In this post, we well see how to use Azure Key Vault in a console application, in order to store and retrieve a connection string.
First of all, what is Azure Key Vault?
From Microsoft web site:
“Azure Key Vault is a cloud service that provides a secure store for secrets. You can securely store keys, passwords, certificates, and other secrets. Azure key vaults may be created and managed through the Azure portal. In this quickstart, you create a key vault, then use it to store a secret.”
In a nutshell with Azure Key Vault we have a secure place where we can store our passwords, keys and so on.
We start going to Azure portal where we will create an App registration, in order to be able to manage an Azure Key Vault in our application:
Go to Azure portal:
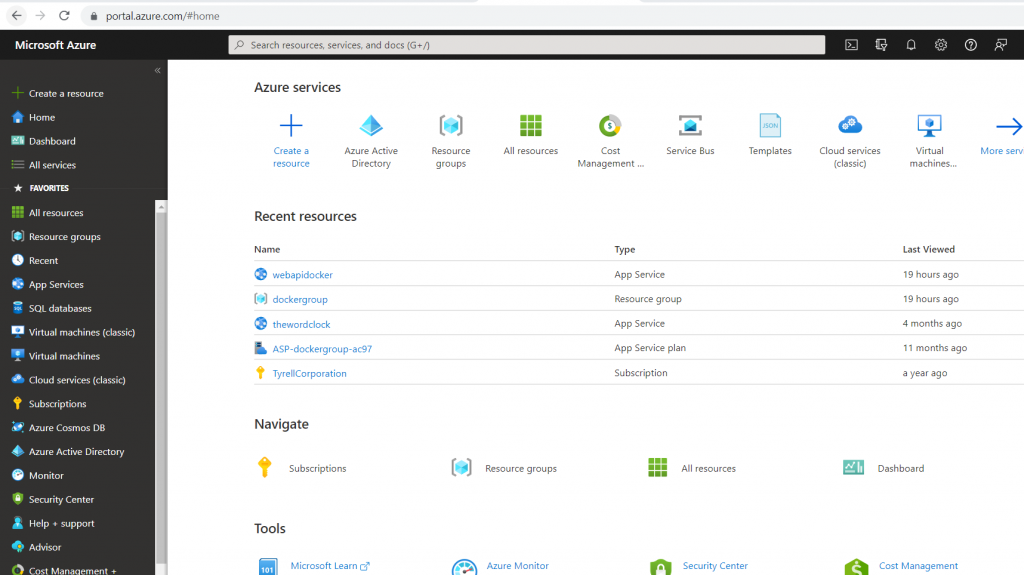
Select Azure Active Directory:

Select App registrations:

Select New registration:

Insert a Name and then press the button “Register”:

Save the value of Application ID and then go to “Certificates & secrets”:
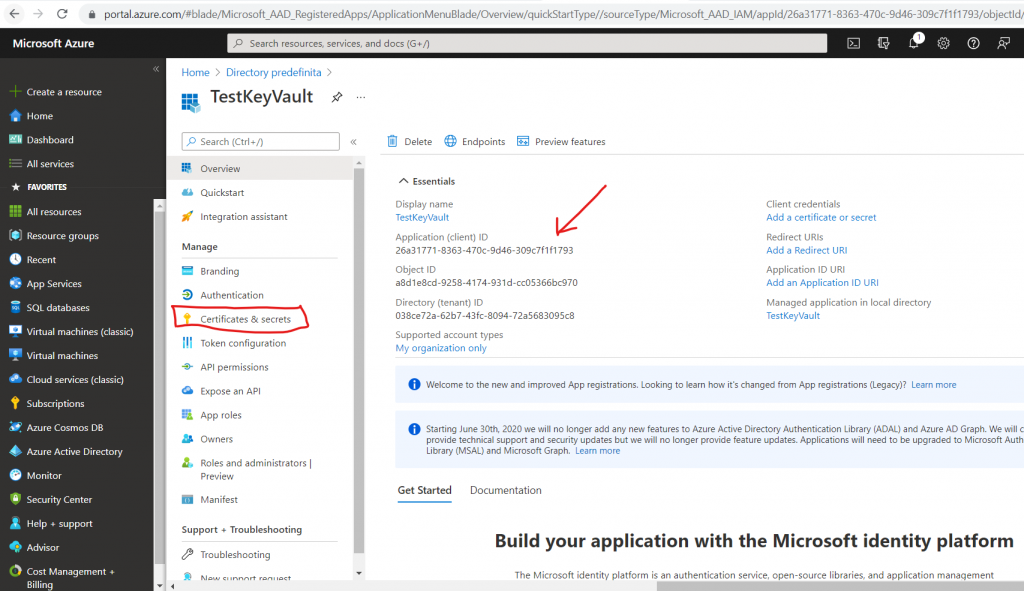
Add a “New client secret”:
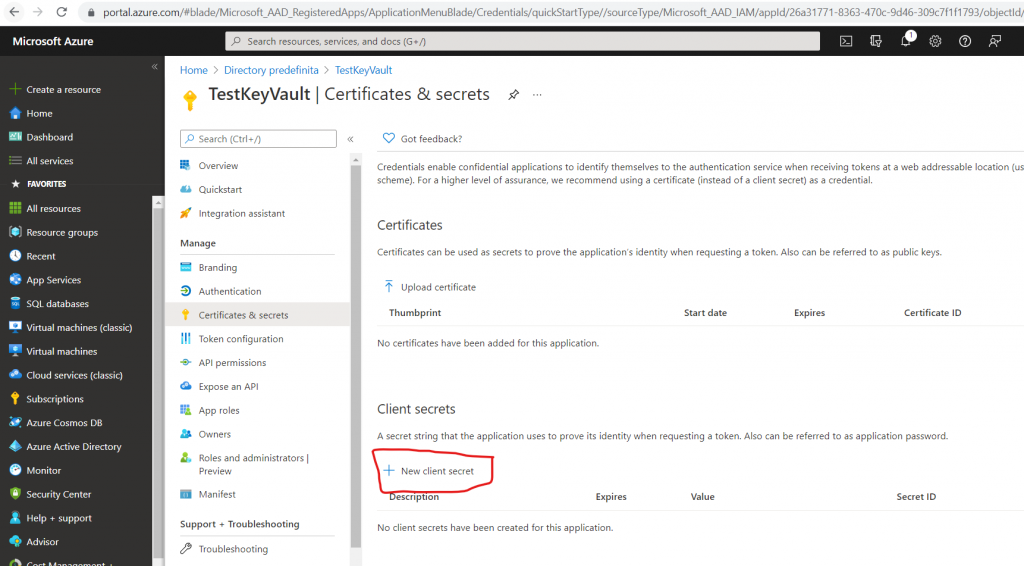
Insert a Description and the date of expiry:
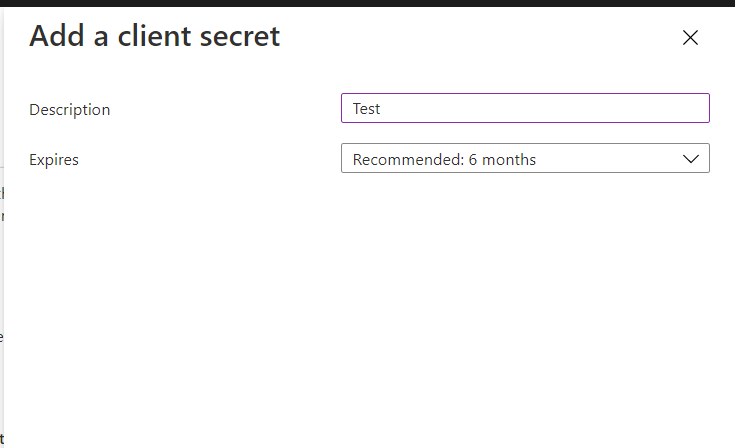
