In this post, we will see how to implement an Alert in SwiftUI.
We create a project called AlertTest and add this code in the file ContentView:
[CONTENTVIEW.SWIFT]
import SwiftUI
struct ContentView: View {
// definition of variables used to manage Alert and show the date
@State private var showAlert = false
@State private var today = ""
// function used to get the current date
func ShowDate() {
// get the current date and time
let currentDateTime = Date()
// initialize the date formatter and set the style
let formatter = DateFormatter()
formatter.timeStyle = .medium
formatter.dateStyle = .long
// get the date time String from the date object
today = formatter.string(from: currentDateTime)
}
// function used to change the value of showAlert variable
func ShowWindow() {
self.showAlert = true
}
var body: some View {
ZStack {
// Definition background color
Color.green.edgesIgnoringSafeArea(.all)
VStack{
Spacer()
// button definition
Button(action: ShowWindow) {
Text("ShowWindow")
}
.frame(width: 200, height: 30)
.background(Color.blue)
.foregroundColor(Color.white)
.cornerRadius(35)
// Text used to show the date
Text(self.today)
.padding()
Spacer()
// Alert definition
// the "Yes" button calls the function ShowDate()
.alert(isPresented: $showAlert) {
Alert(title: Text("Title Window"), message: Text("Do you want to show the current date?"), primaryButton: .default(Text("Yes"), action: { self.ShowDate() }), secondaryButton: .cancel(Text("No")))
}
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
This is all and now, if we run the application, this will be the result:
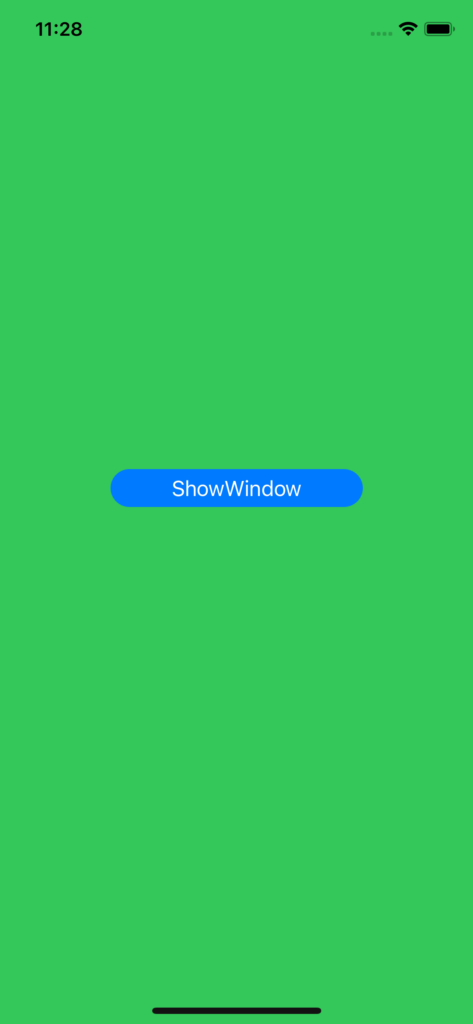
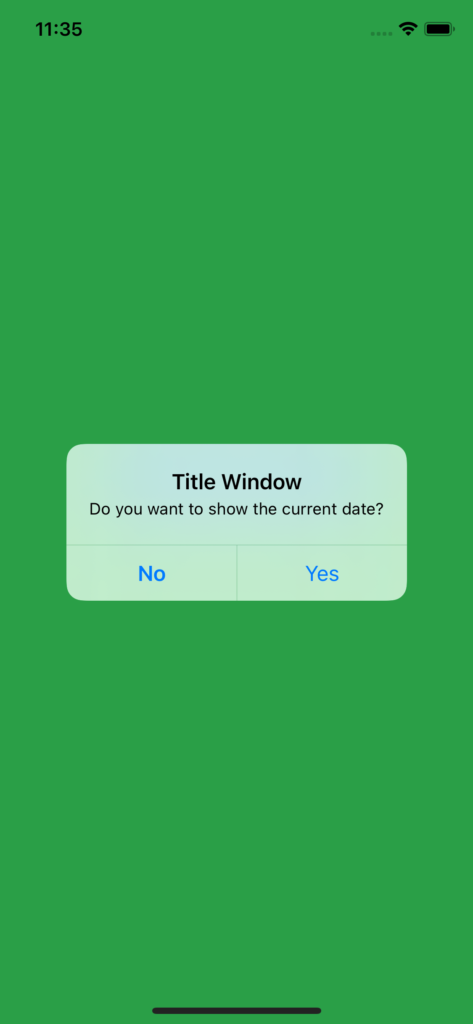
