In this post, we will see how to define a Class from scratch and how to inherit from an existing in Python.
DEFINE A CLASS
Define a Class called Person:
class Person:
# definition of a Constructor where the last value is optional
def __init__(self, inputA, inputB, inputC=10):
self.A = inputA
self.B = inputB
self.C = inputC
# definition of a method called PrintInput
def PrintInput(self):
print(f"{self.A} - {self.B}")
# definition of a method called
def AddValue(self, C):
result = self.A + self.B + C
print(f"The value of the total sum is: {result}")
Now, we create a Python script, where we will use the class Person:
[TEST2.PT]
# we import the class Person from the file Person.py
from Person import Person
print("Insert two values")
value = [int(i) for i in input().split()]
objUser = Person(value[0], value[1])
objUser.PrintInput()
objUser.AddValue(30)
If we run the program, this will be the result:

INHERIT A CLASS FROM AN EXISTING
We define a Base Class called Dog:
class Dog:
def __init__(self, inputcolor, inputyears):
self.color = inputcolor
self.years = inputyears
def printInfo(self):
print(self.color, self.years)
def printInfo2(self):
print(f"{self.color}/{self.years}")
Now, we define a class Chihuahua inheriting from class Dog:
from Dog import Dog
# define a class called Chihuahua
# injection of Base class Dog
class Chihuahua(Dog):
breeder="TinyOro" # CONSTANT
def __init__(self,inputcolor, inputyears, inputname):
self.name = inputname
Dog.__init__(self, inputcolor, inputyears) # Base class Constructor
def printDogInfo(self):
print(self.name, self.color, self.years)
# Overriding method printInfo
def printInfo(self):
print("Override")
# Overloading method printInfo2
def printInfo2(self, val):
print("OVERLOADING", val)
Finally, we create a Python script, where we will use the class Chihuahua:
[TEST3.PY]
from Chihuahua import Chihuahua
objDog = Chihuahua('black',8,'Dorian')
objDog.printInfo()
objDog.printDogInfo()
print(objDog.breeder)
objDog.printInfo2('Test')
If we run the program, this will be the result:
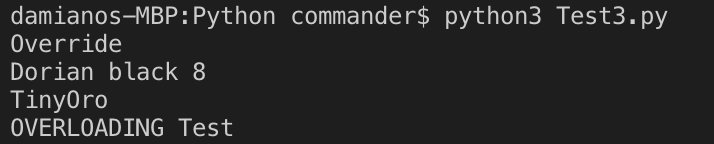