Finally, save the value of “Client secret” created right now:
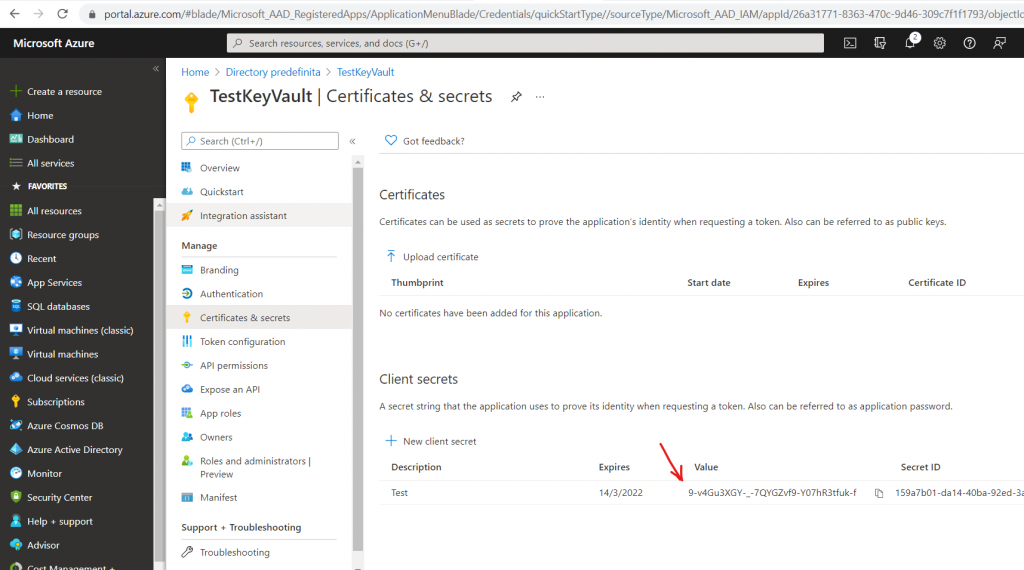
Now, we have to create an Azure Key Vault where we will save our “connection string”:
Go to Azure portal, click on “Create a resource” and search for “Key Vault”:

Click on Create:
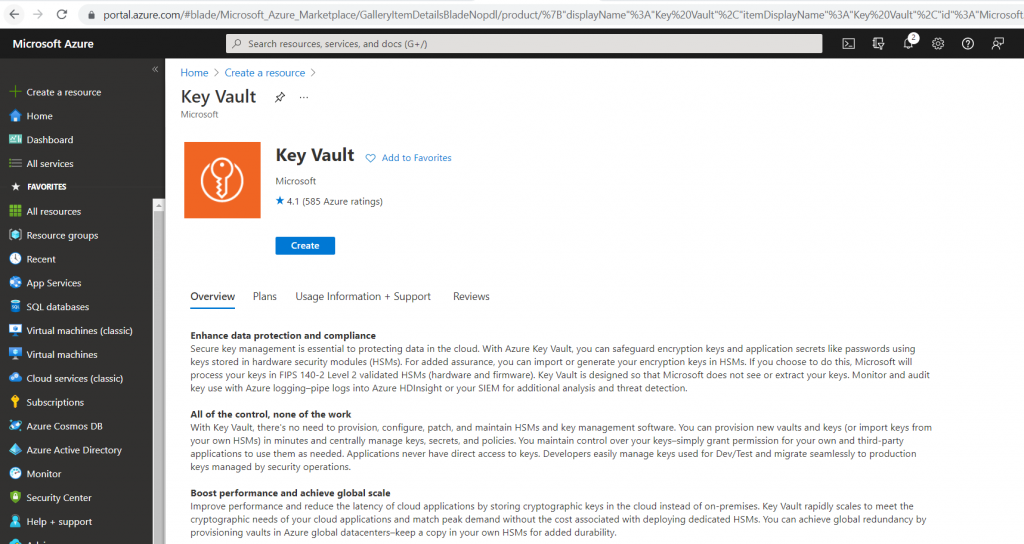
Insert all parameters and then click on Review + create:

Finally, we click on Create:

Now, we save the values of “Vault Uri” and “Directory ID” and then click on “Secrets”:

Click on Generate/Import:

Insert Name/Value (in this case “Server=myServerName\myInstanceName;Database=myDataBase;User Id=myUsername;Password=myPassword;”) and then click on Create:
