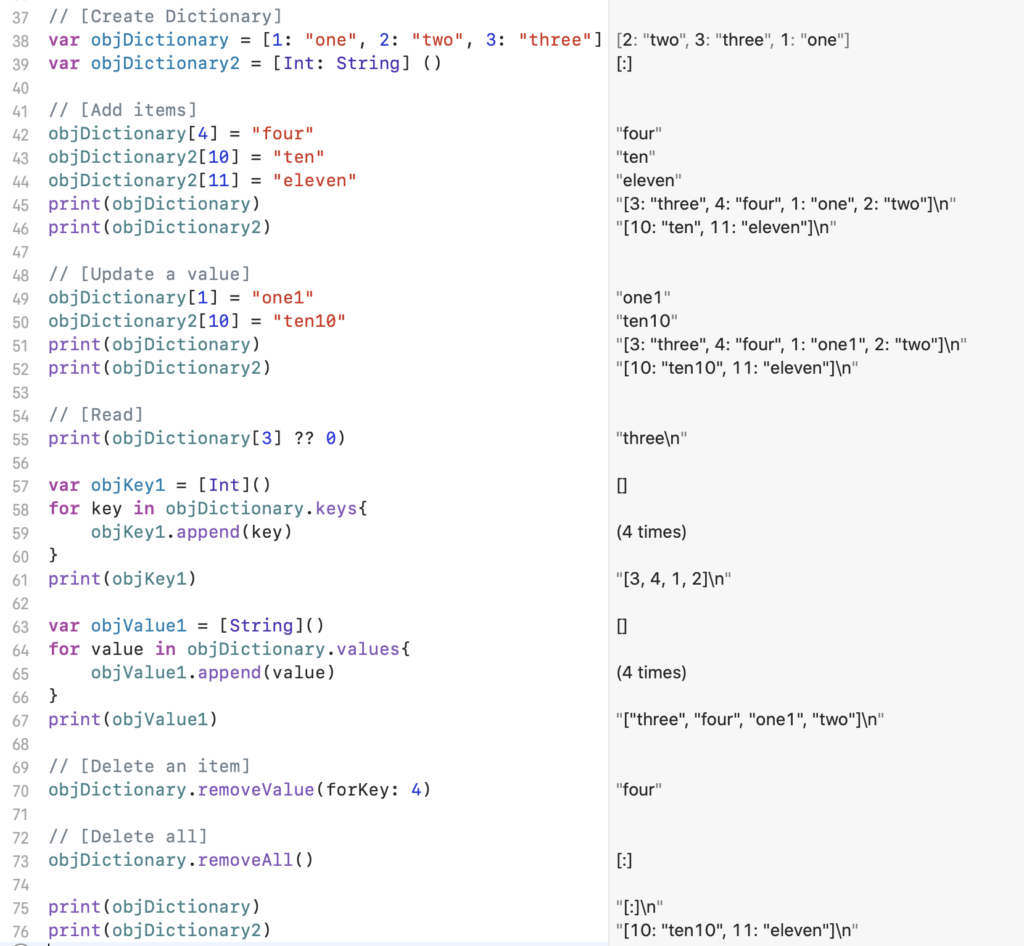