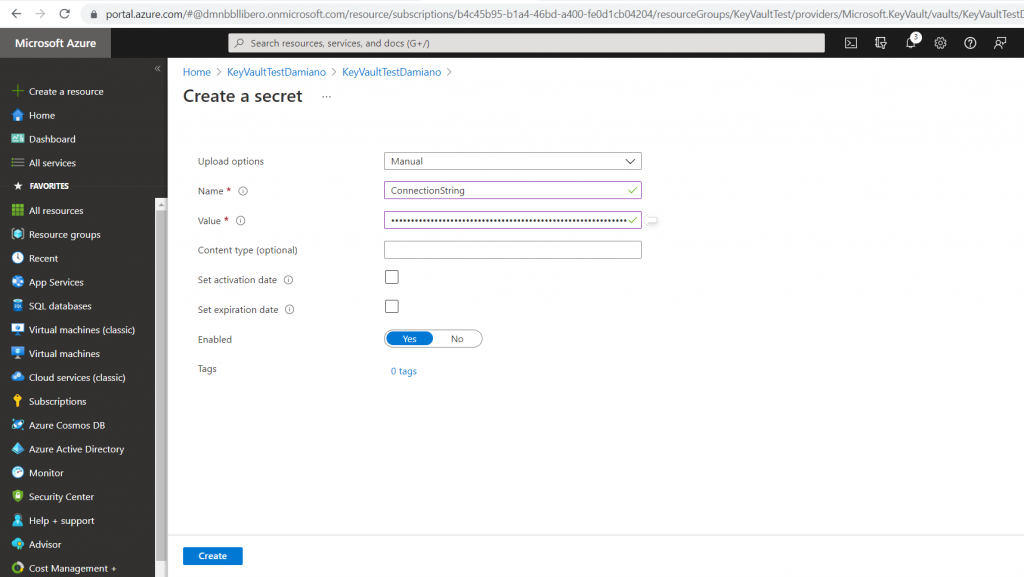
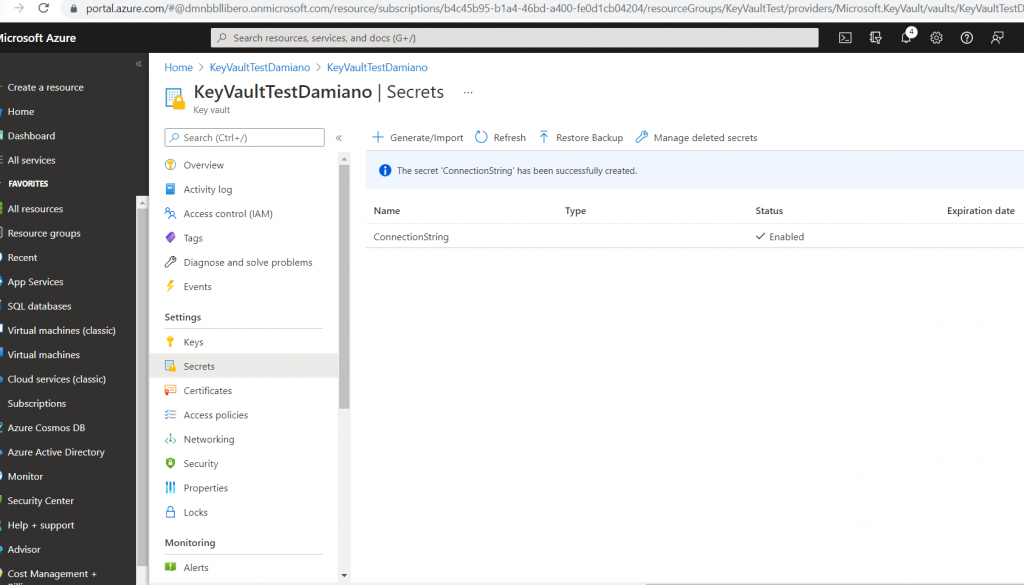
Now, we click on Access Policies in order to allow the Application created above to manage this Azure Key Vault:
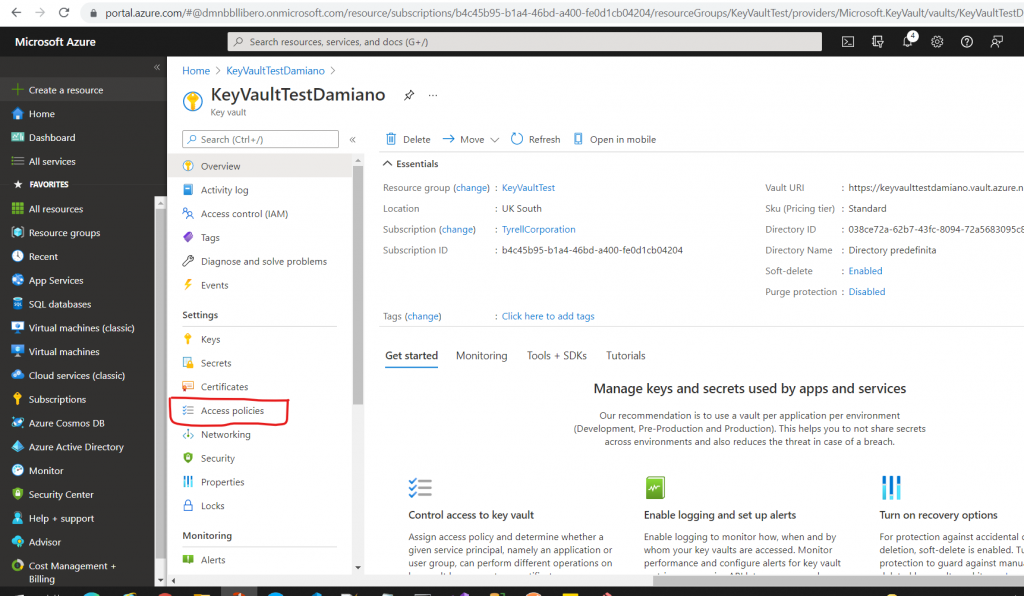
Click on Add Access Policy:

Here, we have to insert parameters, select the App create above and then we click on Select (for the App) and finally we click on Add:
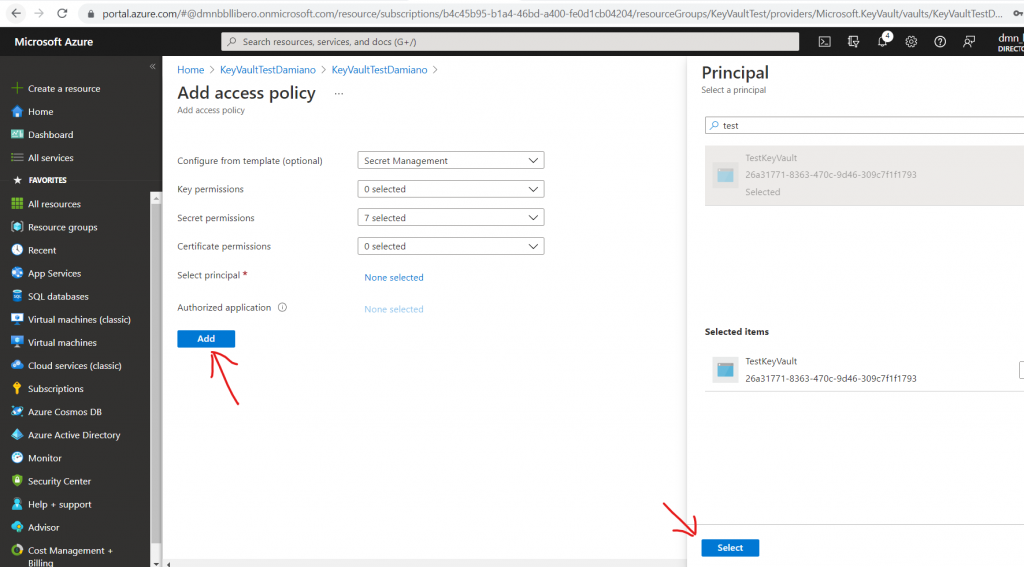
Finally, click on Save:
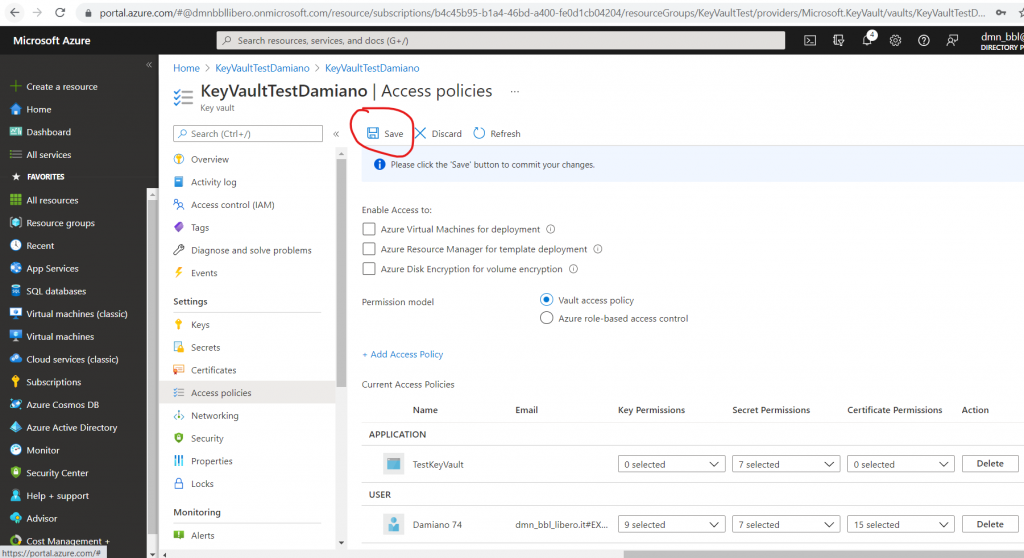
We have done and now, we can create our Console Application where we will add this code in the file Program.cs
[PROGRAM.CS]
using Azure.Identity;
using Azure.Security.KeyVault.Secrets;
using System;
using System.Threading.Tasks;
namespace AppKeyVault
{
class Program
{
static async Task Main(string[] args)
{
string connValue = await ReadSecret();
Console.WriteLine($"The Connection string is: {connValue}");
Console.WriteLine();
}
static async Task<string> ReadSecret()
{
// Definition of all parameters used to manage the Azure Key Vault
string clientId = "26a31771-8363-470c-9d46-309c7f1f1793";
string clientSecret = "9-v4Gu3XGY-_-7QYGZvf9-Y07hR3tfuk-f";
string directoryId = "038ce72a-62b7-43fc-8094-72a5683095c8";
string secretName = "ConnectionString";
string urlKeyVault = "https://keyvaulttestdamiano.vault.azure.net/";
// Definition of the client used to manage the Key Vault
var client = new SecretClient(new Uri(urlKeyVault),
new ClientSecretCredential(tenantId: directoryId,
clientId: clientId, clientSecret: clientSecret));
// Async method used to get the value of a secret stored in Azure Key Vault
var secret = await client.GetSecretAsync(secretName);
return secret.Value.Value;
}
}
}
If we run the application, this will be the result:
